Understanding React Fiber: How It Improves Rendering Performance
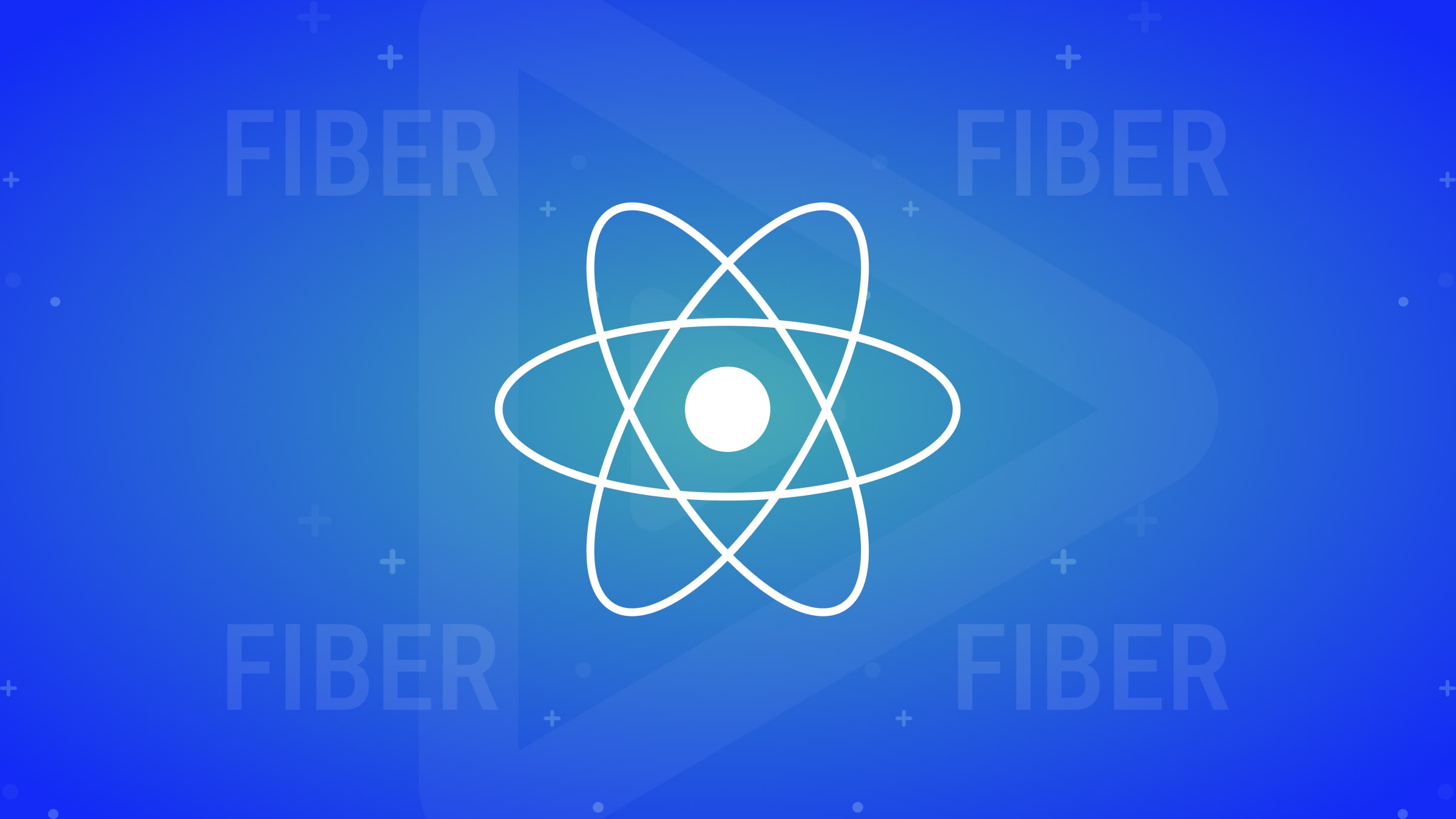
React Fiber is a core update introduced in React 16 that changes how React renders UI updates, significantly improving performance and responsiveness. Before Fiber, React would render updates synchronously, sometimes freezing the UI during large updates. Fiber fixes this by breaking rendering into small chunks, scheduling them efficiently, and allowing the browser to stay responsive.
Key Takeaways
- React Fiber splits rendering into incremental, asynchronous chunks.
- Fiber prioritizes updates, improving UI responsiveness.
- Fiber enables advanced features like Concurrent Mode and Suspense.
- No changes to your existing React code are required to benefit from Fiber.
What is React Fiber?
React Fiber is the internal reconciliation algorithm of React. It compares changes in your components and efficiently applies necessary DOM updates. Fiber replaces the older, synchronous stack reconciler with an incremental, asynchronous rendering approach.
Stack Reconciler (Old):
- Synchronous and blocking
- Updates completed in one go
- Could freeze the UI
Fiber Reconciler (New):
- Incremental and asynchronous
- Work divided into smaller units
- Can pause and resume rendering
How React Fiber Improves Rendering Performance
Fiber improves performance by:
Incremental Rendering (Time Slicing)
Fiber breaks rendering tasks into smaller units spread across multiple frames, preventing UI freezes.
Prioritization of Updates
Fiber schedules high-priority tasks, like user interactions, first. Lower-priority tasks run when the browser is idle.
Concurrency Support
Fiber lets React pause, resume, or cancel rendering tasks based on priority, keeping the UI fluid and responsive.
Key Features of React Fiber
- Improved Reconciliation: Efficiently identifies minimal necessary DOM changes.
- Scheduling and Prioritization: Manages rendering priority for smooth user experiences.
- Suspense and Concurrent Mode: Handles asynchronous operations seamlessly, preventing UI blockage.
Comparing React Fiber with the Old Reconciliation Algorithm
The older reconciler rendered all updates synchronously, often causing UI lag. Fiber avoids this by working incrementally:
- Old reconciler: One big update (potentially slow, unresponsive UI).
- Fiber reconciler: Many small, interruptible updates (smooth, responsive UI).
Example scenario:
- Old: Heavy list updates block interactions.
- Fiber: User interactions immediately interrupt list updates, making apps feel faster.
How to Optimize Your React Code with React Fiber
Follow these best practices:
-
Use Concurrent Features (React 18+):
import { useTransition } from 'react'; function FilterList({ items }) { const [filteredItems, setFilteredItems] = useState(items); const [isPending, startTransition] = useTransition(); const handleInput = (e) => { const input = e.target.value; startTransition(() => { setFilteredItems(items.filter(item => item.includes(input))); }); }; return <input onChange={handleInput} />; }
-
Prevent Unnecessary Renders: Use
React.memo
anduseMemo
to avoid redundant renders. -
Lazy Loading and Code-Splitting: Load components on-demand to speed up initial rendering:
const HeavyComponent = React.lazy(() => import('./HeavyComponent')); function App() { return ( <Suspense fallback={<div>Loading...</div>}> <HeavyComponent /> </Suspense> ); }
-
Employ Windowing for Large Lists: Use libraries like react-window to efficiently render large lists.
-
React Profiler: Use React DevTools Profiler to find and fix performance issues.
Conclusion
React Fiber significantly enhances React’s rendering capabilities, making apps faster and more responsive. Its incremental rendering, prioritization, and concurrency allow complex apps to deliver smooth, uninterrupted user experiences.
FAQs
React Fiber is React’s internal algorithm for efficiently updating the UI by rendering incrementally rather than all at once.
No. React Fiber works automatically from React 16 onwards without requiring changes in your existing code.
Fiber breaks rendering into chunks, prioritizes urgent tasks, and supports concurrent operations, keeping the UI responsive.
No. Fiber is the underlying engine enabling concurrent features like Concurrent Mode and Suspense, which are built on Fiber.
Time slicing splits large rendering tasks into smaller chunks, allowing React to pause and resume work without freezing the UI.