10 Practical Examples of using Day.js
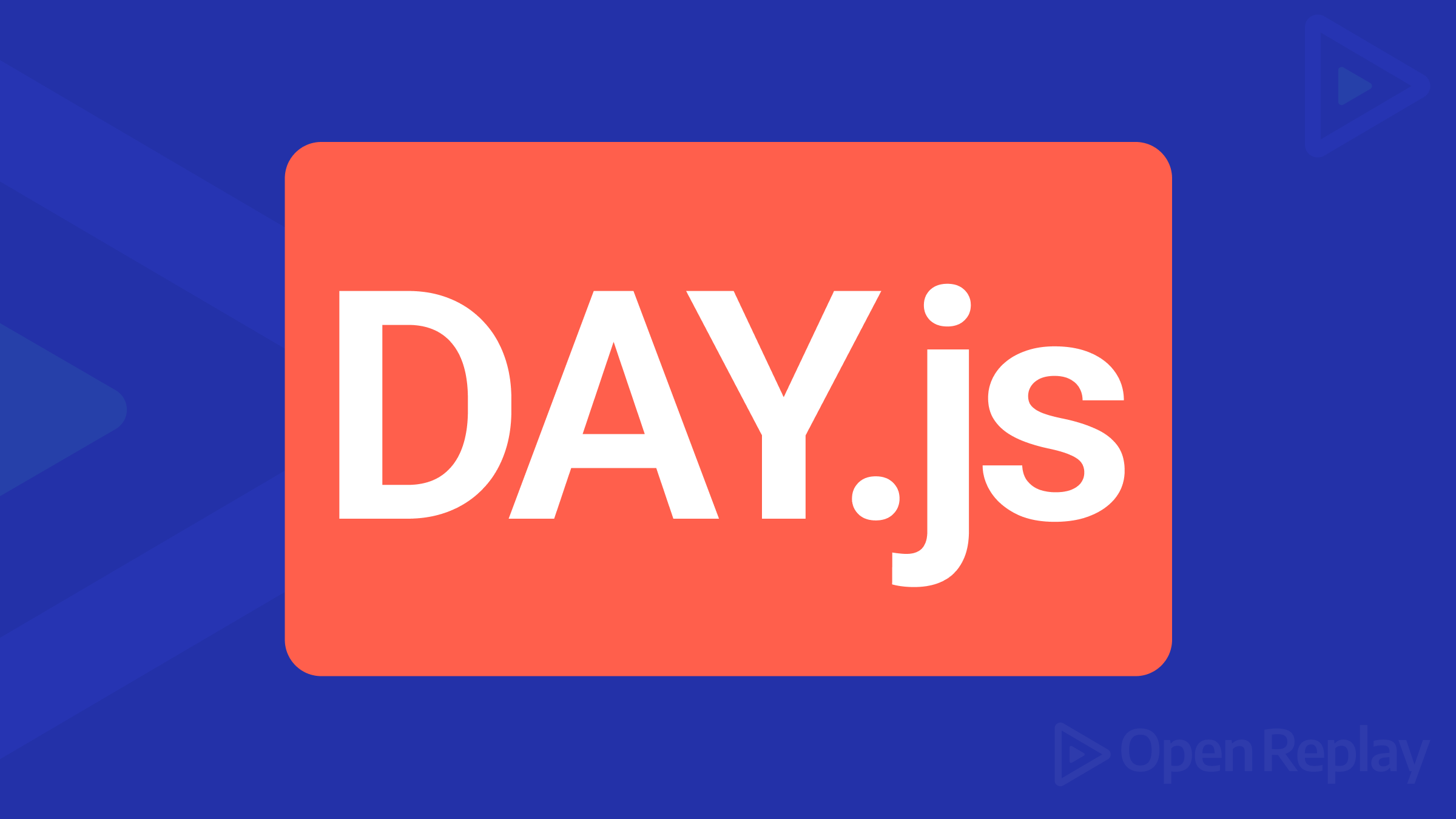
Managing dates and times is a fundamental aspect of many JavaScript web applications. That’s because they are essential for tracking activities, logging data, scheduling events, and providing prompt information to users. This article will show you ten examples of the powerful Day.js library for your own usage.
Discover how at OpenReplay.com.
Like all the other mainstay programming languages, JavaScript provides a way to handle dates and times. This is the date object:
const now = new Date();
console.log(now); // Mon Aug 05 2024 09:22:32 GMT+0100 (West Africa Standard Time)
The problem with the JavaScript built-in date object is that it’s inconsistent and cumbersome. Developers struggle with it because:
- it is error-prone
- it has a complex API
- it makes date manipulation difficult
- it’s a pain to work with time zones.
- and more
JavaScript developers used to rely on Moment.js to handle dates. It provided a better API but was heavy and got heavier after using it. The maintainers have also decided to deprecate it.
Day.js emerged as a lightweight alternative. It offers a simple, chainable API and efficient date formatting and manipulation, effectively addressing the issues with the built-in date object and Moment.js.
Day.js also makes it easy to transition from Moment.js because it offers a similar API. It has a rich plugin system and supports multiple locales out of the box. And because of its minimalistic and performant approach, it offers a better alternative to a large library like Moment.js and the built-in date object.
To start with Day.js, you can use a CDN from jsDelivr
or cdnjs
or install it on your Node JS project with your favorite package manager.
# CDNJS
<script src="https://cdn.jsdelivr.net/npm/dayjs@1/dayjs.min.js"></script>
# jsDelivr
<script src="https://cdn.jsdelivr.net/npm/dayjs@1.11.12/dayjs.min.js"></script>
# install with NPM
npm install dayjs
# Install with Yarn
yarn add dayjs
# Install with PNPM
pnpm add dayjs
Let’s look at ten practical ways you can use Day.js in your web projects.
Practical Example of Using Day.js #1: Formatting Dates for Display
One of the most practical uses of Day.js is to format dates for display. To do this, you need a dayjs()
instantiation variable:
const now = dayjs();
console.log(now);
This gives you an object. When you expand it, this is what it looks like:
You then have to chain the format()
method and pass in any of the following to format the date:
DD
: day of the month (01-31)MM
: month of the year (01-12)YYYY
: full yearHH
: hours (00-23)mm
: minutes (00-59)ss
: seconds (00-59)
The format you pass in depends on how date is formatted in the target locale.
Such formats include:
DD-MM-YYYY
: day-month-full yearMM-DD-YYYY
: month-day-full yearYYYY-MM-DD
: full-year-month-day
const now = dayjs().format('DD-MM-YYYY HH:mm:ss');
console.log(now); // 05-08-2024 10:33:58
You’re the decider of the separator, so you can use whatever you want:
// slash separator
const now = dayjs().format('DD/MM/YYYY HH:mm:ss');
console.log(now); // 05/08/2024 10:35:10
// dot separator
const now = dayjs().format('DD.MM.YYYY HH:mm:ss');
console.log(now); // 05.08.2024 10:35:38
You can take that further by updating the date and time in real time using the setInterval()
function.
Here’s the HTML code, an empty div
element styled with Tailwind CSS in which the time will be inserted into:
<div
id="current-time"
class="text-4xl font-bold text-gray-200 bg-gray-700 p-6 rounded-lg shadow-lg text-center"
></div>
And this is the JavaScript code:
function updateDateTime() {
const now = dayjs();
const formattedDateTime = now.format('YYYY-MM-DD HH:mm:ss');
document.getElementById('current-time').textContent = formattedDateTime;
}
// Update the date and time every second
setInterval(updateDateTime, 1000);
updateDateTime();
The code above is essentially a function that formats the date and inserts it into the HTML. The function is then referenced inside the setInterval
function and called under it.
Here’s the result now:
Here are other format strings you can use in:
YY
: two-digit yearM
: the monthMMM
: abbreviated month nameMMMM
: full month nameD
: day of the monthd
: day of the weekdd
: two-letter short name for the day of the weekddd
: three-letter short name of the day of the weekdddd
: full name of the day of the weekh
: the hour in (12-hour clock)hh
: the hour (2-digit 12-hour clock)m
: the minute (0-59)s
: the second (0-59)SSS
: three-digit millisecond (000-999)A
: AM/PMa
: am/pm
Practical Example of Using Day.js #2: Parsing Dates from Strings
In your web projects, you may need to parse dates that come from a database, an API, or user input.
Day.js lets you comfortably do that by calling the format()
method on the date. Here are some examples:
const date1 = dayjs('2024-08-05'); // YYYY-MM-DD format
const date2 = dayjs('08-05-2024'); // MM/DD/YYYY format
const date3 = dayjs('08-05-2024'); // DD/MM/YYYY format
const date4 = dayjs('2024-08-05T14:30:00Z'); // ISO format
console.log(date1.format('YYYY/MM/DD')); // 2024/08/05
console.log(date2.format('MM/DD/YYYY')); // 08/05/2024
console.log(date3.format('DD/MM/YYYY')); // 05/08/2024
console.log(date4.format('YYYY/MM/DD HH:mm:ss')); // 2024/08/05 15:30:00
Practical Example of Using Day.js #3: Comparing Dates
Comparing dates is another common requirement in many websites and apps. This could be needed for checking deadlines, validating expiration dates and ranges, or tracking deadlines.
For this purpose, Day.js provides methods like isBefore
, isAfter
, isSame
, and others. These methods return booleans (true
and false
).
Here’s a simple implementation of the isBefore()
and isAfter()
methods:
const date1 = dayjs('2024-12-25');
const date2 = dayjs('2025-01-01');
console.log(date1.isBefore(date2)); // true
console.log(date1.isAfter(date2)); // false
console.log(date1.isSame(date2)); // false
You can do something based on the resulting true
or false
:
const date1 = dayjs('2024-12-25');
const date2 = dayjs('2025-01-01');
if (date1.isBefore(date2)) {
console.log('Christmas comes before new year');
} else {
console.log('Christmas does not come before new year');
}
You need to load plugins for other comparison methods, such as isBetween()
, isSameOrBefore()
, isSameOrAfter()
, and isLeapYear()
.
Practical Example of Using Day.js #4: Calculating the Differences Between Two Dates
Day.js provides the diff()
method for calculating age or the number of days left until a deadline.
The diff()
method takes two arguments: the first required argument is the date you want to compare against, and the second is an optional unit of measurement.
Here’s a basic usage of the diff()
method:
const date1 = dayjs('2023-01-01');
const date2 = dayjs('2024-01-01');
const difference = date2.diff(date1, 'day');
console.log(difference); // 365 – a year
You can calculate this difference in other units like months, hours, minutes, and seconds by passing that unit into the diff()
method:
const date1 = dayjs('2023-01-01');
const date2 = dayjs('2024-01-01');
const date3 = dayjs('2023-04-04');
// Calculate the difference in months
const diffInMonths = date2.diff(date1, 'month');
console.log(`Difference in months: ${diffInMonths}`); // Difference in months: 12
// Calculate the difference in years
const diffInYears = date2.diff(date1, 'year');
console.log(`Difference in years: ${diffInYears}`); // Difference in years: 1
// Calculate the difference in hours
const diffInHours = date2.diff(date1, 'hour');
console.log(`Difference in hours: ${diffInHours}`); // Difference in hours: 8760
// Calculate the difference in minutes
const diffInMinutes = date2.diff(date1, 'minute');
console.log(`Difference in minutes: ${diffInMinutes}`); // Difference in minutes: 525600
// Calculate the difference in seconds
const diffInSeconds = date2.diff(date1, 'second');
console.log(`Difference in seconds: ${diffInSeconds}`); // Difference in seconds: 31536000
Here’s a way you can calculate birthday:
const birthday = dayjs('1991-01-27');
// Get the current date
const today = dayjs();
// Calculate the age in years
const age = today.diff(birthday, 'year');
console.log(`John Doe is ${age} years old`); // John Doe is 33 years old
Practical Example of Using Day.js #5: Adding and Subtracting Time
There’s an add()
and a subtract()
methods you can use to make calculations while setting deadlines, scheduling, or calculating past or future dates.
Here’s how you can add time:
// Create a date
const date = dayjs('2024-08-05');
// Add 7 days to the date
const newDate = date.add(7, 'day');
// format the dates in "ddd, D MMM" format
const formattedOriginalDate = date.format('ddd, D MMM');
const formattedNewDate = newDate.format('ddd, D MMM');
console.log(formattedNewDate); // Mon, 12 Aug
console.log(`A week from ${formattedOriginalDate} is ${formattedNewDate}`); // A week from Mon, 5 Aug is Mon, 12 Aug
And here’s how you can subtract time:
const date = dayjs('2024-08-05');
// Subtract 7 days from the date
const newDate = date.subtract(7, 'day');
// format the dates in "ddd, D MMM" format
const formattedOriginalDate = date.format('ddd, D MMM');
const formattedNewDate = newDate.format('ddd, D MMM');
console.log(formattedNewDate); // Outputs: Mon, 29 Jul
console.log(`A week before ${formattedOriginalDate} is ${formattedNewDate}`); // A week before Mon, 5 Aug is Mon, 29 Jul
Practical Example of Using Day.js #6: Working with Timezones
To work with timezones, you have to include the UTC
and timzone
plugin CDNs in your HTML:
<script src="https://cdn.jsdelivr.net/npm/dayjs@1/plugin/utc.js"></script>
<script src="https://cdn.jsdelivr.net/npm/dayjs@1/plugin/timezone.js"></script>
You should then load both plugins in your JavaScript file like this:
dayjs.extend(dayjs_plugin_utc);
dayjs.extend(dayjs_plugin_timezone);
You can then use the date.tz()
method and pass in the timezone you want, relative to a certain date or time:
dayjs.extend(dayjs_plugin_utc);
dayjs.extend(dayjs_plugin_timezone);
const date = dayjs('2024-08-05T13:30:00Z');
const NewYorkTime = date.tz('America/New_York');
const UruguayTime = date.tz('America/Montevideo');
const UKTime = date.tz('Europe/London');
console.log(
`Original date in UTC: ${date.format('ddd, D MMM YYYY HH:mm:ss [UTC]')}`
); // Original date in UTC: Mon, 5 Aug 2024 14:30:00 UTC
console.log(
`Time in New York: ${NewYorkTime.format('ddd, D MMM YYYY HH:mm:ss')}`
); // New York Time: Mon, 5 Aug 2024 09:30:00
console.log(
`Time in Uruguay: ${UruguayTime.format('ddd, D MMM YYYY HH:mm:ss')}`
); // Uruguay Time: Mon, 5 Aug 2024 10:30:00
console.log(`Time in UK: ${UKTime.format('ddd, D MMM YYYY HH:mm:ss')}`); // UK Time: Mon, 5 Aug 2024 14:30:00
Practical Example of Using Day.js #7: Validating Dates
In some situations, you would have to make sure a date entered by the user is valid and even validate dates from a database or API. There’s an isValid()
method for this purpose.
This would help prevent errors in date calculations and ensure the correct date is added to the database.
What you need to do is call the method on that date, as you can see below:
console.log(dayjs('2024-02-29').isValid()); // true
console.log(dayjs('2025-02-29').isValid()); // true
console.log(dayjs('this is a string').isValid()); // false
console.log(dayjs('20243-14-67').isValid()); // false
Unfortunately, the isValid()
method does not perform strict validation of date strings. For example, 2025 is not a leap year, so a date like 2025-02-39
should return false
, but it returns true
:
console.log(dayjs('2024-02-29').isValid()); // true – 2024 is a leap year so is indeed true
console.log(dayjs('2025-02-29').isValid()); // true – 2025 is not a leap year so should be false
To fix this, you can use the customParseFormat
plugin with the isValid()
method.
Include the CDN in your HTML:
<script src="https://cdn.jsdelivr.net/npm/dayjs@1/plugin/customParseFormat.js"></script>
Load the plugin:
dayjs.extend(dayjs_plugin_customParseFormat);
Use strict parsing by passing in the format and true
to the dayjs()
standard method:
console.log(dayjs('2024-02-29', 'YYYY-MM-DD', true).isValid()); // true
console.log(dayjs('2025-02-29', 'YYYY-MM-DD', true).isValid()); // false
Practical Example of Using Day.js #8: Getting the Start or End of a Period
You would need to know the start or end of a year, month, or week to manage recurring events and subscriptions or assign a task.
Day.js provides the startOf()
and endOf()
methods for those purposes. Both methods take period you want to get the start and end for (year, month, week, and day).
//Get the current date and time
const now = dayjs();
// Get the start of the day
const startOfDay = now.startOf('day');
console.log(`Start of the day: ${startOfDay.format('YYYY-MM-DD HH:mm:ss')}`); // Start of the day: 2024-08-05 00:00:00
// Get the start of the week
const startOfWeek = now.startOf('week');
console.log(`Start of the week: ${startOfWeek.format('YYYY-MM-DD HH:mm:ss')}`); // Start of the week: 2024-08-04 00:00:00
// Get the start of the month
const startOfMonth = now.startOf('month');
console.log(
`Start of the month: ${startOfMonth.format('YYYY-MM-DD HH:mm:ss')}`
); // Start of the month: 2024-08-01 00:00:00
// Get the start of the year
const startOfYear = now.startOf('year');
console.log(`Start of the year: ${startOfYear.format('YYYY-MM-DD HH:mm:ss')}`); // Start of the year: 2024-01-01 00:00:00
Practical Example of Using Day.js #9: Working with Relative Time (Displaying dates relative to the current time)
Displaying dates relative to the current time is useful in social media apps, messaging apps, blogging platforms, and notifications.
For this, Day.js has the from()
, fromNow()
, to
(), and toNow()
methods through the relativeTime
plugin.
from
is from the future, like in X days
, and to
is to the future, like 4 days ago
.
To display relative time, the first thing you need to do is to include the Day.js relativeTime
plugin CDN in your HTML:
<script src="https://cdn.jsdelivr.net/npm/dayjs@1/plugin/relativeTime.js"></script>
You then have to load the CDN in your JavaScript file or a script you have within your HTML:
dayjs.extend(dayjs_plugin_relativeTime);
from
returns the relative time from a given date to another date:
const today = dayjs('2024-08-05');
const aPastDay = dayjs('2024-08-01');
console.log(today.from(aPastDay)); // in 4 days
fromNow()
returns the relative time from a given date to the current date and time:
const today = dayjs('2024-08-04');
console.log(today.fromNow()); // 2 days ago
to
returns the relative time to a given date from another date:
const today = dayjs('2024-08-05');
const aPastDate = dayjs('2024-08-01');
console.log(today.to(aPastDate)); // 4 days ago
toNow
returns the relative time to a given date from the current date and time:
const today = dayjs('2024-08-04');
console.log(today.toNow()); // in 2 days
Practical Example of Using Day.js #10: Handling Leap Year
Day.js provides an isLeapYear()
method with which you can check whether a year is a leap year or not. It returns true
if the year is a leap year and false if not. This could be useful for a calendar or date validation.
To use the isLeapYear()
method, you have to include the isLeapYear
plugin CDN in your HTML and load it in your script. Here’s how to do that:
<!-- Include the CDN -->
<script src="https://cdn.jsdelivr.net/npm/dayjs@1/plugin/isLeapYear.js"></script>
// Load the plugin
dayjs.extend(dayjs_plugin_isLeapYear);
After doing that, you can call the isLeapYear()
method:
const isLeapYear = dayjs('2024-03-22').isLeapYear();
console.log(isLeapYear); //true
const isNotLeapYear = dayjs('2019-01-01').isLeapYear();
console.log(isNotLeapYear); // false
Conclusion
You’ve seen how powerful, flexible, and at the same time, simple it is to handle dates and times with Day.js. It helps perform a lot of tasks like formatting dates, comparing dates, and even figuring out differences between two dates. There’s no doubt that it’s a good choice for your project any day, any time.
In addition, you’ve seen how less cumbersome it is to validate dates, work with timezones, and display relative time. Imagine if you attempt to do this with the JavaScript date object.
The next thing from here is to start using Day.js in your projects by installing the NPM package or including and loading the CDN.
To learn more about Day.js, check out the official documentation.
Understand every bug
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — the open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.