What Does `//` Mean in Python? (With Examples)
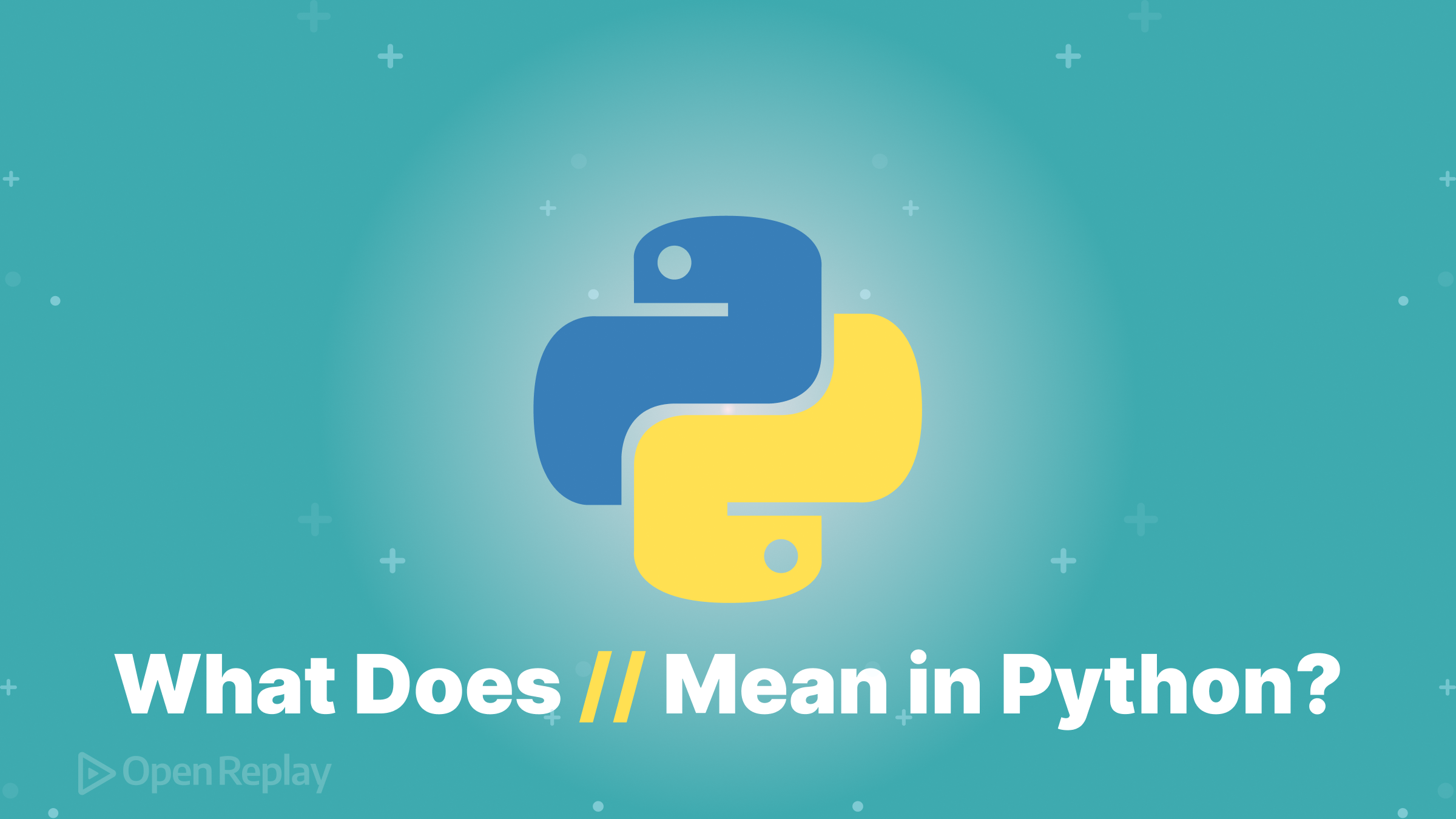
In Python, //
is the floor division operator. It divides two numbers and rounds the result down to the nearest whole number.
For example:
print(10 // 3) # Output: 3
print(-10 // 3) # Output: -4
Key Takeaways
//
performs floor division, meaning it rounds down the result.- Works with integers and floating-point numbers.
- Always rounds towards negative infinity (
-∞
).
Understanding Floor Division (//
)
The //
operator divides two numbers but instead of returning a floating-point result, it truncates (rounds down) to the nearest integer.
Example:
print(7 // 2) # Output: 3 (instead of 3.5)
print(-7 // 2) # Output: -4 (rounds towards -∞)
How Does //
Handle Floats?
Even if one of the operands is a float, the result remains floored but in float format:
print(7.0 // 2) # Output: 3.0
print(-7.5 // 2) # Output: -4.0
Difference Between /
and //
Operator Description Example /
Normal division (returns float) 5 / 2 = 2.5
//
Floor division (rounds down) 5 // 2 = 2
When to Use //
?
- When you only need whole numbers (e.g., counting items).
- Avoiding float precision errors (e.g., indexing arrays).
- Ensuring integer results in loops.
Example: Using //
for pagination:
items_per_page = 10
total_items = 95
pages = total_items // items_per_page
print(pages) # Output: 9
FAQs
No, if one of the operands is a float, the result is a float.
Because `//` rounds towards negative infinity (`-∞`).
Yes, `a // b` is equivalent to `math.floor(a / b)`.
Conclusion
The //
operator in Python performs floor division, rounding the result down to the nearest whole number. It’s useful when working with integer math, loops, and pagination.