Working with CSS variables
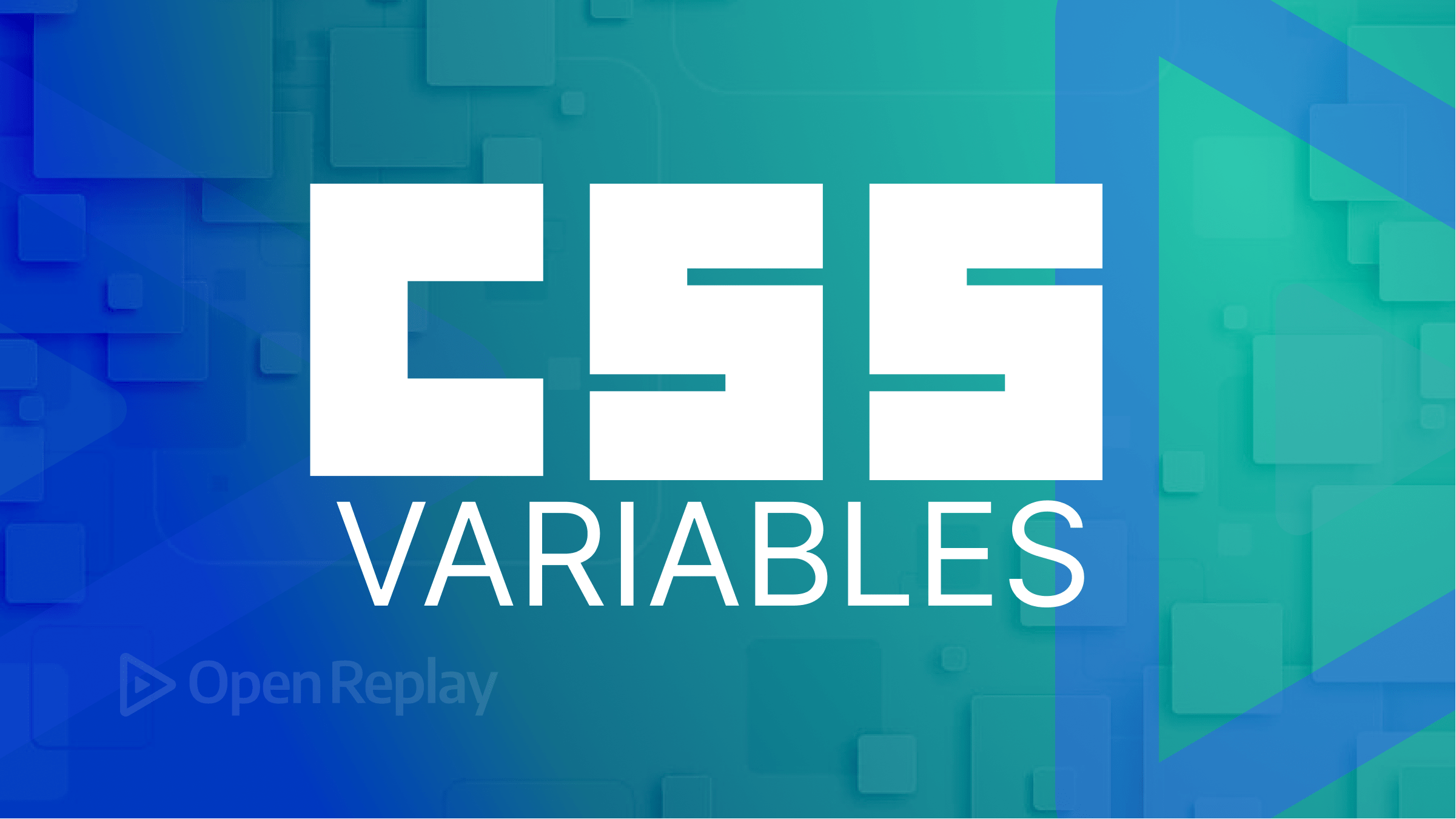
One of the usefulness of CSS variables is storing values such as colors, sizes, and other design elements in one place and then using them throughout your stylesheet. This article will teach you the meaning of CSS variables and how to make it easier to maintain and update your styles to improve your front-end development skills.
What are CSS variables?
The CSS variables, or custom properties, are an effective tool that allows you to define reusable values in your stylesheet and apply them throughout your project. You can use them in various contexts, including colors, lengths, fonts, etc. In addition, they allow you to store values in one place and reuse them throughout your stylesheet rather than repeating the same value multiple times. As a result, it can make your stylesheets more organized, maintainable, and easier to modify.
Browsers have different capabilities and may support different versions of web standards, so web developers must consider browser support when building and testing websites. To ensure that a website is compatible with a particular browser, web developers can use a combination of manual testing and tools such as browser compatibility testing platforms. However, it is also essential to remember that different versions of the same browser may have different levels of support for web standards, so testing multiple browser versions may be necessary. The following browsers support the CSS variables:
- Google Chrome
- Mozilla Firefox
- Microsoft Edge
- Apple Safari.
Why learn CSS variables?
There are several benefits to using CSS variables, and they include the following:
- They make it easier to maintain your stylesheets because you can define a value once and use it throughout your stylesheet.
- They allow users to customize a website’s appearance by changing the variables’ values.
- They can be helpful for websites that allow users to customize the color scheme or font size.
- They allow you to store and access data more efficiently.
- They prevent you from repeating the same values multiple times throughout your code.
- They make your code more straightforward and more effortless to read.
- They make it easier to update and maintain your code.
- They can help you organize your code by using descriptive names for your variables.
- They make it easier to understand what your code is doing and how different parts of the program are connected.
- They are essential for building more complex programs. As your program grows in size and complexity, you will need to use variables to store and manipulate data in various ways.
How to declare a CSS variable?
You can store values such as colors, font sizes, and other styles repeatedly used throughout a website. A CSS variable is defined using two dashes, --
, and to declare a CSS variable, you use the --
prefix followed by the variable name.
Example:
: root {
--main-color: blue;
}
The above example will create a variable called --main-color
with the value blue.
Referencing CSS variables
To use a variable, you can use the variable in your CSS by referencing it using the var() function in your CSS rules. For example:
: root {
--main-color: blue;
--txt-color: white;
}
body {
color: var(--main-color);
}
h1 {
color: var(--txt-color);
}
The above sets the color property of the body element to the value of the —main-color variable, blue. More so, select the color property of the h1 to the value of the —txt-color, white.
Declaring variables directly
You can declare variables directly in the style sheet of an element using the style attribute like this:
<div style="--main-color: blue;"></div>
With the above example, this element will have a blue color.
Inheriting CSS variables
It’s essential to note that CSS variables are inherited by default. Thus, if a CSS variable is not defined on an element, it will be inherited from its parent element. However, you can override inheritance by defining the variable on the component. Example:
: root {
--main-color: blue;
}
body {
color: var(--main-color);
}
In this example, the --main-color
variable is set on the root pseudo-class, representing the document’s root element. The body element then inherits this variable to set the text color to blue.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
How to use CSS variables?
To use CSS variables, you first need to define them using the — prefix followed by the variable name. For example:
<!DOCTYPE html>
<html>
<head>
<title>CSS Variables</title>
<style>
:root {
--main-color: blue;
--txt-color: white;
}
body {
background-color: var(--main-color);
}
h1 {
color: var(--txt-color);
}
h2{
border-bottom: 2px solid var(--main-color);
}
.container {
color: var(--main-color);
background-color: var(--txt-color);
font-size: 24px;
font-weight: bold;
padding: 30px;
}
button{
background-color: var(--txt-color);
color: var(--main-color);
font-weight: bold;
border: 1px solid var(--main-color);
padding: 5px;
}
</style>
</head>
<body>
<h1>Example of using CSS variable for color</h1>
<div class="container">
<h2>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor.</h2>
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum</p>
<p>
<button>Yes</button>
<button>No</button>
</p>
</div>
</body>
</html>
Here is the result:
The above example defines a variable called —main-color with a value of blue and —txt-color with a value of white. The: root pseudo-class refers to the document’s root element, which makes the variable available throughout the document.
Overriding the value of a CSS variable
To override the value of a CSS variable, you can set the variable’s value using the — prefix and the name of the variable, followed by the value you want to set. In addition, define it again in a different scope. For example:
<!DOCTYPE html>
<html>
<head>
<title>CSS Variables</title>
<style>
:root {
--main-color: blue;
--txt-color: white;
}
body {
background-color: var(--main-color);
--main-color:green;
}
h1 {
color: var(--txt-color);
}
h2{
border-bottom: 2px solid var(--main-color);
}
.container {
color: var(--main-color);
font-size: 24px;
font-weight: bold;
background-color: var(--txt-color);
padding: 30px;
}
button{
background-color: var(--txt-color);
color: var(--main-color);
border: 1px solid var(--main-color);
font-weight: bold;
padding: 5px;
}
</style>
</head>
<body>
<h1>Overriding CSS variables.</h1>
<div class="container">
<h2>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed.</h2>
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p>
<p>
<button>Yes</button>
<button>No</button>
</p>
</div>
</body>
</html>
Here is the result:
In this example, the value of the —main-color variable is blue by default but overridden to green for the body element.
Specifying a default value for the variable
You can specify a default value for the variable using the var() function. For example:
body {
color: var(--main-color, blue);
}
It will set the color property of the body element to the value of the —main-color variable.
Using CSS variables for font
You can store CSS variable values such as font sizes and other styles repeatedly used throughout your project.
<!DOCTYPE html>
<html>
<head>
<title>CSS Variables</title>
<style>
:root {
--font-size: 24px;
--font-weight: 800;
}
body{
border: 1px solid blue;
}
div{
font-size: var(--font-size);
font-weight: var(--font-weight);
}
p{
font-size: var(--font-size);
font-weight: var(--font-weight);
}
</style>
</head>
<body>
<h1>Example of using CSS variables for font:</h1>
<div> Lorem ipsum consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolor.</div>
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident.</p>
</body>
</html>
Here is the result:
Syntax of the var() function
The var()
function is a CSS function used to set the value of a custom property (also known as a “CSS variable”) to a specific value. It is used in a CSS rule to specify the custom property’s value.
The syntax for the var() function is as follows:
var(--custom-name, value)
- The
--custom-name
is the name of the custom property you want to set the value. It is a required parameter, and the variable name must start with two dashes--
. - The
value
is the value you wish to set for the custom property. If the custom property does not exist, it creates the specified value, and it is an optional parameter.
Scope with CSS variables
The CSS variables have a specific scope, determining where they can be accessed and used. The scope of a CSS variable is determined by where it is defined. Therefore, you can define CSS variables at the following levels:
- Global level: These CSS variables defined at the global level are accessible from anywhere in the document. They are defined outside of any selector using the: root pseudo-class.
- Local level: These CSS variables defined at the local level are only accessible within the element defined and its children. They are defined outside any selector or using the var function within a style rule.
How to define CSS variables at the global and local levels
To define a CSS variable at the global level, you can use the: root pseudo-class and set the value of the variable using the — syntax (). For example:
: root {
--main-color: red;
}
To define a CSS variable at the local level, you can use the var() function within a style rule for a specific element. For example:
button {
color: var(--main-color);
}
In the above examples, the --main-color
variable is defined globally and can be accessed anywhere in the document. On the other hand, the color: var(--main-color);
variable is determined at the local level and can be accessed only within the button element and its children.
Conclusion
The CSS variables are essential to store values such as colors, font sizes, and other design properties. With them, you can easily understand how your code functions and how different parts of the program are connected.
A TIP FROM THE EDITOR: For some more on writing modular, efficient CSS, don’t miss Using CSS Modules In React.