Top 5 alternatives to Webpack
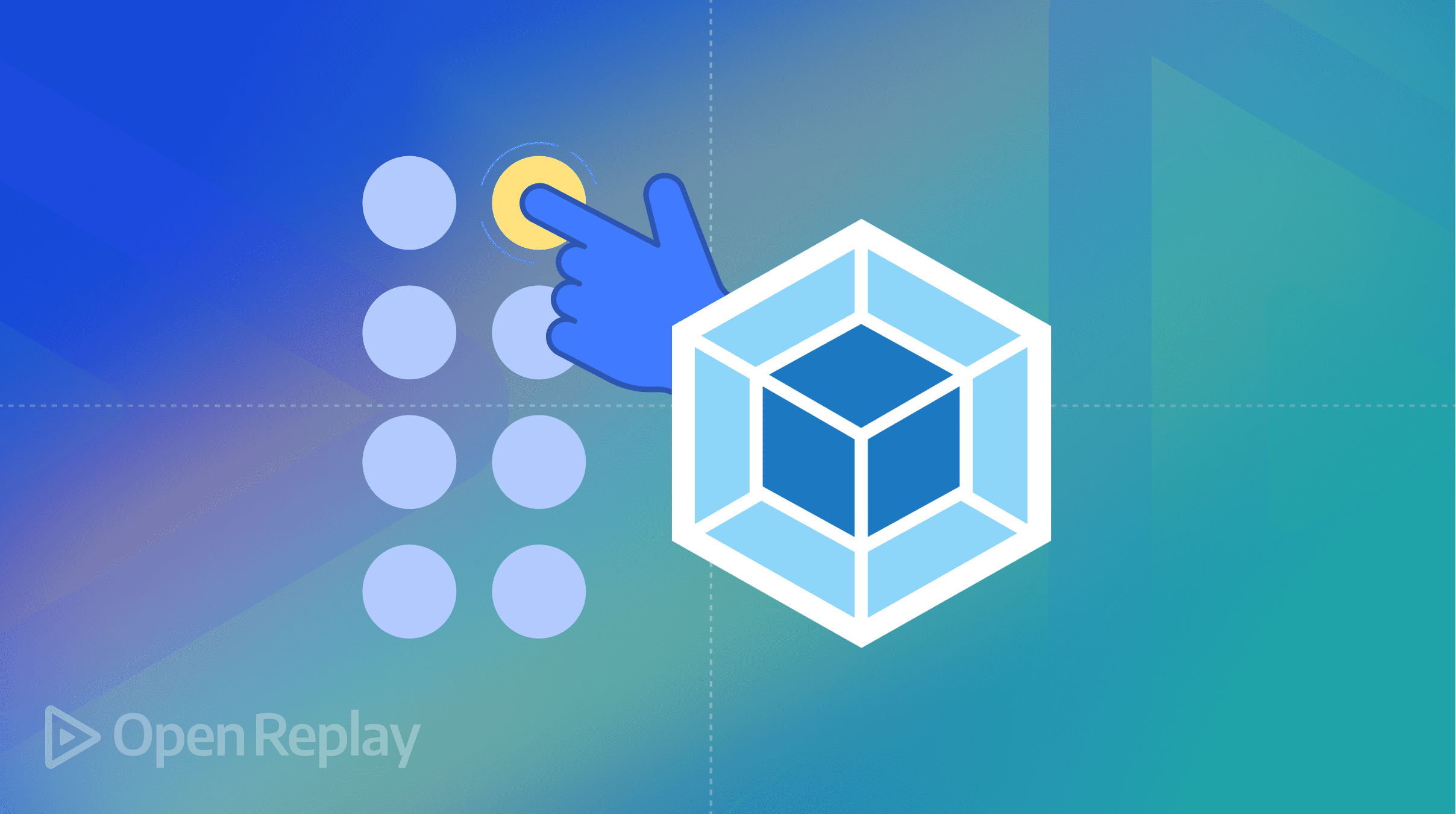
Developing a website or web app requires many packages and dependencies, such as Javascript tools like React and Angular, CSS tools like Sass or Less, and HTML tools like pug
. It is therefore a good practice to separate your code into smaller manageable chunks/files.
However, manually getting these separate files together during production can be a problem, as it could slow down your application, hence the need for bundlers. A bundler is a tool used to solve this latency issue by combining and merging your code into a single file.
Module bundlers are a way to organize and combine many files of JavaScript code into one file, making it easier and faster for them to run in a browser. They help you manage the dependency relationship of your code and load modules in dependency order for you- image asset, CSS asset, etc. A JavaScript bundler can be used when your project becomes too large for a single file or when you’re working with libraries that have multiple dependencies.
A popular and widely used bundler is Webpack, a module bundler made purposely for JavaScript applications. In this article, we will focus on Webpack and its top alternatives.
Webpack is a free and open-source module bundler for JavaScript applications. It is used to bundle JS files for usage in a web browser, and it can transform front-end assets such as HTML, CSS, and images if corresponding loaders are included.
Webpack works by processing your application and internally building a dependency graph which maps every module your project needs and generates one or more bundles, often just a single bundle.js file that can be plugged into the browser easily and used for the application.
It is important for pulling your resources together and providing code splitting. It is also helpful in converting embedded images into data (URL) and may perform tree shaking.
While we would like to go on and talk more about Webpack, our primary focus is to introduce you to the various alternatives to Webpack. However, you can look up the documentation for more details on the bundler. Several module bundlers provide the same functionality as Webpack. Here are the top five(5) bundlers you can use instead of Webpack:
Browserify
Browserify is an open-source JavaScript bundler tool that allows you to use Node.js modules directly in the browser. Thus, with Browserify you can write code that uses require()
in the same way you would in Node. It integrates well with npm and allows you to bundle your JavaScript dependencies into one file that can be referenced within a <script>
tag.
Features of Browserify:
Here are some notable features of browserify
- Lets you use a node-style require() to organize your browser code.
- Comes with an in-built automatic build system that makes building modules fast and straightforward.
- Provides straightforward npm integrations that allow you to reuse your node code without needing a native CLI.
- Integrates with tools like Babel, jsdom, imageboss, etc.
Basic configuration
To get started, install Browserify using the command below:
npm install browserify
First, create a main.js
file, then build a new JavaScript file with all of the attached modules using the command below:
browserify js/main.js -o js/findem.js
This command reads your main.js
file and outputs it into the findem.js
file defined by the `-o’ option.
Look up the documentation for more details on the bundle.
ESBuild
ESBuild is an extremely fast JavaScript/CSS bundler written in Golang. It is a CLI, NPM package, and Go module that makes bundling JavaScript accessible and fast. The main goal of this project is to bring about a new era of build tool performance and create an easy-to-use modern bundler along the way.
It is particularly known for its speed and is considered to be faster than other build tools. It has been described to be a linker for the web, meaning it can be used to quickly link JavaScript (JS, JSX, TS, and TSX) and CSS dependencies as deployable assets for the web, and this can be done by bundling, code-splitting, using plugging, e.t.c.
Here are some of the notable features of ESBuild:
- Extremely fast and doesn’t require a cache
- Supports ES6 and CommonJS modules
- Can perform tree shaking of ES6 modules
- Includes an API for JavaScript and Go
- Supports TypeScript and JSX syntax
Basic configuration
To get started, download and install ESBuild locally using the command below:
npm install esbuild
For your first bundle, create a file called app.ts
, then input this code in it:
let info: string = "Hello, esbuild!";
console.log(info);
You can bundle the TypeScript code via CLI:
esbuild app.ts --outfile=output.js --bundle
This command accepts the app.ts
file as the entry point and outputs it to the output.js
file.
You can look up the documentation to get more details on the bundle.
Parcel
Parcel is an open-source bundler written in Rust, with support for many popular languages like Typescript and SASS, and file types like images, font, and videos. It is considered a beginner-friendly bundler as it requires zero configurations, and it builds your code in parallel using worker threads, utilizing all of the cores on your machine.
Notable features of Parcel include:
- Requires zero configuration
- Integrates with React Fast Refresh and the Vue Hot Reloading API to automatically preserve your application state between updates
- Includes an out-of-the-box development server
- Provides hot reloading, i.e., it automatically updates your code in the browser
- Comes with in-built diagnostics to notify you of errors in the terminal or browser.
Basic configuration
To get started, install Parcel with the following command:
npm install --save-dev parcel
Next, create an HTML file and JavaScript file in place, and start Parcel’s built-in development server by running the following command:
npx parcel src/index.html
This starts the server and tells it what file to use as the entry point. Open http://localhost:1234/ in your browser to view what you’ve built so far. You can look up the documentation for more information on the bundle.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
Rollup.js
According to the Rollup official documentation, “Rollup is a module bundler for JavaScript which compiles small pieces of code into something larger and more complex, such as a library or application.” Also considered the next-generation ES module builder, this bundler allows developers to write future-proof code using the new ESM (EcmaScript Modules) format, using import and export included in the ES6 revision of JavaScript instead of CommonJS or AMD.
Notable features of Rollup include:
- Optimizes ES modules for faster native loading in modern browsers
- Compiles your code to other formats such as CommonJS modules, AMD modules
- Can import existing CommonJS modules through a plugin
- Can perform three shaking
Basic configuration:
To get started, install Rollup with the following command:
npm install --global rollup
For a basic browser bundle:
# compile to a <script> containing a self-executing function ('iife')
rollup main.js --file bundle.js --format iife
This command takes the main.js
as the entry point of your application and compiles all imports into a single file named bundle.js
.
Look up the documentation for more details on the bundle
Vite.js
Vite is a next-generation front-end tooling created by Evan You (creator of Vue) that provides a lightning-fast dev server, bundling, and a great developer experience. It uses esbuild for pre-bundling dependencies during development, making it faster than other module bundlers. It doesn’t bundle projects in development environments. Instead, it uses native ES module imports and is also highly extensible via its Plugin API and JavaScript API with full typing support.
Here are some of the notable features of Vite:
- Support for CSS Preprocessors, PostCSS, and CSS modules
- Support for .tsx and .jsx files using ESBuild for transpilation
- Supports popular front-end libraries like React, Vue, and Vanilla JS through templates
- Vite provides a Hot module replacement (HMR) API over native ESM
- It includes built-in Typescript, JSX, and Sass support
Basic Configuration
To get started, create a Vite application using the following command:
npm create @vitejs/app my-vite-app
NB “my-vite-app” is the name of your Vite application.
Next, run the following commands to finish the installation:
cd vite_application
npm install
Then enter this command to start the development server:
npm run dev
Look up the documentation for more information on this bundle.
Conclusion
Module bundlers are integral to web development as they provide reliable solutions for transforming and bundling application code to create static assets. Knowledge of various bundlers and their different features provides you with options on what bundler best fits your project.