TypeScript for JavaScript developers
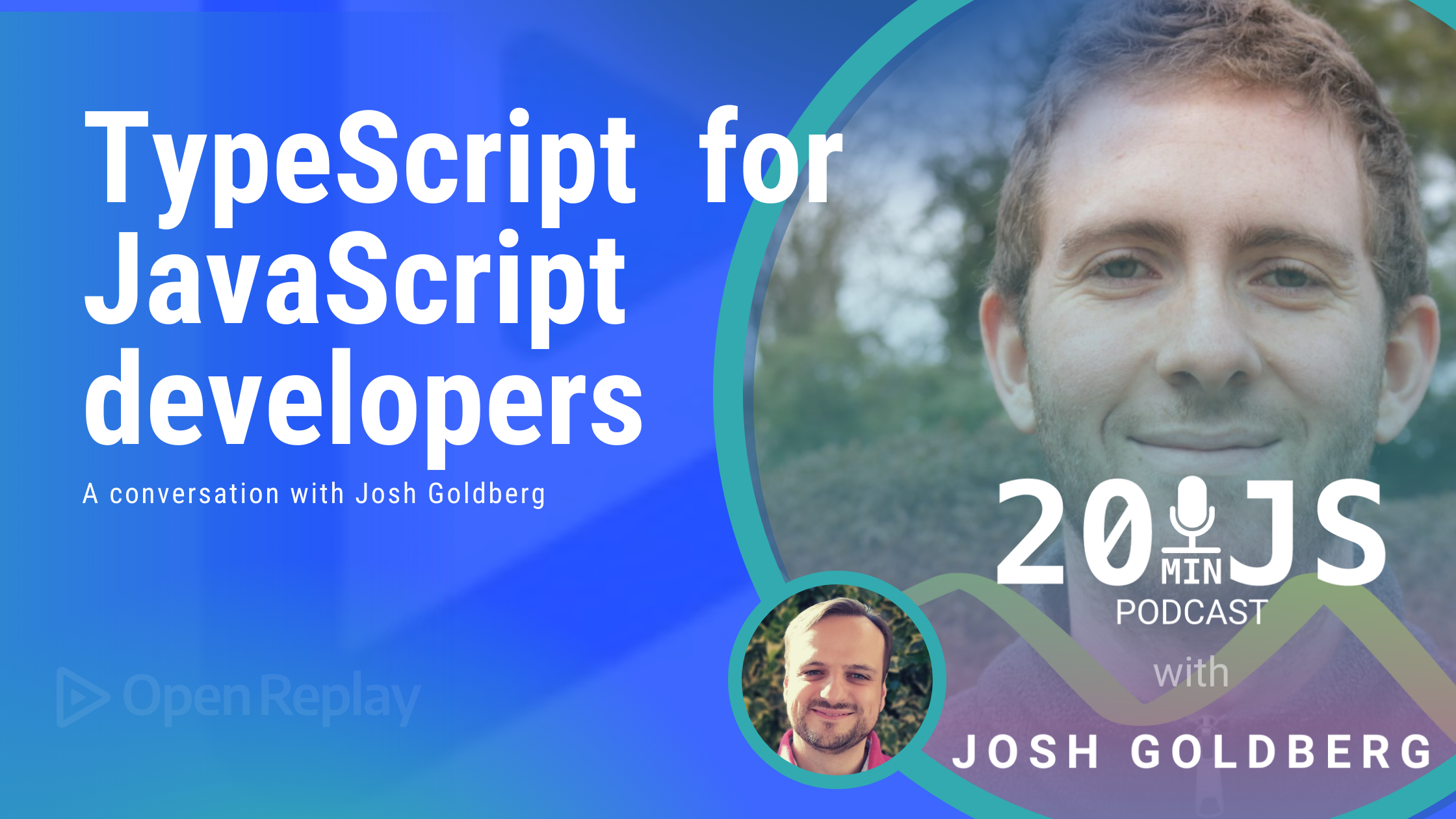
In this week’s episode of 20MinJS, we have a conversation about learning TypeScript with Josh Goldberg, author of “Learning TypeScript”. Josh has been coding in TypeScript for nearly 10 years already (can you believe the language is 10 years old!?) and he’s helped many developers make the transition. During this episode we cover questions such as:
- Should you even bother learning TypeScript if you already know JavaScript?
- Isn’t TypeScript the same as using unit tests to ensure we’re not incorrectly assigning some values?
- How difficult is it to learn TypeScript once you know JavaScript?
- What are the best resources to learn TypeScript?
You can always listen to the full episode with the player below or use your favorite podcasting app. Otherwise, enjoy the read!
Highlight #1: Is it worth learning TypeScript if you already know JavaScript?
This is a very common question because TypeScript is eventually transpiled into JavaScript for its execution. So it would only be sensible to think that whatever you can do with TS you can do as well with JS. And it is true, to a certain degree.
Whether you like it or not, many companies inside the Web Development industry are starting to adopt it. Whether that’s because of the type-checking capabilities, the
fantastic integration with popular IDEs or the OOP features, more and more companies are starting to use TypeScript as part of their tech stack.
And when that happens, you as a developer-only have two options: you stay behind and miss on potentially great job opportunities, or update yourself and make sure you’re
capable of dealing with the challenges these companies throw your way.
If you do a quick search online, you’ll find companies such as Slack, Medium, Asana, Canva, and many others stating that TypeScript is part of their stack.
So, in other words, is it worth learning TypeScript? Heck yeah!
Open Source Session Replay
OpenReplay is an open-source, session replay suite that lets you see what users do on your web app, helping you troubleshoot issues faster. OpenReplay is self-hosted for full control over your data.
Start enjoying your debugging experience - start using OpenReplay for free.
Highlight #2: What makes TypeScript so special? I already know ReScript/CoffeeScript/PureScript/etc.
Yes, there are too many JavaScript transpilers out there. Some of them are hobby projects done during a weekend and others try to give you tools that you can use in production. And don’t get me wrong, some of these transpilers are fantastic, and they do a great job at giving you an alternative to JS that can later be translated into JS to be executed.
So why go with TypeScript if there are so many other options? The answer is quite simple: age. TypeScript is, as Josh so elegantly puts it during the interview, the first one to get right the task of giving developers a higher-level language that works on top of JS. It did so 10 freaking years ago, and during all this time, it has been slowly evolving. And it’s been evolving while listening to the community that formed around it.
How can you hope to dethrone a king that’s been learning how to reign for the last 10 years? You can’t, at least not directly.
That means that if you’re looking for a way to write “better” JavaScript (whatever that means to you), then TypeScript is probably your best bet.
Highlight #3: TypeScript vs unit tests
I have to admit I’m guilty of believing this one myself, after all, you often hear and read online that TypeScript can save you from adding silly mistakes into your code when you incorrectly assign a wrong value to a variable. Like doing:
function checkLeapYear(year) {
const leap = new Date(year, 1, 29).getDate() === "29";
if (leap) {
console.log('The year', year, 'is a leap year');
} else {
console.log('The year', year, 'is not a leap year');
}
}
console.log(checkLeapYear(2000))
Can you spot the bug? It’s not a complicated function, but with a silly mistake like that, I’ve managed to break my logic. And yes, TypeScript would not let me do that
because it would know that I’m comparing a number (returned by the getDate
method) with a string.
But then again, I would also be able to catch this problem if I was writing unit tests.
So why do I have to learn TypeScript again?
The answer to that, is that TypeScript is not just type-checking, in fact, that’s a tiny part of what it does, and if you’re only hoping to use it for that, then you’re looking to underutilize a very powerful tool.
As Josh puts it, “TypeScript is not there to validate that your code works at test time that your code works. TypeScript is there to keep you from writing code that you shouldn’t have written in the first place”.
And he’s right. If you’re comparing TypeScript with a testing framework, then you haven’t understood the power behind TypeScript. The idea of using it is to write code that by definition, is more secure (at least when it comes to type safety). It’s also a fanstastic transpiler that allows you to try and work with features in JavaScript that are not present on the language. And here is another area where TS shines.
We’re not just talking about type annotations anymore, we’re talking about advanced OOP concepts such as Interfaces, Generics. We’re also talking about type unions and a lot more. It allows you to describe your code through definitions that JavaScript simply can’t provide.
And on top of that, the native integration that many IDEs have with it, like VSCode (for obvious reasons) give you extra superpowers when coding, even if you’re not directly using TypeScript!
So to answer this topic: no, TypeScript can’t be replaced by unit tests, and if you do, that means you’re not taking advantage of 80% of the language.
Highlight #4: Is ECMAScript’s type annotation proposal going to kill TypeScript?
In case you have yet to hear about it, there is a Stage 1 proposal to add type annotations to JavaScript. Sounds familiar, doesn’t it? Especially if you look at the proposed syntax:
function equals(x: number, y: number): boolean {
return x === y;
}
I mean, it’s very TypeScript-like, and that’s fine, they’re essentially trying to add into the language what the community is using. That said, we’re only dealing with type annotations here, at least as a first step. Do you think this proposal is forshadowing the end of TypeScript?
According to Josh, this is, in fact, a good thing! Yeah, he sees this as an opportunity to bring JavaScript’s syntax closer to TypeScript’s, that way
we could potentially ditch the .ts
extension in the future. At least in the cases where you’re not using any added feature in the language.
And it’ll also act as a gateway for developers to start messing around with type safety without having to make the switch into something they don’t know.
Right now, migrating a JavaScript project to TypeScript requires considerable effort and initial analysis to understand where to start and which files should be modified first. If we had this proposal already accepted, we could be considering lower migration times and less risk involved as well.
While I have to admit that adding type checking to an otherwise dynamically typed language feels strange, I can see Josh’s point. I hope that we get to play with this proposal soon enough and see what effect it has on both communities.
That’s it for the highlights of our interview. Remember that you can listen to the full version on your favorite podcasting app, or with the embedded player above.
Now let me ask you this: are you pro or against TypeScript? What do you think about it after listening (or reading) what Josh has to say about it.