Setting and Updating CSS Variables with JavaScript
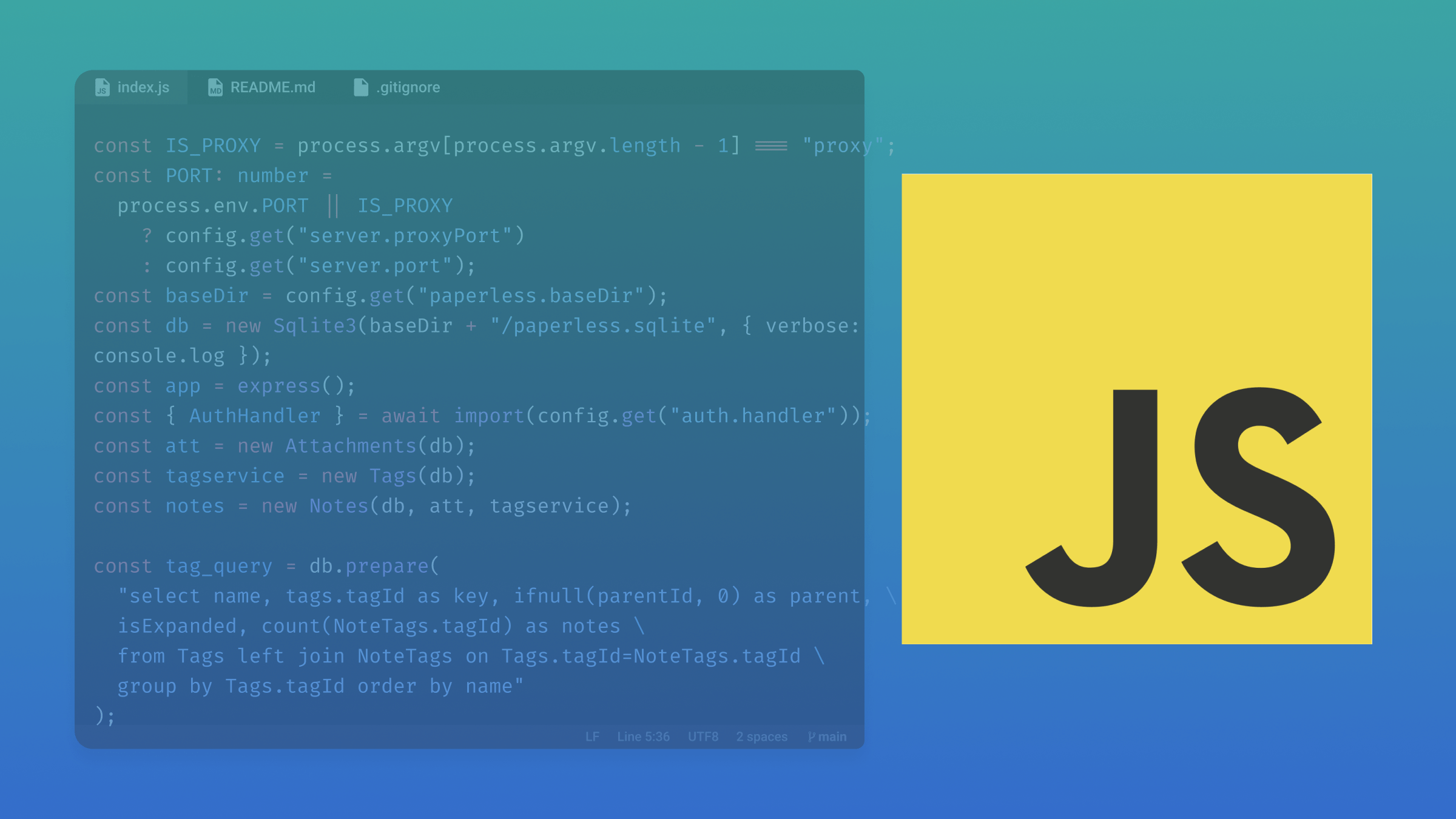
CSS variable is a tool that allows us to declare style values in one place and reuse them throughout our code (global scope) or within the selector where it is declared (local scope). The fact that CSS variables have access to the DOM allows us to change them using JavaScript. As a developer, you should master writing efficient CSS code. This article will teach you how setting up and updating CSS variables with JavaScript could help eliminate inefficiencies.
Discover how at OpenReplay.com.
As a developer, when dealing with CSS, you encounter some inefficiency. To better understand this, let’s look at this mockup design below.
Image screenshotted from Uplabs, edited, and labeled by the author.
Describing the mockup design above has the following features:
- The header and the place order button have the same background color.
- The Cart container background, header, and button text are the same color.
- The product name, quantities, and $23 are the same color.
- The plus sign, the minus sign, and the price are the same color.
Implementing the color of this mockup design in regular CSS should be something like this:
.cart-container {
background-color: #fff;
}
.header {
background-color: #393ef9;
color: #fff;
}
.product-name {
color: #5f6068;
}
.price {
color: #5f58bf;
}
.plus {
color: #5f58bf;
}
.minus {
color: #5f58bf;
}
.quantity {
color: #5f6068;
}
.button {
background-color: #393ef9;
color: #fff;
}
Observe the code above closely. We repeated colors multiple times because two or more selectors share the same color. Dealing with this kind of code, you will encounter some inefficiencies like:
-
Finding the CSS code hard to read and maintain: Trying to change the mockup design color from its default state to a different color will be difficult because following the design pattern(two or more elements should have the same color), you must change the color of all the elements manually, which is a very stressful method of maintaining CSS code and debugging will be difficult.
-
Maintaining a consistent look across your websites and applications requires much effort: Keeping a consistent look and feel across websites and applications requires much effort. It involves designing and implementing a unified visual style, including colors, typography, and layout, to create a cohesive user experience.
-
Writing static CSS code that cannot update variables based on user experience: The CSS code above is static. If a user wishes to change the style above to his choice, it is impossible because the user has no control over the styles.
Benefits of setting up and updating CSS variables with JavaScript
The following are some benefits of setting up CSS variables and updating them with JavaScript:
- Code reusability: Helps you to write readable and maintainable code.
- Consistency and Theming: CSS variables help to maintain a consistent look and feel across your websites or applications.
- JavaScript integration: The CSS variables live in the DOM, which implies we can set up and update CSS variables with JavaScript to give a user level of control over the styles of websites and applications. That is to say, a user can change colors, fonts, and more to his choice.
Let’s rewrite the above code with CSS variables and see how it eliminates inefficiencies.
:root {
--primaryColor: #393ef9;
--lightPrimaryColor: #5f58bf;
--secondaryColor: #5f6068;
--neutralColor: #fff;
}
.cart-container {
background-color: var(--neutralColor);
}
.header {
background-color: var(--primaryColor);
color: var(--neutralColor);
}
.product-name {
color: var(--secondaryColor);
}
.price {
color: var(--lightPrimaryColor);
}
.plus {
color: var(--lightPrimaryColor);
}
.minus {
color: var(--lightPrimaryColor);
}
.quantity {
color: var(--secondaryColor);
}
.button {
background-color: var(--primaryColor);
color: var(--neutralColor);
}
We declared all the color values in the :root
selector and reused them throughout the code, making it easy to control all the element colors in one place. We can make users change the style to their preferred color or font if we integrate with JavaScript. Later in this article, we‘ll create a Custom styles webpage where users can customize the background and text color using CSS variables and JavaScript. Don’t worry if you are not familiar with the CSS variables. I will teach you how to use them right away.
How to set up CSS variables in a stylesheet
To set up CSS variables, we declare them and then access and use them in our code. Let’s get familiar with the syntax before learning to declare and access the variable.
Variables syntax
To create a variable, we first write two dashes(- -), followed by a name, a colon(:), a CSS value, and then closed with a semicolon(;). Like this:
--primaryColor: #393ef9;
How to declare CSS variables
To declare a variable, we can do that in the :root
selector.
Example:
:root {
--primaryColor: #393ef9;
}
How to access and use CSS variables
To access and use the CSS variable, we use the var()
function, and the var()
function allows us to insert the value of a CSS variable.
Example:
/*declaring CSS variables*/
:root {
--primaryColor: #393ef9;
--secondaryColor: #5f6068;
}
/*accessing and using CSS variables*/
.header {
/*Inserted the value of CSS variable with var() function*/
background-color: var(--primaryColor);
}
.product-name {
/*Inserted the value CSS variable with var() function*/
color: var(--secondaryColor);
}
Different ways to declare CSS variables
You can declare variables in two places.
- Global scope
- Local scope
Declaring CSS variables with global scope
When you declare CSS variables at global scope, it will be accessed and used throughout the document. Declare the variable at the :root
selector to make it a global variable.
Example:
:root {
--primaryColor: #393ef9;
}
Here, we declared the variables at the :root
selector. The :root
selector matches the document’s root element.
Declaring CSS variable with local scope
To create a variable with a local scope, declare it inside the selector that will use it.
.product-name {
--primaryColor: #5f58bf;
color: var(--primaryColor);
}
Only the selector .product-name
will use this variable --primaryColor
. No other selector can access and use the variable.
These are just the basics of CSS variables enough to get you started. You can read more about working with CSS variables, where Eze Thankgod explains it in greater detail.
Best practice for naming and organizing CSS variables
When naming and organizing CSS variables. Here are a few things to do:
- Use meaningful and descriptive names: Choose names that indicate the purpose or role of the variable. For example, instead of using “color1” or “var1” ” use names like “primaryColor” or “background-color” to name your variables.
- Be consistent: Use a consistent naming convention throughout your project. Consistent naming convention makes it easier to understand and maintain your code. You can use camel case, kebab case, or any other convention, but be consistent.
- Group-related variables: Organize your variables into logical groups based on their purpose. For example, group all color-related variables together, all typography-related variables together, and so on.
How to access CSS variables with JavaScript
CSS variables live in the DOM, which means we can access them with JavaScript. To access the value of variables, we first set it up in a stylesheet like this:
:root {
--primaryColor: #5f58bf;
}
Let’s access the variable value declared in the :root
selector above:
// Get the root element
const root = document.querySelector(":root");
//Get the style properties for the root
const style = getComputedStyle(root);
//Get the style value for the root
const primaryColor = style.getPropertyValue("--primaryColor");
//Checking if we have access to the CSS variable
console.log(primaryColor);
If you check your console, you should have the value #5f58bf
displayed to show that JavaScript has access to the variable.
This code allows us to access the value of the variable --primaryColor
declared in the root
selector. By using getComputedStyle
and getPropertyValue
, we can retrieve the value of the variable and store it in the primaryColor
. In this case, the value of primaryColor
would be #5f58bf
. We can now be able to update the value in JavaScript.
How to update CSS variables with JavaScript
To update CSS variables with JavaScript, we first set them up in a stylesheet, access them, and then update them.
For example:
<!doctype html>
<html>
<head>
<style>
:root {
--primaryColor: blue;
}
p {
color: var(--primaryColor);
}
</style>
</head>
<body>
<p>CSS variables</p>
</body>
<script>
//Get the root element
const root = document.querySelector(":root");
//Change the style value for the root
root.style.setProperty("--primaryColor", "pink");
</script>
</html>
In this code, we set the paragraph color to blue in a stylesheet and later changed it to pink with JavaScript.
Updating CSS variables with JavaScript could be helpful when you want to give users control over the CSS styling. To better illustrate this, let’s create a simple Custom styles webpage where users can customize the background and text color using CSS variables and JavaScript.
How to create a Custom Styles webpage
Users can customize the background and text color in this Custom Styles webpage. You can check out the demo project we are about to build below: Custom-style webpage
First, let’s set up the HTML structure:
<!doctype html>
<html>
<head>
<title>Custom Styles</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<h1>Welcome to Custom Styles!</h1>
<p>Change the default styles to your liking:</p>
<div class="color-picker">
<label for="bg-color">Background Color:</label>
<input type="color" id="bg-color" />
<label for="text-color">Text Color:</label>
<input type="color" id="text-color" />
</div>
<script src="index.js"></script>
</body>
</html>
Let’s create the stylesheet file style.css
to add style to our webpage:
:root {
--bgColor: #ffffff;
--textColor: #000000;
}
body {
background-color: var(--bgColor);
color: var(--textColor);
text-align: center;
font-size: 24px;
padding: 20px;
}
.color-picker {
margin-top: 20px;
}
Next, let’s create the JavaScript file index.js
to handle the user’s customization:
// Get the color picker inputs
const bgColorInput = document.getElementById("bg-color");
const textColorInput = document.getElementById("text-color");
// Update the CSS variables when the inputs change
bgColorInput.addEventListener("input", () => {
document.documentElement.style.setProperty("--bgColor", bgColorInput.value);
});
textColorInput.addEventListener("input", () => {
document.documentElement.style.setProperty(
"--textColor",
textColorInput.value,
);
});
In this JavaScript code above, we select the color picker inputs using their respective IDS. We then add event listeners to each input, so when users change the color, the corresponding CSS variable will update with the new value. For example, in the image below, the user changes the webpage’s default background and text color to blue and white, respectively.
You can download and reuse the project source code from the file repository.
One reason for updating CSS variables with JavaScript is to dynamically change the styling of elements based on user interactions or other events. Theme switcher is another good project example. A user can switch between different themes, and JavaScript can update the CSS variables for colors, fonts, and other styles based on the selected theme. You can use the link below, where Mr. Web Designer teaches how to build a theme switcher project on his YouTube channel to learn how to build the Theme switcher project.
Create a Theme Color Switcher using HTML, CSS, And Vanilla JavaScript.
Conclusion
By utilizing CSS variables and JavaScript, we can efficiently set up and update style values, reducing redundancy in our code. This approach allows for easier maintenance and flexibility when making design changes. Plus, integrating CSS variables with JavaScript gives users a greater level of control over the CSS styles of our web pages. By exposing certain CSS variables to the user interface, we can allow them to customize and personalize the website look according to their preference.
Gain control over your UX
See how users are using your site as if you were sitting next to them, learn and iterate faster with OpenReplay. — the open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.