Demystifying Security in Front-end Development: A Comprehensive Overview
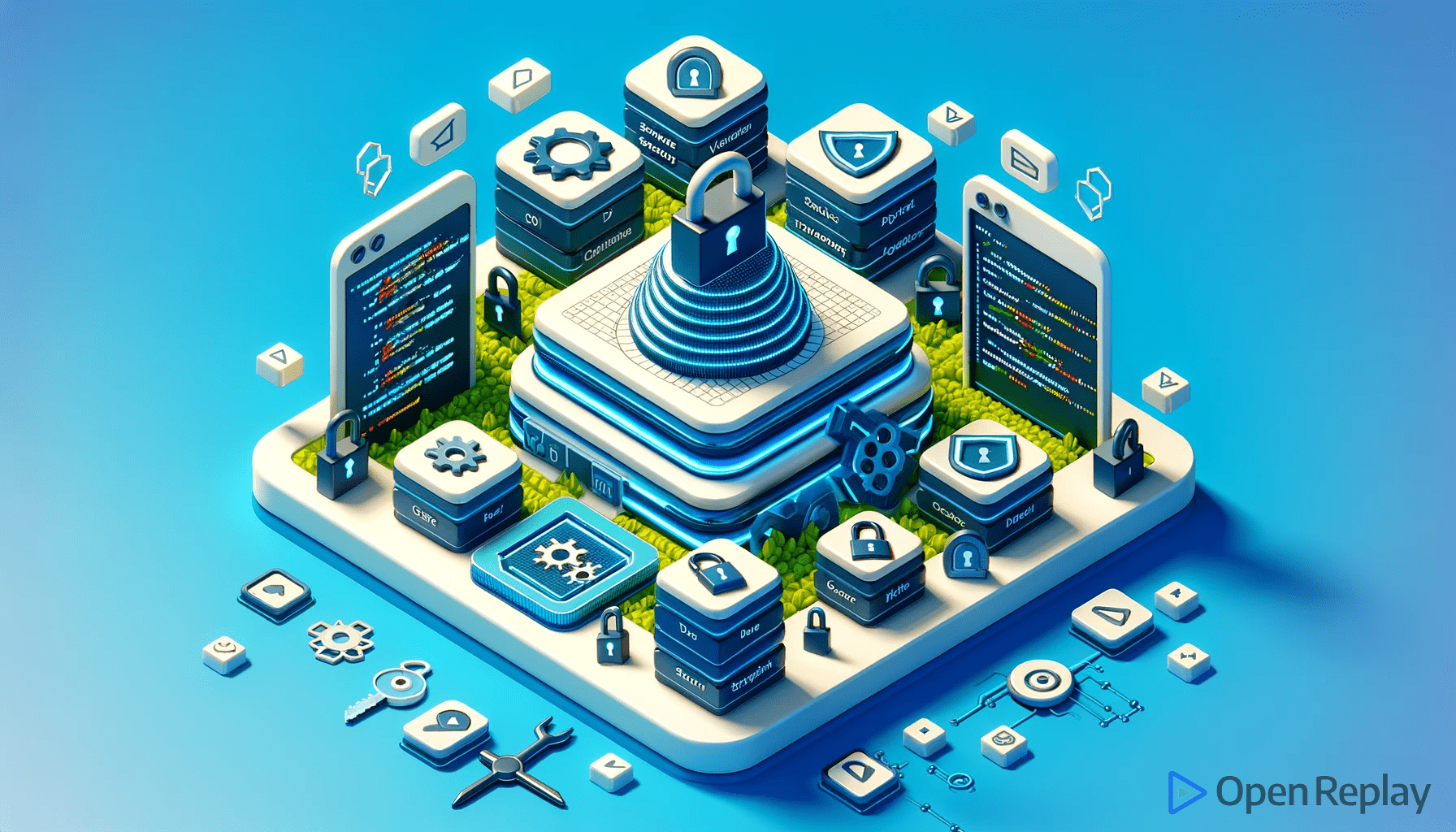
Front-end security refers to the set of practices and measures implemented to secure the user interface and experience of a web application, as well as the client-side components of any software application, and protect users from malicious attacks that can compromise their data and privacy. This article shows the use of encryption, secure coding practices, and secure communication channels to create a more secure environment for users.
Spot abnormal user behaviors and iron out the bugs early with OpenReplay. Dive into session replays and reinforce your front-end against vulnerabilities that hackers search for.
Discover how at OpenReplay.com.
Front-end security is crucial to protecting users, an organization’s reputation, and its compliance status. Some common security threats in front-end app development are:
- Cross-site scripting (XSS) attacks exploit vulnerabilities in web applications to inject malicious code into the client-side script.
- Injection attacks attempt to inject malicious code into the input fields of an application.
- Broken access control occurs when an application fails to properly restrict access to certain resources or functionality.
- Insecure direct object references occur when an application uses user-supplied input to access an object without proper validation.
Protecting Sensitive Data
Sensitive data protection involves using a variety of security measures to keep private and confidential information secure from unauthorized access, theft, or disclosure. This includes protecting personal data, financial records, intellectual property, and other sensitive information. Some security measures used to protect sensitive data include:
Client-side Data Encryption
Client-side encryption protects sensitive data by encrypting it before it leaves the client device, such as a web browser or mobile app. Even if an attacker intercepts the data in transit or obtains access to the server, the information is unintelligible without the decryption key. This makes client-side encryption a valuable tool for protecting sensitive data, as it helps to ensure that the data remains secure even if the server is compromised.
When a user enters or creates sensitive data, it is immediately encrypted on the client device using an encryption algorithm and a secret key. This process transforms the data into a scrambled format that cannot be understood or used without the corresponding decryption key. The encryption key is vital for decrypting the data and must be managed securely on the client side; without this key, the data remains unreadable and essentially useless.
Once the data is encrypted on the client side, it is sent to the server or database. Even if an attacker were to intercept the data during transmission, it would still be unreadable because it is in an encrypted format. Even if an attacker could access the database, they would only see the encrypted data, which would be useless without the encryption key. This helps to ensure that the data remains protected even if the database is compromised. When a user needs to access or modify the data, they provide the decryption key, which is used to decrypt the data on the client side. This ensures that the sensitive information is only exposed in its readable format when needed and remains encrypted at all other times. This provides an additional layer of protection for the data, as it is only in its readable format for a short period of time.
Securely Managing API Keys and Secrets
In web applications and services, securely managing API keys and secrets is essential for protecting sensitive data. API keys and secrets are used for authentication and authorization, and if they are compromised, it can lead to unauthorized access, data breaches, or abuse of services.
The best practice for managing API keys and secrets is storing them outside your source code. Environment variables are the most common way to do this, as they allow you to keep secrets separate from your application code. This prevents the accidental exposure of secrets if your code is accidentally leaked or shared through version control. Using environment variables makes it easy to change or update your secrets without having to make changes to your code.
In addition to using environment variables, consider using secrets management tools and services. These can provide an even more secure and centralized way to store and manage secrets. Popular options include HashiCorp Vault, AWS Secrets Manager, and other similar solutions. These tools often come with advanced features such as encryption, versioning, and access control, which can further enhance the security of your secrets.
In using secrets management tools, it is also important to regularly rotate your API keys and secrets. This limits the time a compromised key could be used, reducing the risk of service disruption or data breach.
Implementing logging and auditing mechanisms is another important measure for protecting API keys and secrets. This allows you to monitor who accesses or modifies your secrets so you can quickly detect suspicious activity or unauthorized access.
Avoiding Data Exposure in the Front-end
When it comes to protecting sensitive data, avoiding data exposure in the front end is a crucial step. This means taking measures to ensure that no sensitive data is exposed to users or potential attackers through the user interface. This includes avoiding the use of sensitive data in any code that is executed in the browser and ensuring that all user input is properly sanitized and validated to prevent malicious input from being sent to the server.
When designing a user interface, collecting and displaying only the minimum amount of data necessary for the current task or functionality is important. This helps to avoid exposing sensitive or unnecessary information that users do not need to see.
Client-side validation is an important tool for preventing the submission of data that does not meet the required criteria. By validating the data on the client side before it is sent to the server, developers can prevent users from accidentally or intentionally submitting data that could be harmful or expose sensitive information.
It is crucial to always use HTTPS when transmitting data, as it encrypts the data in transit. This prevents malicious actors’ eavesdropping and interception of data, ensuring that sensitive information remains secure.
Configuring Cross-Origin Resource Sharing (CORS) settings is an important step for protecting sensitive data from unauthorized access. By configuring CORS, developers can control which websites can request their API, preventing malicious websites from accessing sensitive data.
By following these best practices, among others, for protecting sensitive data in the front end, you can significantly reduce the risk of data exposure and unauthorized access. With proper configuration of CORS, HTTPS, and client-side validation, you can create a more secure environment that is less vulnerable to common attacks.
Secure coding practices
Secure coding practices are a set of rules and best practices that are designed to help developers create software that is less vulnerable to attacks. The goal is to reduce the number of potential vulnerabilities in a program, making it more difficult for attackers to exploit them.
Avoiding Common Security Pitfalls in JavaScript
Secure coding practices for JavaScript are essential to mitigate the security risks that the language can introduce. JavaScript is a client-side scripting language, meaning that it runs on the user’s browser. As a result, there is a higher risk of potential vulnerabilities and attacks, such as Cross-site scripting (XSS), Cross-site request forgery (CSRF), and other client-side attacks.
To avoid pitfalls in JavaScript, always validate and sanitize user input, checking that the input data is in the expected format and that it doesn’t contain any malicious code or characters that could be used to exploit a vulnerability before using it on the client side.
It is important to avoid using inline JavaScript within HTML attributes, such as onclick
or href
, as this can leave your application vulnerable to script injection attacks. Instead, you should use event listeners and binding functions to handle interactions with your application. These techniques will separate the JavaScript code from the HTML, making it more secure.
You should avoid using the eval()
function in your code, as it can execute arbitrary code and is susceptible to injection attacks. There are many safer alternatives to eval()
that you can use instead, such as the Function()
constructor or the JSON.parse()
function. These alternatives are more secure and should be used whenever possible to avoid potential vulnerabilities.
Client-side storage mechanisms, such as localStorage
and sessionStorage
, should be used cautiously when storing sensitive data. While these mechanisms can be convenient and useful, they are not as secure as server-side storage and can be more easily exploited by attackers.
Using security headers like X-Content-Type-Options
, X-Frame-Options
, and X-XSS-Protection
can significantly improve the security of your application. These headers can prevent common attacks like cross-site scripting and cross-site request forgery and help to ensure that your application is served securely.
Using Libraries and Frameworks with Good Security Practices
To improve the security of your application, it’s important to choose third-party libraries and frameworks that have been developed and maintained with security in mind. When selecting third-party libraries, it’s important to look for those that adhere to industry standards and best practices, such as OWASP Top 10 or SANS Top 25, also look for projects that have a strong community of contributors and a demonstrated commitment to releasing security updates. Familiarizing yourself and your development team with the security documentation for any third-party libraries you use is crucial. This documentation can help you understand potential security issues and how to address them. Regularly scanning your application’s dependencies for known vulnerabilities can help to identify potential security risks.
Threat modeling and security reviews are critical in identifying and mitigating risks. By modeling potential threats to your application, you can determine the most vulnerable areas and prioritize mitigations. Additionally, regularly reviewing the security of your third-party libraries can help you stay up to date on new vulnerabilities and address them promptly. Regular security audits or assessments, such as penetration testing, can further ensure your application is secure.
Regularly Updating Dependencies to Fix Security Vulnerabilities
Updating your dependencies to fix security vulnerabilities is a fundamental best practice for secure coding. Keeping your dependencies up to date can help protect your software applications from potential vulnerabilities. It helps you keep pace with emerging threats and best practices in security. It also ensures that the entire stack remains secure. It helps to patch known security vulnerabilities.
Maintaining a comprehensive list of all the dependencies used in your project, including the version numbers, is important to effectively update dependencies regularly for security. Use tools and services that provide notifications when new versions are released. These tools scan your codebase for dependencies and compare the versions you are using with the latest releases.
When updating dependencies, testing the new versions in a development or staging environment before deploying them to production is important. Use package managers and automation tools (such as npm, Maven, or Composer) to help streamline the process of updating dependencies.
It is also important to stay informed about security announcements and news from the maintainers of your dependencies. Staying up-to-date on these announcements can help you prioritize updates and mitigate risks quickly.
Security Testing for Front-end Applications
Front-end security testing is a type of security testing that focuses on the front-end components of a web application, such as the user interface, scripts, and client-side code. It aims to identify security vulnerabilities and weaknesses in the front end that attackers could exploit to gain unauthorized access or manipulate the application. Front-end security testing aims to ensure that the front-end is secure and protected against common threats.
The front end includes JavaScript, HTML, CSS, and localstorage
mechanisms such as cookies and web storage.
Manual Code Reviews and Static Code Analysis
Software development has two main approaches to code review: manual code review and static code analysis. Both methods have their advantages and disadvantages, and choosing the right approach depends on the specific project and development goals.
-
Manual Code Reviews:- Manual code review is a process in which a developer or a team of developers manually inspects the source code of a program. The reviewers identify issues, such as bugs, security vulnerabilities, and violations of coding standards, and then provide feedback. This process is typically done during or after the development phase, and it can help to improve the quality of the code and catch issues that might otherwise have been missed. It leverages the expertise and experience of human reviewers. It can also check for errors and issues that automated tools may not be able to detect, such as violations of coding standards and guidelines.
- Advantages: One of the biggest benefits of manual code review is that human reviewers can identify and catch errors that automated tools might miss. For example, reviewers can detect complex logic errors, such as off-by-one errors and race conditions, and identify code design flaws. In addition, the manual code review process can provide valuable insights into the overall quality and maintainability of the code, which can help inform future development decisions.
- Disadvantages: The process can be time-consuming and labor-intensive for large codebases. In addition, the quality of the review depends on the experience and expertise of the reviewers, and a lack of experience can lead to missed errors and vulnerabilities. The process can be subjective and inconsistent, and different reviewers may conclude differently about the same code.
-
Static Code Analysis:- Static code analysis is automated testing that analyzes the source code without actually running it. These automated tools scan the code for potential issues, such as syntax errors, coding standards violations, security vulnerabilities, and performance bottlenecks. Static code analysis tools work by using predefined rules and patterns, known as rulesets, to identify potential issues in the code. The rulesets are usually customizable, so you can configure the tool to look for specific issues based on your organization’s coding standards and security requirements. Static analysis tools can be run quickly and automatically, which makes them a valuable part of the development pipeline. They can be configured to run automatically on every commit or pull request, providing rapid feedback to the developers.
- Advantages: One of the key benefits of static code analysis is its efficiency in identifying common coding mistakes, adherence to coding standards, and potential security vulnerabilities. The process can be automated and integrated into the development pipeline, making it suitable for quickly scanning large codebases. The static code analysis results can help ensure consistency across the codebase, detect coding standards violations, and enforce best practices.
- Disadvantages: There is the potential for false positives, where the tool reports an issue that does not exist, and false negatives, where the tool fails to identify a genuine issue. This means it’s important to verify the issues reported by the tool with a manual code review. In addition, automated static analysis may not be as effective at identifying complex logical errors or design flaws as a human reviewer.
Automated Security Scanning Tools
Security scanning tools are automated applications designed to identify and analyze security vulnerabilities, weaknesses, and other security issues in computer systems, applications, and networks. These tools are critical for security teams as they provide a systematic and comprehensive way to discover and address vulnerabilities that might otherwise go undetected.
Some key types of Automated security scanning tools are:
- Static Application Security Testing (SAST):- SAST tools are designed to analyze the source code of an application, bytecode, or binary code. They identify potential vulnerabilities, coding errors, and adherence to coding standards.
- Dynamic Application Security Testing (DAST):- DAST tools are used to assess an application from the outside, simulating attacks that malicious actors could perform. They send various requests to the application and analyze its responses to identify vulnerabilities that might be exploited during runtime, such as cross-site scripting, SQL injection, authentication issues, and misconfigurations.
- Interactive Application Security Testing (IAST):- IAST tools combine the best of both worlds, SAST and DAST. They analyze the runtime behavior of an application and its source code, identifying vulnerabilities and providing real-time feedback to developers. IAST tools can provide greater insight into the application’s security posture than either SAST or DAST alone, making them a valuable addition to any security testing strategy.
- Software Composition Analysis (SCA):- SCA tools are designed to identify and mitigate security vulnerabilities and licensing issues in third-party and open-source libraries and components used in an application. SCA can help ensure that applications are built using secure and compliant components, minimizing the risk of introducing vulnerabilities into the code.
- Network Scanners:- Network scanning tools are used to identify security vulnerabilities in network infrastructure and devices by scanning IP addresses, ports, and network protocols. By probing the network for open ports, listening services, and weak configurations, network scanning tools can find weaknesses that attackers could exploit. Network scanners can also test for vulnerabilities in firewalls, servers, and routers, helping to secure the network and prevent data breaches.
User Testing for Security Flaws
User testing for security flaws, also known as security usability testing or security user experience testing, is an important part of developing secure software. This process involves observing how users interact with the system and identifying any security-related issues that might arise in real-world use. It’s crucial to understand how users are likely to use the system and what security risks they might face to design and implement effective security measures.
Common Security Flaws to Look for in User Testing
- Password Weaknesses:- Weak password practices are a common cause of security breaches, so checking for them during security testing is crucial. Some examples of weak password practices include using easily guessable passwords, reusing passwords across different accounts, or not setting up two-factor authentication (2FA).
- Phishing Susceptibility:- Phishing is a common type of attack that can lead to security breaches, so it’s important to test how easily users fall for these types of attacks. Some common phishing methods include emails, links, and social engineering. Email phishing involves sending emails that appear to be from a legitimate source, while link phishing involves providing links that lead to malicious websites. Social engineering involves manipulating users into divulging sensitive information or taking actions that compromise security.
- Confusing Security Settings:- Security testing should also assess how easy it is for users to configure privacy settings, enable secure communication, and use encryption. It’s important to make sure that users can easily find and understand the settings they need to configure and that they can choose secure communication options without difficulty.
- Account Recovery Issues: Another key aspect of security testing is evaluating the account recovery process, including the ease of use, effectiveness, and security of the process. A well-designed account recovery process should be easy to use while still protecting user data and preventing unauthorized access.
- Data Handling:- This includes looking at how users share data and how they protect it from unauthorized access. For example, testing can assess whether users are aware of the risks associated with sharing sensitive information and whether they are taking steps to protect their data, such as using encryption or strong passwords.
- Security Alerts:- Observing how users respond to security alerts and warnings is crucial. For example, many web browsers provide alerts when a user is visiting an insecure website, but users don’t always take action in response to these alerts. Similarly, antivirus software may alert users to potential malware, but users may not understand the warning or follow the recommended steps to remove the threat.
When testing users for security flaws, it is important not only to identify and fix any issues found but also to incorporate user feedback and findings into the overall design and development process. This feedback can help improve the security usability of the system by ensuring that security measures are easy to understand and use without negatively impacting the overall user experience. The goal is to balance security and usability so that users feel confident and secure when using the system.
Handling Errors and Logging
Handling errors and logging are key software development and maintenance practices. They are essential for ensuring an application’s reliability, security, and performance, and they involve capturing, managing, and analyzing errors, exceptions, and events during the application’s execution. These practices can help developers identify and fix problems, optimize performance, and ensure compliance with industry standards and regulations.
Avoiding Detailed Error Messages in Production
It is generally best practice to avoid providing detailed error messages. While such messages can be helpful for developers and administrators when troubleshooting, they can also provide valuable information for attackers. Therefore, it’s important to balance providing enough information to diagnose and fix issues while minimizing the risk of exposing sensitive information. Errors that expose sensitive data can seriously risk users’ privacy and security. This is because error messages can inadvertently reveal valuable information that malicious actors could exploit. For example, if an error message includes a user’s account ID, an attacker might be able to use that information to launch a targeted attack. Similarly, exposing the database schema or table structure could allow an attacker to craft a targeted attack that exploits specific weaknesses in the database.
In addition to being a security risk, detailed error messages can also be confusing and misleading for users. A user who sees a detailed error message with complex technical information may not understand what it means or how to respond appropriately. This can lead to further confusion and potentially make the situation worse. For example, the user might attempt to fix the problem themselves and inadvertently make it worse, or they might report the error to the wrong person or department.
A best practice for handling errors is to design custom error pages that provide an informative, user-friendly message without revealing technical details. This allows users to know that an error occurred without confusing them with details that they might not understand or find useful. For example, the error page could simply state that an error has occurred and provide a contact email address for support. This way, users can report the error without worrying about understanding the specifics. Although it’s important to keep error messages user-friendly and avoid displaying technical details, it’s still crucial to capture detailed logs for debugging purposes. When errors occur, it’s important to be able to investigate and fix them as quickly as possible.
However, these log files should be stored securely, away from any publicly accessible areas, and only authorized users should be able to access them. Error codes should be used, generic error messages should be provided, and continuous monitoring of applications for leakage of any sensitive information should be done, too. Additionally, it’s important to thoroughly test error pages to ensure they function correctly in all circumstances.
Proper Error Handling and Reporting
Proper error handling and reporting are essential for developing reliable and secure software. By effectively identifying, tracking, and addressing errors, developers can create a better user experience and minimize the risk of security vulnerabilities. A well-designed error handling and reporting process can help improve the software’s quality, reduce the risk of data loss or security breaches, and ultimately make the application more efficient and reliable. Ultimately, error handling and reporting should be integral to any software development process.
It’s important to keep error messages clear, concise, and informative when designing them. An error message aims to provide the necessary information to resolve the error in a way that is easy to understand for both developers and end-users. Error messages should be written in plain language, with simple and straightforward wording. They should also be designed to be visually clear and easy to read. In implementing error handling and reporting in a software application, it’s important to have a comprehensive logging mechanism in place. Logs should record detailed information about errors, warnings, and other important events, including timestamps, user context, and any relevant data. The logging mechanism should be able to handle a large volume of data and be capable of storing and searching logs efficiently.
Additionally, logs should be secure and only accessible to authorized users. In addition, Graceful degradation is an important factor to consider in designing error handling for a software application. This is the practice of designing the application to continue functioning even when errors occur, with a reduced set of features and functionality. By implementing graceful degradation, the application can continue to provide basic functionality and service even when errors occur, rather than crashing or locking up. This can help to maintain service availability and minimize disruption for end-users.
Content Security and Data Sanitization
Content security and data sanitization are two important aspects of ensuring the security of a software application. Content security refers to securing any content that is transmitted by the application, such as text, images, or documents. This includes measures such as encryption and access control. Data sanitization refers to ensuring that any user input is properly cleaned and validated before being used in the application. This can help prevent common attacks such as cross-site scripting and SQL injection.
Handling Untrusted Content
Handling untrusted content is an essential part of securing web applications. Untrusted content refers to any data that comes from an external source, such as user input, third-party applications, or remote systems. Since this content is not under the control of the application, it can introduce security vulnerabilities such as XSS and SQL injection. Therefore, it’s important to implement secure coding practices, input validation, and sanitization to ensure that untrusted content is handled securely.
Content Security Policy (CSP), as a practice for handling untrusted content, is a web security standard that allows developers to specify which sources of content are considered trusted. CSP defines a set of policies for scripts, styles, images, and other types of content and restricts the loading of content from sources that are not on the whitelist. This makes it possible to prevent common attacks, such as XSS and cross-site request forgery (CSRF), by limiting the execution of malicious code from untrusted sources. Also, the Cross-Origin Resource Sharing (CORS) standard allows you to specify which domains are allowed to access resources on your web application. By setting CORS headers, you can prevent external domains from accessing your resources unless they are explicitly allowed. This helps to prevent unauthorized access to sensitive data and mitigates the risk of CSRF attacks. The HTML sandbox
attribute allows you to isolate potentially untrusted content, such as iframes, and limit its access to the rest of the page. This makes it harder for untrusted content to perform actions such as accessing cookies, executing scripts, or modifying other elements on the page.
Input validation is an important part of securing a web application. By validating user input on the server side, you can ensure that it conforms to expected formats and constraints. This can help to prevent injection attacks, such as SQL injection and command injection, by ensuring that only valid data is passed to the server. Some examples of input validation include checking that email addresses are in the correct format, that numbers are within a valid range, and that dates are valid. HTML purification libraries and filters are essential tools for protecting web applications from malicious code. By purifying user-generated content before rendering it, these libraries and filters can remove or neutralize any potentially harmful code, such as script tags, inline styles, or cross-site scripting (XSS) attacks.
By implementing these tools, you can significantly reduce the risk of malicious code affecting your application and the users who interact with it.
Implementing Safe Data Sanitization Techniques
Data sanitization is a critical component of securing a web application. By implementing safe sanitization techniques, you can prevent a range of security vulnerabilities, including XSS and SQL injection. These techniques typically involve removing or encoding untrusted input to prevent malicious code from being executed. The specific sanitization methods used will vary depending on the type of input and the context in which it is being used. However, the goal is always to ensure that the sanitized data is safe to be used in the application.
By validating the input against expected formats and constraints, it’s possible to detect invalid or malicious data before it can be processed by the application. This can include validating email addresses, checking numbers are within a given range, and enforcing length restrictions. Escaping or encoding data before it is rendered in HTML ensures that any potentially malicious content is rendered as static text rather than being interpreted as HTML. This helps to prevent XSS attacks by preventing malicious scripts from being injected into the HTML of the page.
Regular security testing includes vulnerability assessments, which are designed to identify any potential security weaknesses in the application, and penetration testing, which involves simulating attacks on the application to find any exploitable vulnerabilities. By regularly performing these tests, it’s possible to identify and fix issues before they can be exploited by an attacker. This helps to ensure the security of the web application and the data it handles.
Protecting Against Script Injection and HTML Manipulation
Script injection and HTML manipulation are common vulnerabilities that can lead to serious security breaches if not properly addressed. This is because these vulnerabilities can allow an attacker to inject malicious scripts into the HTML of a web page, which can then be executed by the page’s users. This can lead to a number of potential attacks, such as XSS attacks, which can steal data, hijack user sessions, or even redirect users to malicious websites. By taking appropriate security measures, it’s possible to prevent these attacks and protect users and data. Some practices for the mitigation of risks associated with script injection and HTML manipulation include:
- Input validation: Implementation of strict input validation on client and server sides to prevent issues like malformed or malicious data from being sent to the server.
- Output escaping: The use of proper encoding functions for your specific programming language or framework. This ensures that any potentially harmful characters in user input are properly escaped or encoded.
- Properly configure cookies: It prevents attackers from stealing or manipulating cookies, which could lead to serious security issues.
- Cross-Site Request Forgery (CSRF) Protection: Cross-site request forgery (CSRF) is a type of attack that tricks a user into sending a request to a web application that they’re already authenticated to, without their knowledge or consent. This can be prevented by implementing a variety of protection mechanisms. One common mechanism is to include a unique token in each form submission, which can be verified by the server to ensure that the request is legitimate. Other mechanisms, such as requiring additional authentication steps for certain actions, can also be used to help prevent CSRF attacks.
Security Updates and Best Practices
It’s crucial to regularly apply security updates and follow best practices for maintaining the security of any software application or system. As new threats and vulnerabilities are discovered, updates and patches are released to address them. Failing to install these updates can leave the application or system vulnerable to attack. In addition to applying updates, following best practices such as using strong passwords, implementing access controls, and using secure coding techniques can help to further strengthen the security of the system.
Keeping Front-end Dependencies Up-to-Date
Keeping front-end dependencies like JavaScript libraries, CSS frameworks, and other external assets up-to-date is an important part of securing and maintaining web applications. Out-of-date front-end dependencies can introduce security vulnerabilities, performance issues, and compatibility problems. To address these issues, developers should regularly review their dependencies for updates and apply them promptly. This not only ensures that the application is secure but also that it functions properly and is optimized for performance.
-
Importance of Keeping Front-end dependencies up to date:
- Security: By regularly updating dependencies to the latest versions, developers can patch vulnerabilities and reduce the risk of attack.
- Performance: By taking the time to update dependencies, developers can improve the overall performance and usability of their applications.
- Compatibility:- it is important to ensure that the application works well across a wide range of browsers and devices, providing a consistent and reliable experience for users.
-
Best Practices for Keeping Front-end dependencies up to date:
- Regular Dependency Checks: Using dependency management tools like npm, developers can automate the process of identifying and updating outdated packages. This tool can also be used to lock dependencies to specific versions to ensure that the application always uses the intended versions of packages. By using this tool and regularly reviewing dependencies, developers can keep their projects secure, performant, and compatible.
- Version range specification: Specifying version ranges for each package in the project’s
package.json
file allows developers to define the range of versions that are acceptable for each package, which helps to avoid issues caused by accidentally upgrading to a new major version. - Automated Dependency Updates: Automated dependency management tools and services like Dependabot provide a convenient way to keep track of updates and ensure that dependencies are always up-to-date. By connecting these tools to your project’s source code repository, they can monitor for new releases and notify you when updates are available.
- Documentation and Changelogs:- it is important to review the release notes and changelogs to understand what has changed and how it might affect your project. These notes can provide valuable information about breaking changes, new features, and other important updates.
By following these best practices, you can ensure that your front-end dependencies are always up-to-date, secure, and compatible with the latest web standards. This will not only improve the security and stability of your application but also allow you to take advantage of new features and optimizations that can improve the overall user experience.
Conclusion
Front-end development handles the critical task of presenting sensitive data to users and must be robust and secure. It is important to understand the risks and potential vulnerabilities and to take a comprehensive approach to security, including protecting user data, ensuring the integrity of the application, and managing dependencies.
By following best practices, developers can build secure and resilient web applications that protect users and data while providing a seamless user experience. A proactive and up-to-date approach is key to maintaining a secure front end. With an understanding of the risks and threats, developers can implement effective security measures that safeguard their applications and the trust of their users. By taking a holistic view of front-end security, you can protect your systems and users from harm.
Secure Your Front-End: Detect, Fix, and Fortify
Spot abnormal user behaviors and iron out the bugs early with OpenReplay. Dive into session replays and reinforce your front-end against vulnerabilities that hackers search for.