Top Visual Studio Code Extensions for React developers
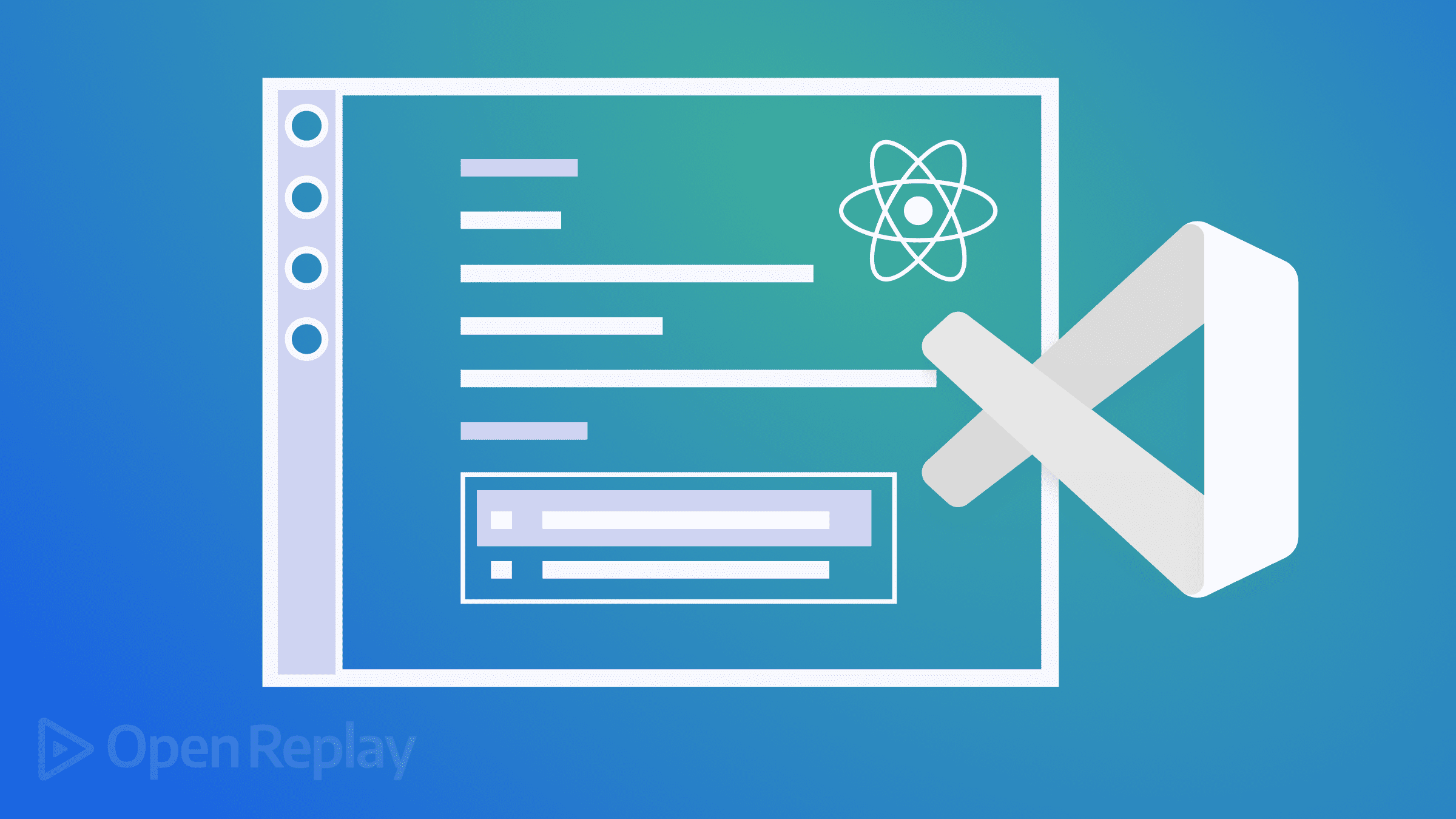
Over time, Visual Studio developed to become the greatest and most widely used text editor for developing any web application with many programming languages. Visual Studio Code is the most popular text editor among developers, With more than 13 million users globally. Because it is completely functioning right out of the box, most developers underutilize it. You can download VS Code and begin creating applications and APIs, essentially plug-and-play.
This article is for you if you are a React developer who has been using the VS Code IDE for projects and looking to take advantage of using extensions to speed up your productivity. My primary motivation for including such extensions is to improve the process because doing so can raise productivity. I’ll go through the best extensions to help you speed up the process of developing apps for the framework.
Snippets for ES7/React/Redux/GraphQl/React-Native
This extension provides JavaScript and React/Redux snippets. Here is the link for the marketplace ES7/React/Redux/GraphQl/React-Native snippets.
These extensions support languages like JavaScript, JavaScript React, Typescript, and TypeScript React. Below are the most commonly used prefix methods that you can use for your React apps.
React Components:
rcc
import React, { Component } from 'react'
export default class Demo extends Component {
render() {
return <div>Hello, World</div>
}
}
rce
import React, { Component } from 'react'
export class Demo extends Component {
render() {
return <div>Hello, World</div>
}
}
export default Demo
There are other great shortcuts that you can use most, like-
React
Prefix | Method |
---|---|
imrd→ | import ReactDOM from ‘react-dom’ |
imr→ | import React from ‘react’ |
Redux
Prefix | Method |
---|---|
rxreducer→ | redux reducer template |
rxaction→ | redux action template |
Snippets for Typescript React Code
This extension contains snippets of React typescripts.
Installation: You can install this extension using the Command Palette (Ctrl + Shift + P) or ( Cmd + Shift + P) and type the extension’s name.
This extension supports languages like Typescript(.ts) or TypeScript React (.tsx)
Here are the two snippets for creating react components with TypeScript.
-
Default Export React
-
Export React Component
Simple React Snippets
As we all know, snippets are the solution to speed up your development. So, Simple React Snippets for VS Code was built by Burke Holland.
Here, imrc
gets expanded to import React,{Component} from React
;
After installing your VS Code Extension, you can use the snippets by typing the shortcuts and tab or enter.
Here are some of the examples mentioned below:
imr - Import React
import React from 'react';
imrc - Import React and Component
import React, {Component} from 'react';
You can also check out more snippets by following these links.
ESLint
You can integrate your ESLint on your VS Code IDE. If you are new to ESLint, check out this documentation.
If you haven’t installed ESLint either locally or globally. You can use the command npm install eslint
in the workspace folder for the locally installed; for a global install, use npm install -g eslint
.
For a new folder, you need to create a .eslintrc configuration file. You can do this by running the command eslint
in your terminal.
After installing your Eslint locally, run the command .\node_modules\.bin\eslint --init
under Windows.
If you are using version < 2.x, then follow these steps - This extension contributes the following variables to the settings.
-
eslint.enable:
It enables or disables ESLint for the workspace folder -
eslint. debug:
It enables the ESLint’s debug mode (same as —debug command line option) -
eslint.lintTask.enable:
whether the extension contributes a lint task to lint a whole workspace folder: Command line options applied when running the task for linting the whole workspace (https://eslint.org/docs/user-guide/command-line-interface) -
eslint.lintTask.options:
control the package manager to be used to resolve the ESLint library. -
eslint.packageManager:
options to configure how ESLint is started using either the ESLint class API or the CLIEngine API. -
eslint.run:
run the linter onSave or onType; default is onType.eslint.quiet:
it lets you ignore the warnings.
React PropTypes Intellisense
This extension lets you find the React PropTypes in your file and add them to the suggestion list.
PropTypes is a runtime type checking for React Props and other similar objects. You can use it to document the types of properties passed to components. PropTypes is frequently used with React components and was first made available as part of the React core module. PropTypes provide a means for validating the data you receive.
Type-checking will help you find many flaws as your software develops. You can type-check your entire program for some apps using JavaScript extensions like Flow or TypeScript. React includes several built-in type-checking capabilities; however, you don’t have to utilize them. You may assign the unique propTypes property to type-check on the props for a component.
It works with all the implementations of the “PropTypes” feature, i.e., Static PropTypes, the prototype from a prototype.
If you are unaware of PropTypes, refer to this typechecking prop types will help you understand.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
Material Icon Theme
The Material Icon Theme for Visual Studio Code is fantastic. It’s incredibly eye-catching and facilitates finding whatever I need inside the explorer rapidly in my mind.
You can customize your folder color using the command palette or the user settings "material-icon-theme. folders.color": "#ef5350",
Here are the commands you can use as per your wish.
Press Ctrl-Shift-P to command palette and type the material Icons to get the list from the dropdown.
For more information, visit Marketplace Extension.
GitLens: Git supercharged
GitLens enhances Git in VS Code and releases hidden data from every repository. With the aid of Git blame annotations and CodeLens, you can quickly see who wrote a piece of code, navigate and explore Git repositories with ease, obtain insightful information through rich visualizations and strong comparison commands, and do so much more.
It is an open-source code extension for the VS code IDE tools. Gitlens lets you understand the code better. GitLens is robust, packed with features, and incredibly flexible to match your needs.
Here are the features of GitLens that provide:
- simple backward and forward revision navigation via a file’s history
- An unobtrusive current line blame annotation at the end of the line that displays the commit and author who most recently updated the line and provides more specific blame information when hovered over
- authorship CodeLens displays the number of authors and most recent commit at the top of files and on code blocks.
Commit Graph: You can quickly see and keep tabs on all active work using the Commit Graph.
Visual File History view: You can easily observe a file’s development in the Visual File History view, including when modifications were made, how big they were, and who made them.
You can follow this link for more information about the GitLens.
Code Formatter: Beautify
In Visual Studio Code, Beautify may be applied to javascript, JSON, CSS, Sass, and HTML.
To add a beautify shortcut to keybindings, use the procedure below. JSON. Replace with the keybindings of your choice.
{
"key": "cmd+b",
"command": "HookyQR.beautify",
"when": "editor focus"
}
Code Formatter: Prettier
It maintains a consistent style by analyzing your code and reprinting it according to its standards, which consider the maximum line length and wrap code as appropriate. This extension will use the local dependencies to be prettier in your project.
The extension can additionally try to resolve global modules if prettier.resolveGlobalModules is set to true. The version of prettier included with the extension will be used if prettier is not already installed locally with your project’s dependencies or system-wide.
Whether running a locally or globally resolved version of Prettier, this extension supports Prettier plugins. This extension will try to register the language and offer automated code formatting for the built-in and plugin languages.
Conclusion
I’ve attempted to include every popular addon for the Visual Code IDE in this article. Adding more languages, debuggers, and tools to your installation with VS Code extensions can help your development process. Developers of extensions may easily integrate into the VS Code UI and add functionality by utilizing the same APIs that VS Code utilizes, thanks to the comprehensive extensibility approach of VS Code.
A TIP FROM THE EDITOR: We have more articles on VSCode extensions: don’t miss 5 Must-Have VS Code Extensions To Boost Productivity (And Why), Top 5 VS Code Extensions For Vue Developers For 2022, and Top Visual Studio Code extensions for developers in 2022!