The Art of Writing Good Code Comments
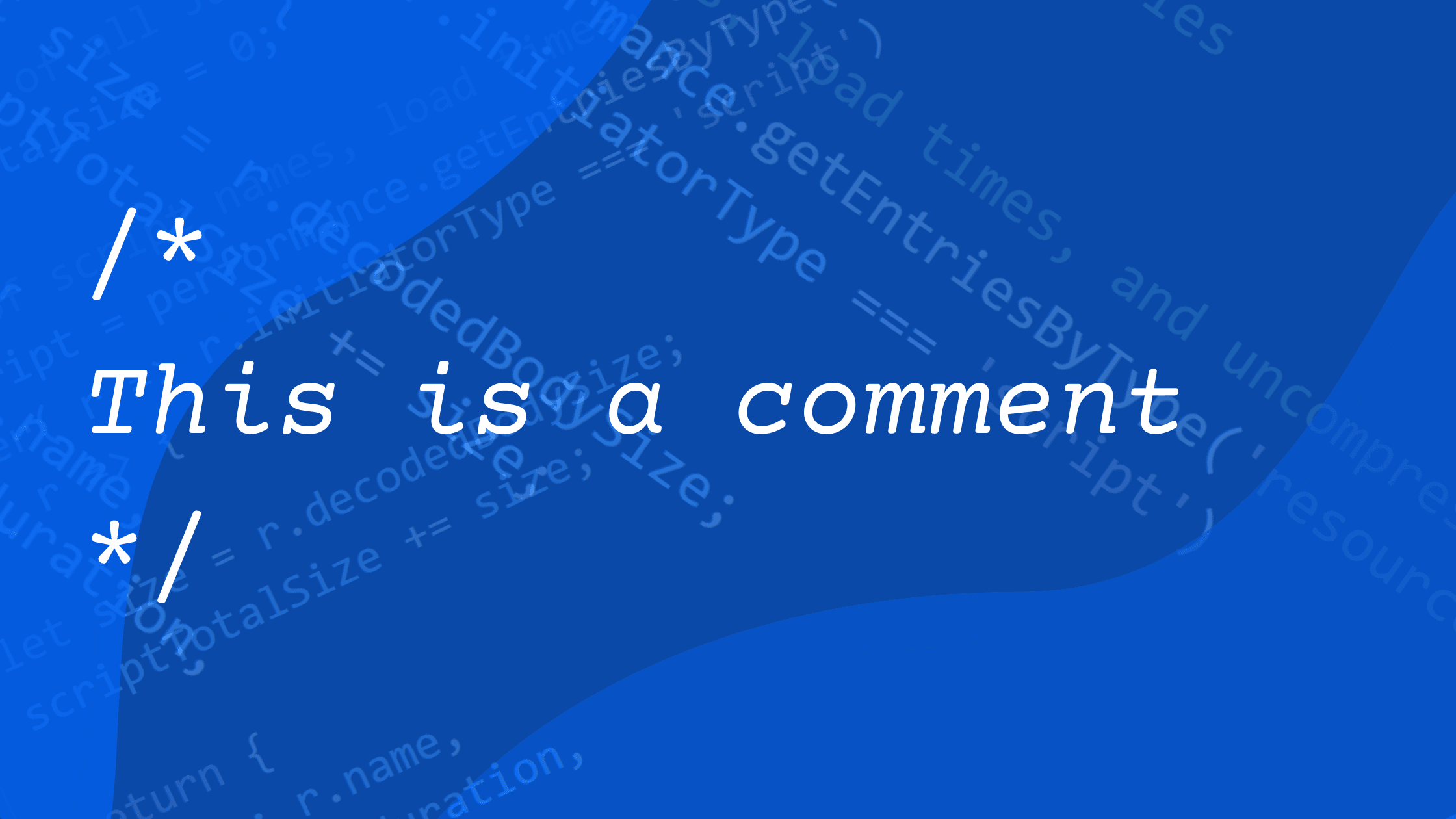
Not many developers like writing code comments. Moreover, they don’t find it a valuable use of their time. We’ve created this blog post to change your mind.
The biggest problem with writing code comments is that developers don’t understand the returned value of adding them. For that reason, it makes sense that they don’t value this skill as they haven’t experienced its value first-hand.
On top of that, it’s not that easy to convince developers to learn the craft of writing meaningfully and contextually comments. Several influential software engineers have been talking about self-explanatory code. In other words, any developer should be able to understand your code by reading it. That means that you have to write very clean code, for instance, using descriptive function names. But often, it’s not possible to create 100% readable code because a specific complex logic requires a more advanced architecture.
If someone can’t understand your code by reading it the first or second time, it’s considered a red flag for bad code. I’m afraid I have to disagree with this opinion as there are many scenarios where you want to add that little extra context for your colleagues. Not to mention junior developers who have less experience grasping the full context of a codebase. You can help these junior developers a lot by adding context to your code. It’s free, and requires little time! On top of that, it boosts the onboarding process for any developer.
So, let’s discuss the following topics related to “the art of writing code comments”:
- Purpose of context in code comments
- Levels of code commenting
- Tips for writing better code comments
- Improve your code commenting skills
The purpose of context in code comments
Context is an often-overlooked element when writing code comments, and it’s an important one! Context refers to adding relevant information to your code to help another engineer get a deeper understanding. Adding context doesn’t refer to adding information a developer can deduct from your code.
For instance, you’ve created a function called createNote
, which creates a new “note” object. If we add the following comment, “function to create new note object,” it doesn’t add any value as it’s information the developer can deduct from reading the code. Moreover, it’s a very straightforward function that creates new objects. Thus, it doesn’t require additional code comments.
Assume we are using a more advanced function that takes care of public-private key encryption. We can add several pieces of information to guide a developer:
- Explain the cryptographic package that’s being used
- Explain how the function transforms input
- Provide examples of how to use this function and what output to expect
I’m a big fan of adding small examples that show the input and output of a more complex function. It provides a lot of direct information to other engineers reading your code. They don’t have to run your code to understand how it works because they can directly learn from your example, which is a huge time-saver!
Which types of code comments can you use?
There are three main types of code commenting. However, many other approaches do exist but fall within the following categories:
- Document-level code commenting
- Function-level code commenting
- Logic-level code commenting
If we look at document-level code commenting, it’s a more generic type of comment that explains the functions you find in a document. Other than that, you can add information about the architecture or component the document lives in. The primary purpose of document-level commenting is adding high-level information about your code.
Next, function-level code commenting is the most helpful type of comment. The goal of function-level code commenting is to add context to functions. It’s essential not to overuse function-level commenting because many functions are self-explanatory and don’t require additional context. Only add comments to functions that require added context to speed up other developer’s learning curves.
Lastly, you can make use of logic-level code commenting. Obviously, it’s the lowest level of code commenting that you can use. The goal of logic-level comments is to clarify a complex code snippet. Make sure to only use logic-level comments for complex code paths because they can quickly bloat your code and decrease the readability.
Tips when writing code comments
As mentioned before, context is king, also for code commenting! Ask yourself ‘why’ you have solved a problem in a particular way. This question will help you to describe your code better and add valuable context.
Moreover, it’s important to write code comments while writing code. Don’t complete your code before writing any code comments. It’s much easier to capture the context while you are actively working on your code. Many developers prefer to finish their code and then add the comments. Often, these comments are less accurate or valuable because the context is missing.
Besides that, make sure not to overuse code comments. First of all, adding many comments will make your code appear bulky and harder to digest by another engineer. Not every function needs additional clarifications. You should add context to more valuable functions, like functions containing important business logic. Ideally, you want to find the right balance when writing code comments.
Lastly, many languages offer best practices or standards for code commenting. For instance, JSDoc is a popular commenting standard for the JavaScript community. Additionally, many IDEs understand the JSDoc standard, and therefore, can offer additional clarifications while writing code. For example, explain the use and type of a variable when passing it to a function.
/**
* Represents a book.
* @constructor
* @param {string} title - The title of the book.
* @param {string} author - The author of the book.
*/
function Book(title, author) {
// code
}
This is how an IDE such as Visual Studio Code renders this information automatically.
As an additional benefit, most developers understand JSDoc. It’s a shared language for quickly passing information and context about code or a specific function. How to refine your art of writing code comments? Developers like to give feedback during the code review process. However, not many developers bother to check the quality of code comments. Make sure to pay attention to your colleagues’ code comments and give them feedback. For instance, you can get started with flagging useless code comments that don’t add any context. It’s a great exercise for developers to learn what’s important and what’s not.
Additionally, you can create an extra checklist item in your “definition of done” to review pull requests.
If you want to improve your code commenting, start by looking up popular GitHub repositories and reviewing their style of code commenting. It’s a great place to learn how other developers tackle this form of art.
Open Source Session Replay
OpenReplay is an open-source, session replay suite that lets you see what users do on your web app, helping you troubleshoot issues faster. OpenReplay is self-hosted for full control over your data.
Start enjoying your debugging experience - start using OpenReplay for free.