A Definitive Guide to Reactivity in Solid.js
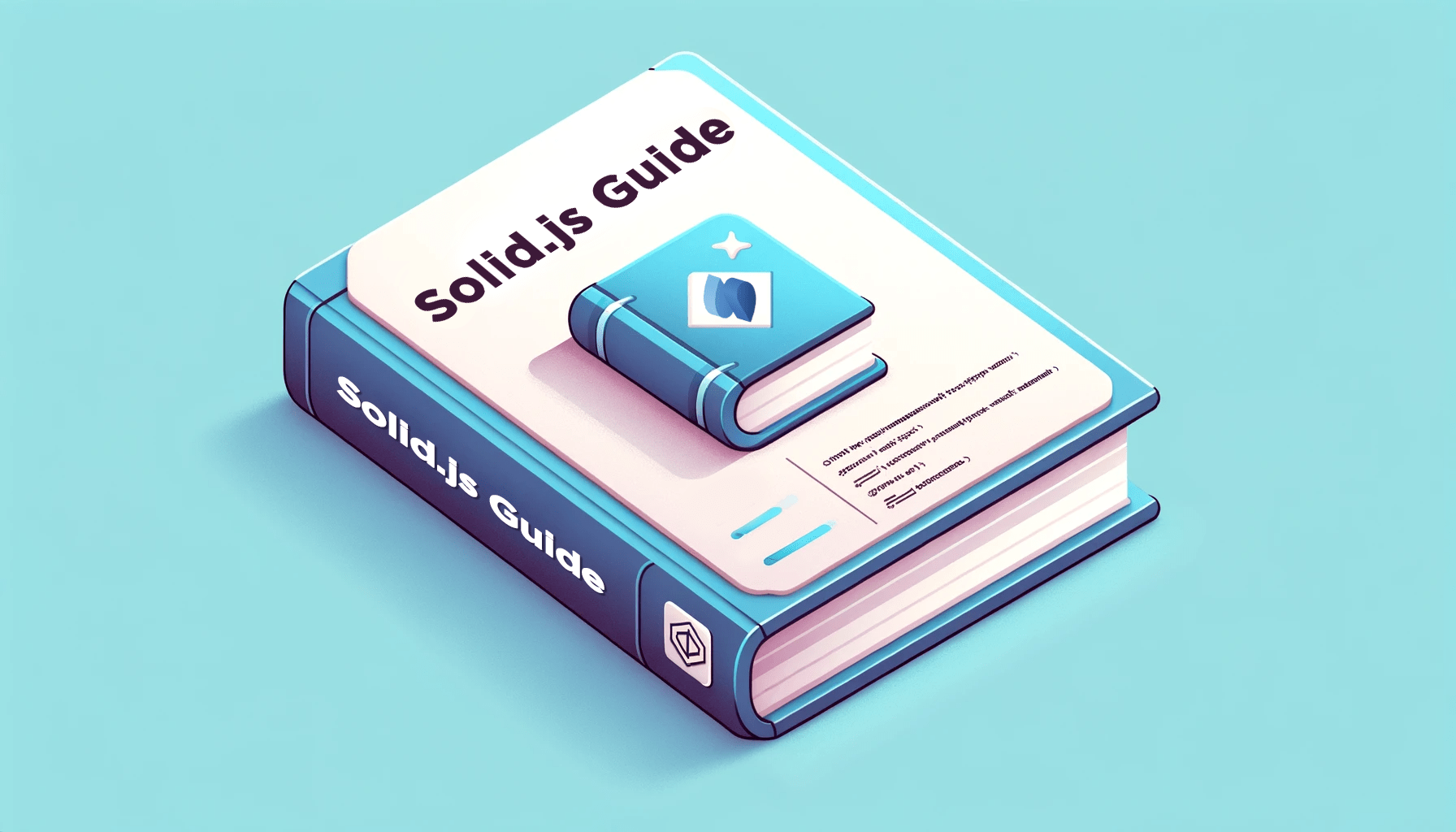
xxxxxxxxxxxxxxxxxxxxxxxxxxxxx xx ABSTRACT TEXT GOES HERE xx xxxxxxxxxxxxxxxxxxxxxxxxxxxxx
Discover how at OpenReplay.com.
Reactivity is a core concept for building lightning-fast, performant, responsive web applications. Solid.js, with its efficient reactivity system, minimizes unnecessary re-renders and remains important for optimizing user interfaces. This article covers essential reactivity concepts in Solid.js, including creating reactive states using
createSignal
, tracking dependencies, describing how to usecreateSignal
andcreateEffect
to define states and effects, and sharing some advantages of Solid.js’s reactivity system over other traditional approaches.
Introduction
Solid.js is a modern JavaScript framework designed for efficient and high-performance web development. It prioritizes reactivity, automatically updates components when data changes, and maintains a responsive user interface.
With its minimal re-rendering and fine-grained dependency tracking, Solid.js optimizes web application performance. Solid.js has a simple, declarative syntax and a small bundle size, making it an excellent choice for building lightning-fast web applications.
In this article, we’ll cover the essential concepts of reactivity in Solid.js so you can build performant web applications with Solid.js.
To better understand this topic, you should have a good grasp of HTML, CSS, and the fundamentals of JavaScript. In addition, you should also have basic knowledge of Solid.js before you continue with this topic.
Now, let’s get started.
Understanding Reactivity in Web Development
In web development, reactivity is the ability of web applications to automatically and efficiently update the interface in response to changes in data or user interactions.
Solid.js, however, has a “high-level” reactive system that does not make use of the virtual DOM (unlike other declarative web frameworks like React, Vue.js, and Elm) but instead makes use of a compiler (that still gives optimal native DOM updates).
Solid.js uses a fine-grained reactivity system, which tracks dependencies at a granular level. This reactive system ensures that only the specific components or parts of the DOM that change are re-rendered, minimizing unnecessary updates and improving performance.
Let’s say you have a complex application with multiple components and shared data. With Solid.js, the reactivity system (unlike other frameworks) automatically updates only the affected parts of the user interface (UI) when data changes. If you’re familiar with React, you’ll recall that React re-renders the entire component, and most times, you may need to use additional libraries or patterns like Redux or Context API to manage the shared states and reduce re-renders.
Importance of Reactivity for Responsive User Interfaces
Reactivity is important for creating user interfaces that feel fluid, interactive, and capable of providing real-time feedback. There are some obvious reasons to consider reactivity the next time you build a web application.
Consider the following reasons:
-
Enhanced User Experience: Reactivity creates a more enjoyable and intuitive user experience. When a user clicks a button, enters text, or performs any action, the interface should update in real time to provide feedback. This immediate response enhances the user experience and makes the application feel more interactive and responsive.
-
Performance optimization: A great framework like Solid.js is designed to be efficient. The built-in reactivity system updates only the parts of the interface that have changed rather than re-rendering the entire component (or page). This minimizes the user’s device and network load, resulting in faster response times and a smoother user experience.
-
Complex state management: Many modern applications have complex state management requirements. Reactivity helps manage this complexity by automatically tracking and updating the state of the application as it changes. This reduces the need for manual state management and helps prevent bugs and inconsistencies in the interface.
The Core Principles of Reactivity in Solid.js
Solid.js prioritizes performance via its fine-grained reactivity system. Reactivity in Solid.js is built upon several core principles. Two of the core concepts are dependency tracking and reactive primitives. These concepts play an important role in how Solid.js updates components efficiently.
Let’s briefly look at each of them in more detail:
1. Dependency Tracking
Dependency tracking is how the Solid.js system takes notes of the relationships between data and components to ensure efficient updates. This mechanism helps Solid.js understand which pieces of data (often called “signals”) are used by the components.
Dependency tracking is important in web or software development projects for several reasons:
-
Real-Time Updates: Dependency tracking allows web apps to automatically update components or data when dependent data changes. This is essential in user interfaces, where changes in data or state should be reflected immediately to ensure a smooth and responsive user experience.
-
Efficiency: Tracking dependencies helps optimize performance by reducing unnecessary updates. With Solid.js, only the affected components or data are updated when their dependencies change, rather than updating everything, which can be computationally expensive.
-
Maintainability: Dependency tracking can simplify code maintenance. Developers can focus on individual components or data without worrying about the intricate details of synchronizing everything. This makes codebases more modular and easier to maintain.
In Solid.js, reactive primitives are used to keep track of dependencies. We’ll get to that shortly.
The thing to note is that dependency tracking aims to update only the parts of the component that depend on the changing data due to any user interactions. This process minimizes the framework’s work and leads to a responsive and efficient user interface.
2. Reactive Primitives
Reactive primitives are key to creating dynamic and responsive user interfaces. They are the building blocks of reactivity in a framework like Solid.js.
Reactive primitives can be read and written to, and when their value changes, they trigger updates in components that depend on them.
Solid.js depends on reactive primitives to track dependencies. When a component accesses the value of reactive primitives, the framework records this as a dependency.
As you’ve learned, Solid.js uses a fine-grained reactivity system to provide efficient dependency tracking and other updates. So, rather than re-rendering an entire component (although it sometimes does that), it updates only what’s necessary.
Now, let’s consider each of them in more detail:
A). createSignal:
The createSignal
defines a piece of reactive state and a function to modify that state. The createSignal
usually has two parts function:
-
The getter function allows you to access the current state of the signal and,
-
the setter function allows you to set or update the current state of the signal.
When the state changes, any components or effects that depend on it will automatically re-render.
Look at an example of how you can use createSignal
to manage and display a counter in a Solid.js component:
import { createSignal } from "solid-js";
function Counter() {
const [count, setCount] = createSignal(0);
const increment = () => setCount(count() + 1);
return (
<div>
<p>Count: {count()}</p>
<button onClick={increment}>Increment</button>
</div>
);
}
In this example, createSignal
is used to create the count
state variable and the setCount
function to update it. Clicking the “Increment” button will automatically update the count and trigger a re-render of the component.
If you have used other JavaScript libraries like React or Vue.js, the
createSignal
is equivalent touseState
in React andref
in Vue.js.
B). createEffect:
The createEffect
is used to create side effects in your component that automatically react to changes in reactive data. It’s similar to the useEffect
hook in React and the watchEffect
in Vue.js.
Here’s an example of how you can use createEffect
to log the current count whenever it changes:
import { createSignal, createEffect } from "solid-js";
function CounterWithEffect() {
const [count, setCount] = createSignal(0);
createEffect(() => {
console.log(`Count is now: ${count()}`);
});
const increment = () => setCount(count() + 1);
return (
<div>
<p>Count: {count()}</p>
<button onClick={increment}>Increment</button>
</div>
);
}
The createEffect
hook will automatically run in the above example whenever the count
state variable changes. It will log the current count to the console.
Reactivity in Solid.js vs. Traditional Approaches
The reactivity model in Solid.js differs significantly from traditional approaches to state management in other frameworks, like React, Angular, or Vue.
Here are the advantages of Solid.js’s reactivity model over traditional approaches:
Fine-Grained Reactivity
Solid.js leverages a fine-grained reactivity model that tracks dependencies at the level of individual data structures and values. When a piece of state changes, only the components that depend on that specific piece of state are re-rendered.
In traditional approaches, such as React, changes to the state can trigger re-renders of entire components or sub-trees, even if only a small portion of the component depends on the changed data.
Additionally, Solid.js distinguishes itself from other frameworks like Angular and Vue.js by not relying on the virtual DOM and opting for a more direct approach through its high-level reactive system and compiler-based optimization for native DOM updates. This nuanced reactivity model enhances performance by minimizing unnecessary updates, offering a unique alternative as a web development framework.
No Virtual DOM
Solid.js does not update changes with a virtual DOM. Instead, it directly updates the real DOM, which sometimes leads to better performance.
Traditional frameworks like React use a virtual DOM to minimize the number of real DOM updates, which can be less efficient in some scenarios. Solid.js stands apart from other declarative frameworks and Angular, which relies on a virtual DOM for its reactivity mechanism.
Reactive Static Analysis
Solid.js’s fine-grained reactivity system leverages a static analysis approach to track dependencies at build time. This approach allows it to optimize and reduce the size of the JavaScript bundle and improve the application’s loading time and runtime performance.
Compared to traditional frameworks like React, Vue.js, and Angular, which primarily determine dependencies at runtime, Solid.js’ static analysis approach provides several advantages. Solid.js can generate a more optimized and compact JavaScript bundle by resolving dependencies during the build process. This contributes to a more efficient runtime performance and results in improved loading times for the application. Frequently, traditional frameworks may face challenges related to less efficient updates and potentially larger bundle sizes due to their runtime dependency determination.
Conclusion
Throughout this guide, we’ve covered the essentials of reactivity in Solid.js for building super-performant web applications. We’ve learned how Solid.js leverages fine-grained reactive systems to track dependencies and uses reactive primitives like createSignal
and createEffect
to manage and react to state and data changes. We’ve also learned some advantages of Solid.js’s reactivity system over other traditional approaches. I hope you found this article helpful. To learn more about Solid.js, read their official documentation.
Gain Debugging Superpowers
Unleash the power of session replay to reproduce bugs, track slowdowns and uncover frustrations in your app. Get complete visibility into your frontend with OpenReplay — the most advanced open-source session replay tool for developers. Check our GitHub repo and join the thousands of developers in our community.