Vue Development - Exploring the Composition and Options APIs
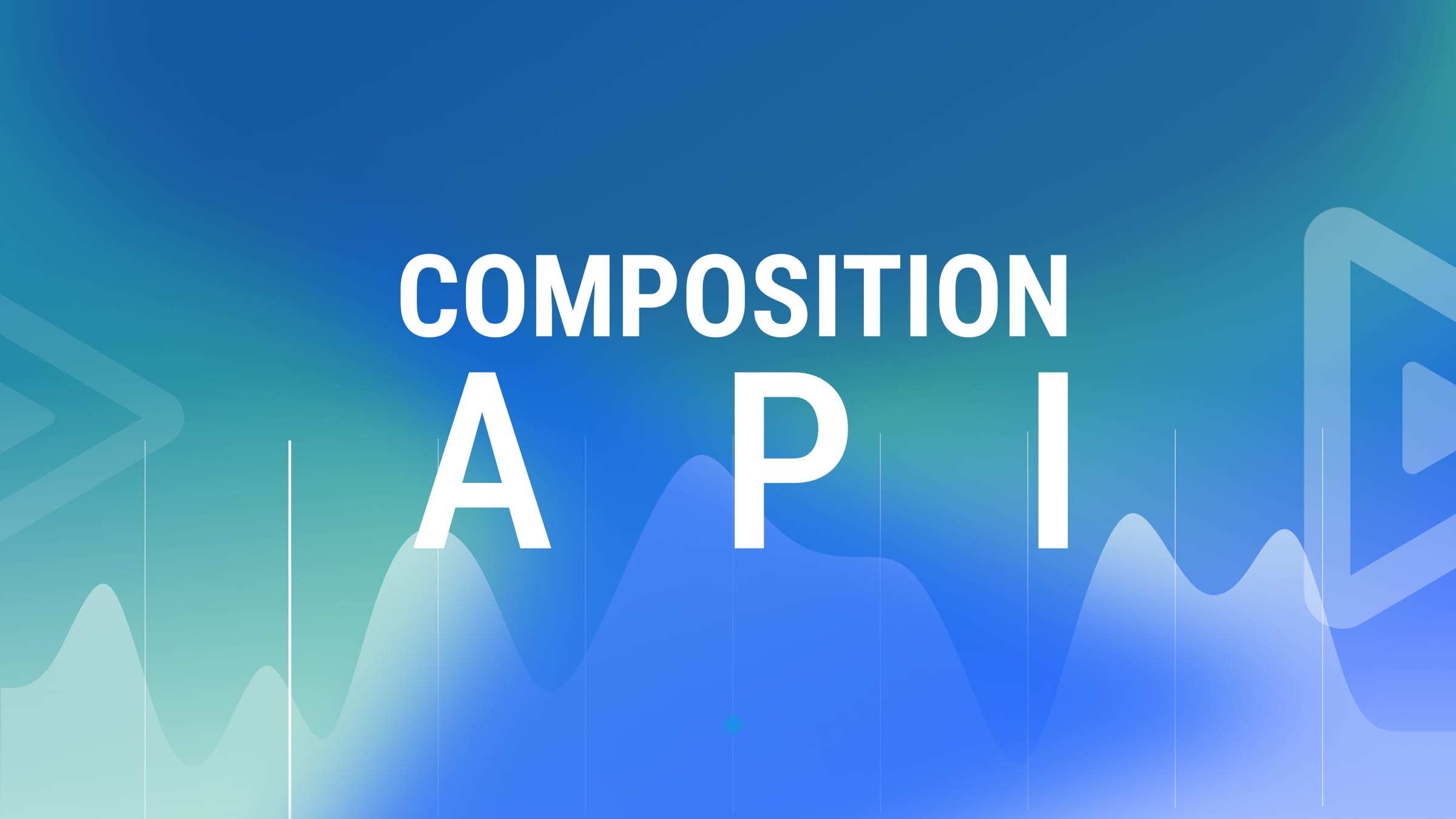
Discover how at OpenReplay.com.
Vue.js, a JavaScript framework for building user interfaces, offers two distinct approaches to crafting components: the Options API and the Composition API. The Options API has been the primary method for creating Vue components since the inception of Vue, making it a great starting point for new Vue.js developers due to its simplicity and user-friendly nature. However, as the project grows in complexity, it may lead to issues like code duplication, difficulty in organizing complex logic in components, and challenges in maintaining large codebases, which can hinder development efficiency and code maintainability.
To address these limitations, Vue 3.0 introduced the composition API. This API brings a new set of principles that allow developers to write more modular, reusable, and maintainable code. It embraces a functional programming paradigm, emphasizing modularity through composables, which are reusable functions that encapsulate component logic, state management, and other functionalities.
When choosing between the Options API and the Composition API, understand that your decision significantly affects your project’s development and maintainability. The Options API suits simpler components, while the Composition API offers a more powerful and flexible approach, allowing you to craft complex, reusable, and maintainable codebases.
Without further ado, let’s jump in and get hands-on! We’ll explore both APIs, check out some code snippets, and understand what makes them different.
Let’s go!👇
Options API
The Options API has a distinct, straight-to-the-point organization for building components, and here’s what a basic structure looks like:
export default {
data() {
return {};
},
computed: {},
methods: {},
};
In the above code snippet, data
stores variables and data properties, computed
holds derived variables, while methods
make it easy to access app functions.
Ready to see this in action by building a countdown app using the Options API?
Let’s go!
<script>
export default {
data() {
return {
targetTime: new Date("2023-12-31T23:59:59").getTime(),
currentTime: new Date().getTime(),
intervalId: null,
};
},
computed: {
timeRemaining() {
return Math.max(0, this.targetTime - this.currentTime);
},
formattedTime() {
const seconds = Math.floor(this.timeRemaining / 1000);
const minutes = Math.floor(seconds / 60);
const hours = Math.floor(minutes / 60);
const days = Math.floor(hours / 24);
return `${days}d ${hours % 24}h ${minutes % 60}m ${seconds % 60}s`;
},
},
mounted() {
this.intervalId = setInterval(this.updateTimer, 1000);
},
beforeDestroy() {
clearInterval(this.intervalId);
},
methods: {
updateTimer() {
this.currentTime = new Date().getTime();
},
},
};
</script>
In this version of the Options API, the data
function handles reactive data properties like targetTime
, currentTime
, and intervalId
, while timeRemaining
and formattedTime
are computed properties declared within the computed
option. Lifecycle hooks, such as mounted
and beforeDestroy
manage the start and stop of the interval. Additionally, the updateTimer
method in the methods
option is employed to update the currentTime
property.
As you can see, the code organization is pretty straightforward to understand because our example is simple, but it may not apply to a more complex component.
Composition API
Composition API is a better approach when dealing with a complex component, especially for reusability purposes.
Here’s what the structure looks like. 👇
export default {
setup() {
return {
};
},
};
The Composition API in Vue 3 adds a layer to the existing Options API. It comes into play within the setup()
function, essentially serving as another facet of the Options API. This setup()
function allows you to tap into Vue 3 reactivity, empowering you to create reactive variables.
Here’s how you can build a countdown timer component with composition API.
<script>
import { ref, computed, onMounted, onBeforeUnmount } from "vue";
export default {
setup() {
const targetTime = new Date("2023-12-31T23:59:59").getTime();
const currentTime = ref(new Date().getTime());
const timeRemaining = ref(targetTime - currentTime.value);
const formattedTime = computed(() => {
const seconds = Math.floor(timeRemaining.value / 1000);
const minutes = Math.floor(seconds / 60);
const hours = Math.floor(minutes / 60);
const days = Math.floor(hours / 24);
return `${days}d ${hours % 24}h ${minutes % 60}m ${seconds % 60}s`;
});
let intervalId;
onMounted(() => {
intervalId = setInterval(updateTimer, 1000);
});
onBeforeUnmount(() => {
clearInterval(intervalId);
});
function updateTimer() {
currentTime.value = new Date().getTime();
timeRemaining.value = Math.max(0, targetTime - currentTime.value);
}
return {
formattedTime,
};
},
};
</script>
Let’s break down the functionality of each part of the code 👇
In this code, the ref
function ensures that targetTime
, currentTime
, and timeRemaining
respond to changes, and the formattedTime
property displays time neatly, relying on timeRemaining
for calculations and formatting.
Meanwhile, onMounted
starts the timer (setInterval
) when the component is on the screen, and onBeforeUnmount
stops the timer before it gets removed, preventing memory issues. The updateTimer
function manages updates, quietly handling tasks like updating currentTime
and smoothly managing timeRemaining
.
Finally, the formattedTime
computed property returns from the setup function, making it accessible in the code.
As you can see, the Composition API provides a more flexible and organized way to manage component logic, especially when dealing with reactivity and lifecycle management. This API introduces new lifecycle functions, such as onMounted
and onBeforeUnmount
, and builds upon the foundation of the Options API.
Migrating to Composition
Transitioning to the Composition API offers a more flexible and scalable way to structure components, which provides several advantages, including improved code organization, better reusability of logic, and enhanced readability.
It becomes particularly beneficial as your Vue projects grow, emphasizing the importance of understanding the reactivity system, which is fundamental to the Composition API.
Composables, reactive, and ref
are key terms you will encounter frequently.
Additionally, Identifying areas within your components where logic can benefit from encapsulation and reusability is crucial.
Furthermore, leveraging your knowledge of Vue core concepts from the Options API will facilitate the transition. The Composition API builds upon these ideas, providing extra features and a more modular structure.
Conclusion
Choosing between Options API and Composition API can pose a challenge, especially for new Vue developers. However, it is crucial to understand the nuances and how they can impact your development experience.
In my early days with Vue, I found the Options API intuitive and easy to grasp. However, as I delved deeper, I discovered the Composition API’s widespread use and numerous benefits.
While Options API has long been foundational, Composition API emerges as a powerful alternative. It provides enhanced modularity, reusability, and maintainability for complex projects. The decision depends on project requirements and preferences. But, embracing the Composition API aligns with current trends, scalability, and future-oriented programming concepts.
Additional Resources
For more information on the Composition and Options APIs, you can visit the Vue.js documentation to learn more about them:
Until next time, ciao!!!
Gain Debugging Superpowers
Unleash the power of session replay to reproduce bugs, track slowdowns and uncover frustrations in your app. Get complete visibility into your frontend with OpenReplay — the most advanced open-source session replay tool for developers. Check our GitHub repo and join the thousands of developers in our community.