How to Use Axios in Node.js (With Code Examples)
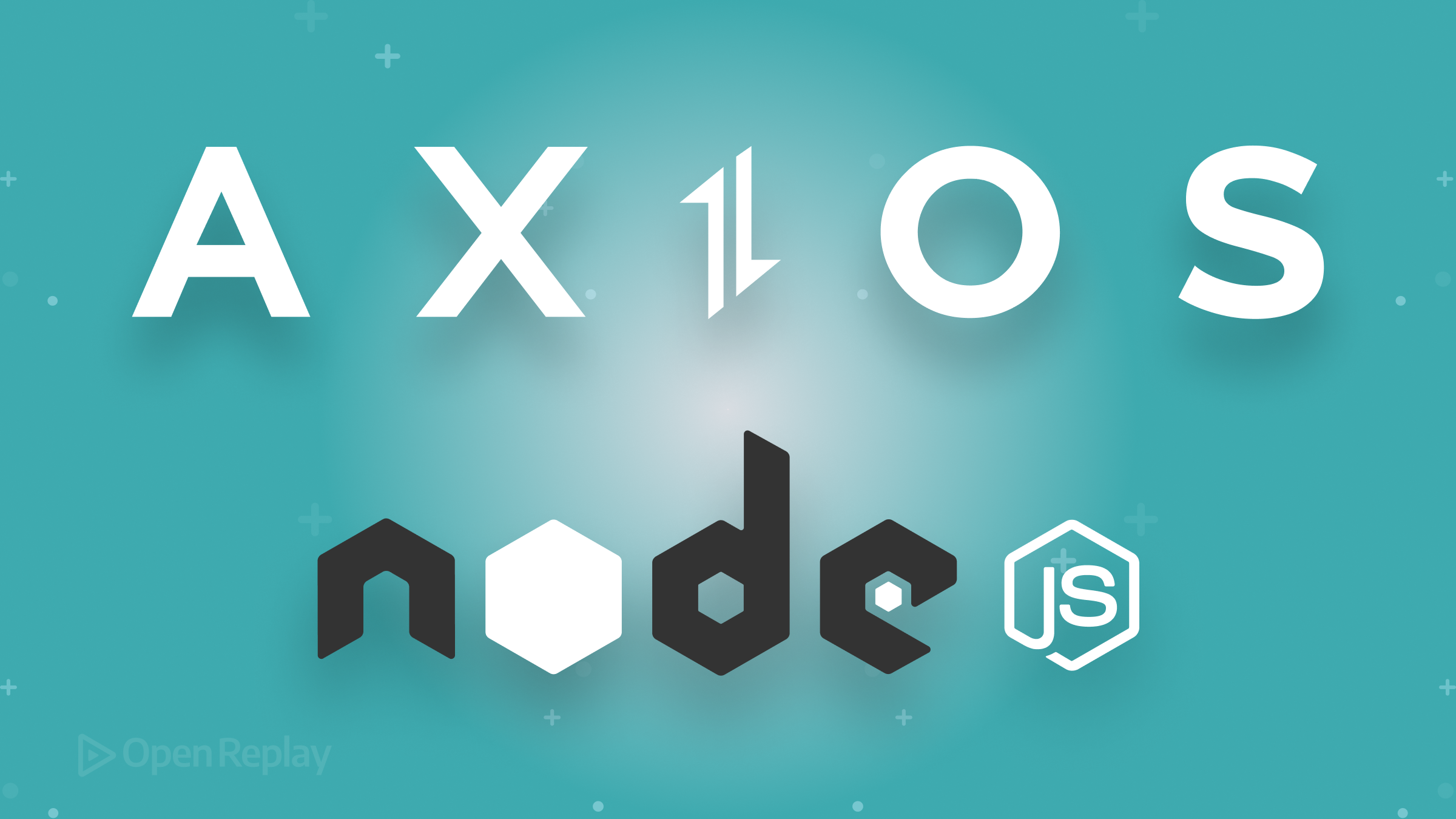
Axios is a promise-based HTTP client that simplifies making HTTP requests in Node.js. It streamlines tasks like sending GET and POST requests, handling responses, and managing errors. In this guide, we’ll explore how to use Axios in Node.js with practical code examples.
Key Takeaways
- Axios provides a simple API for making HTTP requests in Node.js.
- It utilizes promises for cleaner asynchronous code.
- Offers straightforward mechanisms to handle various error scenarios.
Setting Up Axios in Node.js
To get started with Axios in your Node.js project:
-
Initialize your project (if you haven’t already):
npm init -y
-
Install Axios:
npm install axios
-
Require Axios in your application:
const axios = require('axios');
Making GET Requests
A GET request retrieves data from a specified endpoint. Here’s how to perform a GET request using Axios:
const axios = require('axios');
async function fetchData() {
try {
const response = await axios.get('https://jsonplaceholder.typicode.com/posts/1');
console.log(response.data);
} catch (error) {
console.error('Error fetching data:', error);
}
}
fetchData();
Explanation:
- Defines an asynchronous function
fetchData
. - Uses
await
to make an Axios GET request. - Logs response data to the console.
- Handles errors using
try...catch
.
Making POST Requests
A POST request sends data to a server to create a new resource. Here’s how to perform a POST request:
const axios = require('axios');
async function createPost() {
try {
const response = await axios.post('https://jsonplaceholder.typicode.com/posts', {
title: 'New Post',
body: 'This is the content of the new post.',
userId: 1,
});
console.log('Post created:', response.data);
} catch (error) {
console.error('Error creating post:', error);
}
}
createPost();
Explanation:
- Defines an asynchronous function
createPost
. - Uses
await
withaxios.post()
, passing the endpoint and data object. - Logs the newly created post.
- Handles errors properly.
Handling Responses and Errors
Axios provides a structured way to handle responses and errors. The response object includes properties like data
, status
, and headers
.
Here’s how to handle different types of errors:
const axios = require('axios');
async function fetchData() {
try {
const response = await axios.get('https://jsonplaceholder.typicode.com/posts/1');
console.log('Data:', response.data);
console.log('Status:', response.status);
} catch (error) {
if (error.response) {
// Server responded with a status code outside 2xx
console.error('Error data:', error.response.data);
console.error('Error status:', error.response.status);
} else if (error.request) {
// No response received
console.error('No response received:', error.request);
} else {
// Error setting up the request
console.error('Error:', error.message);
}
}
}
fetchData();
Explanation:
- Logs response data and status when successful.
- Differentiates between:
- Errors with responses (
error.response
) - Errors with no response (
error.request
) - Other Axios-related errors (
error.message
)
- Errors with responses (
FAQs
Yes, Axios is isomorphic and can run in both environments.
Axios automatically transforms JSON data for both requests and responses, eliminating the need for manual parsing.
Yes, you can set custom headers by passing a `headers` object in the request configuration.
Conclusion
Axios simplifies HTTP requests in Node.js, offering a clean and concise API. Its support for promises allows for efficient handling of asynchronous operations. By understanding how to perform GET and POST requests and handle responses and errors, you can effectively integrate Axios into your Node.js applications.