React Select in Practice: Real Examples, Customization, and Common Pitfalls
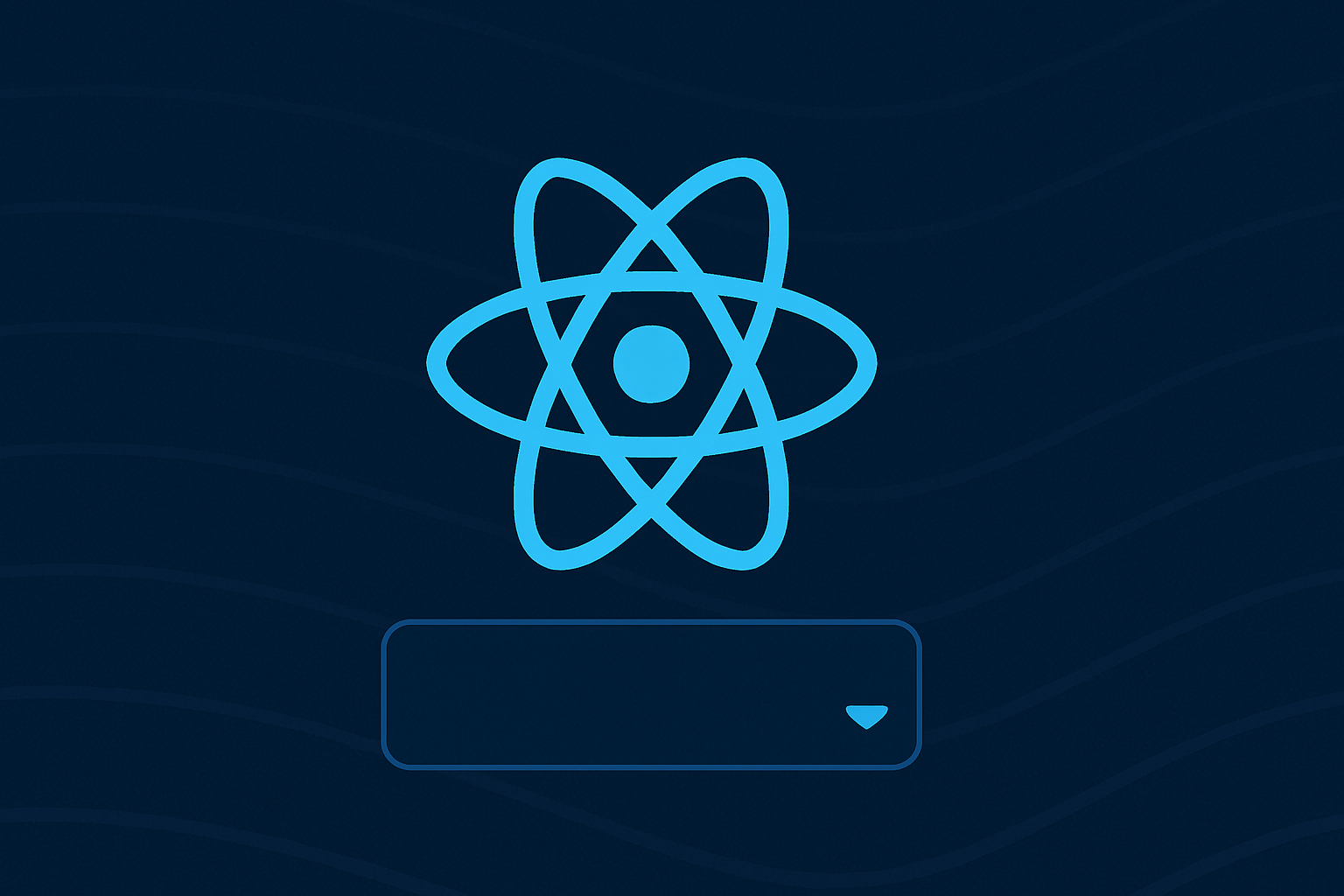
React Select is a powerful and flexible select input component widely favored in React applications for its customizability and ease of use. Basic implementation is straightforward, but real-world usage quickly reveals challenges. This guide covers practical insights and common pitfalls to ensure smooth usage.
Key Takeaways
- React Select is highly customizable using built-in props and styling options.
- Real-world scenarios require handling async loading, large datasets, and integration with form libraries.
- Avoid common pitfalls such as excessive re-renders, styling conflicts, and accessibility issues.
Quick Installation
Install React Select using npm or yarn:
npm install react-select
# or
yarn add react-select
Basic Usage: Minimal Viable Example
Here’s the simplest example to quickly understand React Select:
import Select from 'react-select';
const options = [
{ value: 'apple', label: 'Apple' },
{ value: 'banana', label: 'Banana' },
{ value: 'cherry', label: 'Cherry' },
];
const MyComponent = () => (
<Select options={options} />
);
Practical Customization
Styling React Select
Customize styles using the styles
prop:
<Select
options={options}
styles={{
control: (baseStyles, state) => ({
...baseStyles,
borderColor: state.isFocused ? 'blue' : 'gray',
boxShadow: 'none',
'&:hover': { borderColor: 'blue' },
}),
option: (baseStyles, state) => ({
...baseStyles,
backgroundColor: state.isSelected ? 'blue' : 'white',
color: state.isSelected ? 'white' : 'black',
'&:hover': { backgroundColor: 'lightgray' },
}),
}}
/>
Handling Async Options
Use AsyncSelect
for API-loaded options:
import AsyncSelect from 'react-select/async';
const loadOptions = (inputValue) =>
fetch(`https://api.example.com/fruits?search=${inputValue}`)
.then(res => res.json())
.then(data => data.map(item => ({ label: item.name, value: item.id })));
<AsyncSelect cacheOptions loadOptions={loadOptions} />;
Real-World Use Cases
Integration with React Hook Form
Use React Select effectively with forms:
import { Controller, useForm } from 'react-hook-form';
const MyForm = () => {
const { control, handleSubmit } = useForm();
return (
<form onSubmit={handleSubmit(data => console.log(data))}>
<Controller
name="fruit"
control={control}
render={({ field }) => (
<Select {...field} options={options} />
)}
/>
<button type="submit">Submit</button>
</form>
);
};
Multiselect with Custom Tagging
<Select
options={options}
isMulti
closeMenuOnSelect={false}
hideSelectedOptions={false}
/>
Accessibility Best Practices
Ensure select components are accessible:
- Support keyboard navigation (up/down arrow, enter, escape).
- Clearly label components for screen readers:
<label htmlFor="fruit-select">Choose a Fruit</label>
<Select inputId="fruit-select" options={options} />
Troubleshooting and Common Mistakes
Excessive Re-renders
Use memoization to avoid unnecessary re-renders:
const options = useMemo(() => [
{ value: 'apple', label: 'Apple' },
], []);
Styling Conflicts
Use CSS-in-JS or scoped styles to manage styling conflicts effectively.
Form State Integration Issues
Use React Hook Form’s Controller
to handle state properly.
Quick Performance Tips
- Virtualize large datasets using libraries like
react-windowed-select
. - Debounce async search inputs to optimize performance.
When Not to Use React Select
React Select isn’t ideal for:
- Simple dropdowns (native select or simpler libraries recommended).
- Extremely large datasets without virtualization.
Conclusion
Using React Select effectively requires understanding customization, accessibility, and performance considerations. By addressing practical scenarios and pitfalls, you can ensure your React Select implementations are robust and user-friendly.
FAQs
[TOGGLE question=“How do you clear React Select programmatically?” answer=“You can clear the select by using a ref: <Select ref={ref => ref.clearValue()} />
.” ]
[TOGGLE question=“How do you pre-select options in React Select?” answer=“Pass the selected option(s) as the value
prop to React Select.” ]
Use the `styles` prop or CSS-in-JS libraries like Emotion or Styled Components for detailed customization.