Working with Dates and Times with Day.js
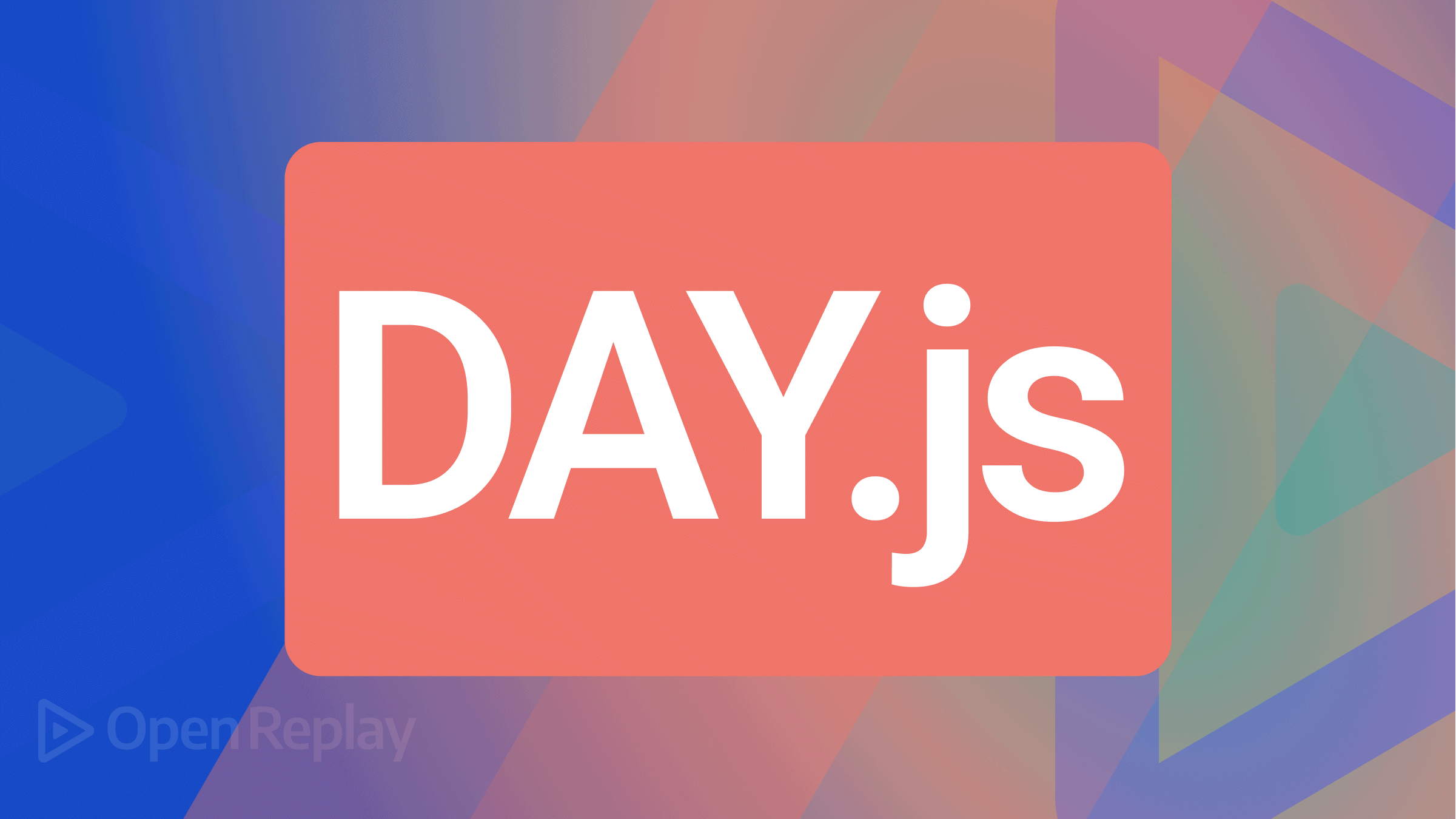
Day.js is a straightforward JavaScript tool for manipulating, displaying, and validating dates and times in modern browsers.
Day.js appears to be a very capable and user-friendly library with a wide range of customization and extension possibilities.
Day.js merely serves as a substitute for Moment.js. The Moment.js team has announced that the project is now a heritage one and is being maintained.
Day.js is one of the most recommended libraries for formatting date and time in JavaScript since it can be used in client-side and server-side rendering and works flawlessly in both scenarios.
Below is a graph showing the monthly NPM downloads of Day.js.
Below is a graph showing the Day.js stars that have been added to GitHub.
In this article, we’ll show how to use it to possibly replace Moment.js.
Why replace Moment.js?
Moment.js is a JavaScript package that makes it very simple to parse, validate, manipulate, and display dates and times in JavaScript.
Since the Moment.js team issued a deprecation notice regarding its use, developers have been forced to migrate to Day.js and other suggested libraries. The disadvantages of Moment.js are listed below.
- Moment.js doesn’t work well with tree-shaking because it causes a large bundle size and performance problems.
- Moment.js has a problem that affects mutability.
- However, because of its complicated APIs and large bundle size, it could result in a significant performance penalty if you are working on a web application that requires high performance.
Benefits of Day.js
- With a comparable API, Day.js is intended to be a simple substitute for Moment.js. Day.js is not a drop-in replacement; however, if you are accustomed to working with Moment’s API and need to move swiftly, consider this.
- With Day.js performance at a high rate.
- Day.js is lighter than Moment.js since the package size of Date.js is only about 232 kB. Day JS’s most recent version is 7Kb (minified) and 2Kb in size (Gzipped).
- Day.js can be included as a JavaScript file from a CDN or local file in addition to supporting import and require.
There’s one drawback, however:
- The features of Day.js are fewer than those of Moment.js.
Day.js Support in JavaScript Community.
The need for ongoing tool updates is what triggers this problem for developers. Any web developer will always aim for maximum optimization to produce simple, quick apps. To guarantee the high performance of software products and to maintain their security, we must regularly upgrade our libraries and frameworks to the most recent versions.
Day.js is an excellent substitute for Moment.js when comparing size and performance.
Getting Started
Finding a CDN for the Day.js is simple, cdnjs.com provides a Day.js CDN to be used on our browser The CDN link has a script tag that may be added at the top or bottom of our HTML code.
<script src="https://cdnjs.cloudflare.com/ajax/libs/dayjs/1.11.5/dayjs.min.js" integrity="sha512-Ot7ArUEhJDU0cwoBNNnWe487kjL5wAOsIYig8llY/l0P2TUFwgsAHVmrZMHsT8NGo+HwkjTJsNErS6QqIkBxDw==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
Using Unpkg:
<script src="https://unpkg.com/dayjs@1.8.21/dayjs.min.js"></script>
To install day in node js, run the following code below.
npm i dayjs
Use the code below to include Day.js as a requirement in a Node.js project:
const dayjs = require("dayjs");
If you are using Babel or ES6:
import Dayjs from "dayjs";
In this tutorial, we’ll format Date and Time using Day.js CDN and plain JavaScript in the browser.
Overview of ISO
A standard method of expressing a numeric calendar date that removes ambiguity is the International Organization for Standardization (ISO) date format. You can use the JavaScript date method, toISOString(), which returns the freshly formed date in ISO format, to confirm this.
new Date().toISOString()
//Output: 2022-08-17T08:13:13.358Z
To learn more about JavaScript dates, kindly visit this link.
Formatting dates
Get the formatted date using the token string that was supplied. Keep in mind that the current date is in ISO format.
When we run Day.js in the browser, the .format() method returns a string in ISO format. This is fairly similar to the vanilla JavaScript’s toISOString() date constructor method.
console.log(dayjs().format())
//Output: 2022-08-17T09:28:20+01:00
Put escape characters inside square brackets (e.g. [MM, DD, YY]):
dayjs().format() // Output: 2022-08-17T09:31:09+01:00
dayjs().format('MM') // Output: 08
dayjs().format('DD') // Output: 17
dayjs().format('YY') // Output: 22
dayjs().format('MMMM') // August
Additionally, you can define the format in which you want the date returned on
console.log(dayjs('2022-04-2').format('DD/MM/YYYY')) // Output: 02/04/2022
The code below will produce an error since it used the wrong date format:
console.log(dayjs('04-2022-2').format('YYYY/MM/DD')) // Output: Invalid Date
The code below will return the date in the desired format, so let’s test it out. Always remember that a plugin is necessary if you want additional Day.js functionality.
dayjs().format('a') // am
dayjs().format('A') // AM
// @ The offset from UTC, ±HHmm
dayjs().format('ZZ') // +0100
// @ The millisecond, 3-digits
dayjs().format('SSS') // 912
dayjs().format('h:mm A ') // 8:28 AM
dayjs().format('h:mm:ss A') // 8:30:51 AM
dayjs().format('MM/DD/YYYY') // 08/19/2022
dayjs().format('M/D/YYYY') // 8/19/2022
dayjs().format('ddd, hA')
// Output: "Fri, 8AM"
dayjs().format('MMM D, YYYY')
// Aug 19, 2022
We can also see some advanced date formatting:
dayjs().format('ddd, MMM D, YYYY h:mm A ');
// @ Output: Fri, Aug 19, 2022 8:23 AM
dayjs().format('MMM D, YYYY h:mm A');
// Aug 19, 2022 8:24 AM
dayjs().format('dddd, MMMM D YYYY, h:mm:ss A')
// Output: "Friday, August 19 2022, 8:15:51 AM"
dayjs().format('dddd, MMMM Do YYYY, h:mm:ss A')
// output => "Friday, August 19o 2022, 8:15:51 AM"
The RelativeTime
Plugin
Before moving on to other examples, let’s discuss the RelativeTime plugin. Using the RelativeTime plugin, you can convert a date and time number into a relative statement, such as “5 hours ago.” Simple, right?
Browser Setup with CD: We must employ a Relativetime CDN and then set it up with Day.js for the RelativeTime plugin to function.
- Make sure the Day.js CDN is included at the top
<script src="https://cdnjs.cloudflare.com/ajax/libs/dayjs/1.11.5/dayjs.min.js"
integrity="sha512-Ot7ArUEhJDU0cwoBNNnWe487kjL5wAOsIYig8llY/l0P2TUFwgsAHVmrZMHsT8NGo+HwkjTJsNErS6QqIkBxDw=="
crossorigin="anonymous" referrerpolicy="no-referrer"></script>
- Second, you must use the RelativeTime Plugin with a CDN that you may obtain from cdnjs.com.
<script src="https://cdnjs.cloudflare.com/ajax/libs/dayjs/1.11.5/plugin/relativeTime.min.js"></script>
- The RelativeTime Plugin is being set up for Day. js
<script>
dayjs.extend(window.dayjs_plugin_relativeTime)
</script>
RelativeTime Plugin for Node.js Require and ES6 Import
The RelativeTime plugin is located inside the Day.js packages when using npm i dayjs
. You only need the route to RelativeTime inside of Day to use it.
- Nodejs require:
const dayjs = require('dayjs')
var relativeTime = require('dayjs/plugin/relativeTime')
dayjs.extend(relativeTime)
- ES6 import
import Dayjs from "dayjs";
import relativeTIme from "dayjs/plugin/relativeTime";
Dayjs.extend(relativeTIme);
Open Source Session Replay
OpenReplay is an open-source, session replay suite that lets you see what users do on your web app, helping you troubleshoot issues faster. OpenReplay is self-hosted for full control over your data.
Start enjoying your debugging experience - start using OpenReplay for free.
Getting Time from X
This gives the string representing relative time from X. The relative time plugin will then enable us to accomplish this.
Always ensure that the relevant plugin is configured using the dayjs.extend. You can read more about Day.js plugin
dayjs.extend(window.dayjs_plugin_relativeTime);
var a = dayjs("2022-01-01");
console.log(dayjs("2020-01-01").from(a))
// Output: 2 years ago
You can obtain the value without the suffix if you pass true.
dayjs.extend(window.dayjs_plugin_relativeTime);
var a = dayjs("2022-01-01");
console.log(dayjs("2020-01-01").from(a, true))
// Output: 2 years
Getting Time from now
This turns the string of relative time from now. Now a RelativeTime Plugin is required.
<script>
dayjs.extend(window.dayjs_plugin_relativeTime);
console.log(dayjs('2000-01-01').fromNow())
</script>
// Output: 23 years ago
From the future:
<script>
dayjs.extend(window.dayjs_plugin_relativeTime);
console.log(dayjs('2050-01-01').fromNow())
</script>
// Output: in 27 years
Without a suffix: You can supply the return date string with a boolean value of true.
dayjs.extend(window.dayjs_plugin_relativeTime);
dayjs('2000-01-01').fromNow(true) // Output: 23 years
Getting Time to Now
This gives back a string representing a RelativeTime to the present. Keep in mind that this depends on the RelativeTime plugin.
dayjs.extend(window.dayjs_plugin_relativeTime);
dayjs('1980-01-01').toNow() // Output: in 43 years
Absent the suffix:
dayjs('1980-01-01').toNow(true) // Output: 43 years
How to Generate a Date’s Unix Timestamp
This gives the Day.js object’s Unix timestamp, or the number of seconds since the Unix Epoch. The Unix timestamp object is a built-in object in Day js, therefore using it doesn’t call for a plugin.
Without milliseconds:
dayjs('2019-01-25').unix() // Output: 1548370800
With milliseconds:
dayjs('2019-01-25').valueOf() // Output: 1548370800000
Use of the Unix Timestamp is always recommended, according to the Day.js documentation.
Counting the Days in a Month
Get the duration of the current month in days. There is no plugin needed.
dayjs('2020-02-04').daysInMonth() // Output: 29
Returning Date as Object
To return the date in an object format, you should employ a toObject plugin with a CDN or require it in node.js or ES6 import.
CDN:
<script src="https://cdnjs.cloudflare.com/ajax/libs/dayjs/1.11.5/plugin/toObject.min.js"
integrity="sha512-qWOc7v2jfO5Zg34fVOIfnpvDopsqDBilo8Onabl/MHIr5idHpg73tVRUGDMVOQs2dUEsmayiagk75Ihjn6yanA=="
crossorigin="anonymous" referrerpolicy="no-referrer"></script>
Node.js
var dayjs = require('dayjs')
var toObject = require('dayjs/plugin/toObject')
dayjs.extend(toObject)
Extending a CDN with toObject()
<script>
dayjs.extend(window.dayjs_plugin_toObject);
dayjs('2020-01-25').toObject()
</script>
Output:
{date: 25, hours: 0, milliseconds: 0, minutes: 0, months: 0, seconds: 0, years: 2020}
Returning a Date as an Array
To return the date in an array format, you should employ a ToArray plugin with a CDN or require it in node.js or ES6 import.
CDN:
<script src="https://cdnjs.cloudflare.com/ajax/libs/dayjs/1.11.5/plugin/toArray.min.js" integrity="sha512-OMkxIxjYZNprkgSmg2ljMvDM2JhqrDxGI6XPwApLvTyS7MLi3uBWayWxt5RzmRSlxwYlfMUgC3Gd4Fycs3HNpw==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
Node.js
var toArray = require('dayjs/plugin/toArray')
dayjs.extend(toArray)
Code example with a CDN:
```javascript
dayjs.extend(window.dayjs_plugin_toArray);
dayjs('2022-08-04').toArray() // Output: [2022, 7, 4, 0, 0, 0, 0]
Obtaining the Time and Date as JSON
For serialization as an ISO 8601 in a string. No plugin is necessary.
dayjs('2019-06-25').toJSON() // Output: 2019-06-24T23:00:00.000Z
dayjs('1996-01-11').toJSON() // Output: 1996-01-10T23:00:00.000Z
dayjs('2025-05-10').toJSON() // Output: 2025-05-09T23:00:00.000Z
Provide the Date and Time as a String.
Returns a string containing the date’s representation. No Plugin is required.
dayjs('2025-03-20').toString() // Output: Wed, 19 Mar 2025 23:00:00 GMT
dayjs('2010-08-08').toString() // Output: Sat, 07 Aug 2010 23:00:00 GMT
dayjs('01-2005-25').toString() // @ Error output: Invalid Date
Parsing Dates
The Day.js object is immutable, which means that all API operations that modify it in any manner will produce a new instance of the object.
String to Date: Examine the following code to parse a string and return it in a date format.
dayjs('2020-08-04T15:00:00.000Z')
An existing native JavaScript Date object can be used to create a Day.js object.
let d = new Date(2021, 02, 11);
let day = dayjs(); // The date returned by the first formatted date is copied in this line
Now with Parse: See the code below when using Parse to return the current date.
new Date(2021, 02, 11);
// Alternative
dayjs(new Date());
Validation
To check if the date and time are valid, use the .isValid()
method in Day.js. which yields a boolean outcome,
dayjs('1996-05-01').isValid(); // Output: true
dayjs('dummy text').isValid(); // Output: false
dayjs('2005-06-09').isValid(); // Output: true
Time Zone
Day.js offers Time Zone compatibility for areas where the same standard time is observed. It takes a plugin to use a Day.js Time Zone. To use Timezone in Day.js, we need both the Time Zone and UTC plugins.
Node.js:
const dayjs = require('dayjs')
const utc = require('dayjs/plugin/utc')
const timezone = require('dayjs/plugin/timezone') // dependent on utc plugin
dayjs.extend(utc)
dayjs.extend(timezone)
CDN for UTC Plugin
<script src="https://cdnjs.cloudflare.com/ajax/libs/dayjs/1.11.5/plugin/utc.min.js"
integrity="sha512-z84O912dDT9nKqvpBnl1tri5IN0j/OEgMzLN1GlkpKLMscs5ZHVu+G2CYtA6dkS0YnOGi3cODt3BOPnYc8Agjg=="
crossorigin="anonymous" referrerpolicy="no-referrer"></script>
CDN for Time Zone Plugin
<script src="https://cdnjs.cloudflare.com/ajax/libs/dayjs/1.11.5/plugin/timezone.min.js"
integrity="sha512-fG1tT/Wn/ZOyH6/Djm8HQBuqvztPQdK/vBgNFLx6DQVt3yYYDPN3bXnGZT4z4kAnURzGQwAnM3CspmhLJAD/5Q=="
crossorigin="anonymous" referrerpolicy="no-referrer"></script>
Day.js Extend for Time Zone and UTC Plugin:
<script>
dayjs.extend(window.dayjs_plugin_utc)
dayjs.extend(window.dayjs_plugin_timezone);
<script>
Code sample:
dayjs.tz("2020-06-01 12:00", "America/Toronto")
Output on the console:
let time = {
$D: 18,
$H: 11,
$L: "en",
$M: 10,
$W: 1,
$d: "Mon Nov 18 2013 11:55:00 GMT+0100 (West Africa Standard Time) {}",
$m: 55,
$ms: 0,
$offset: -300,
$s: 0,
$x: {
$localOffset: -60,
$timezone: "America/Toronto"
}
}
Hold on; we’re not done yet. You can use the .toString()
method included with Day.js to return the Time Zone as a plain string.
dayjs.tz("2013-11-18 11:55", "America/Toronto").toString()
// Output: Mon, 18 Nov 2013 16:55:00 GMT
Zone Parsing
Use a plugin called CurrentParseFormate to assist you in parsing Time Zone if you want to parse dates in Time Zone format.
Node.js
var customParseFormat = require('dayjs/plugin/customParseFormat')
dayjs.extend(customParseFormat)
dayjs('05/02/69 1:02:03 PM -05:00', 'MM/DD/YY H:mm:ss A Z')
// Returns an instance containing '1969-05-02T18:02:03.000Z'
CDN
<script src="https://cdnjs.cloudflare.com/ajax/libs/dayjs/1.11.5/plugin/customParseFormat.min.js" integrity="sha512-FM59hRKwY7JfAluyciYEi3QahhG/wPBo6Yjv6SaPsh061nFDVSukJlpN+4Ow5zgNyuDKkP3deru35PHOEncwsw==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
dayjs.extend(window.dayjs_plugin_customParseFormat);
dayjs.tz("12-25-1995", "MM-DD-YYYY", "America/Toronto").toString()
//Mon, 25 Dec 1995 05:00:00 GMT
Converting to Zone
Update the offset, switch the Time Zone, and then return to a day.js object instance. When a second parameter is passed with a true value, only the Time Zone (and offset) are changed while the local time remains constant.
<script>
dayjs.extend(window.dayjs_plugin_utc)
dayjs.extend(window.dayjs_plugin_timezone);
<script>
Estimating user zone
This predicts the user’s Time Zone automatically.
dayjs.extend(window.dayjs_plugin_utc)
dayjs.extend(window.dayjs_plugin_timezone);
dayjs.tz.guess() //Asia/Calcutta
Default Time Zone setting
Set your preferred time zone as the default one instead of the local one. In a specific dayjs object, a different Time Zone can still be customized.
Node.js
var utc = require('dayjs/plugin/utc')
var timezone = require('dayjs/plugin/timezone') // dependent on utc plugin
dayjs.extend(utc)
dayjs.extend(timezone)
CDN
dayjs.extend(window.dayjs_plugin_utc)
dayjs.extend(window.dayjs_plugin_timezone);
dayjs.tz.setDefault("America/New_York") // Setting the default time
dayjs.tz("2019-08-05 11:00")
Conclusion
We began by gaining a grasp of Day.js and the benefits of using it to format times and dates. Day.js simply replaces Moment.js. Even though Moment.js is not required, Day.js offers all date formatting, parsing, plugins, and localization requirements. You can therefore use dayjs.org to choose and find further information on the official Day.js website.
A TIP FROM THE EDITOR: For a possible future alternative for handling dates and times, read our Is It Time for the JavaScript Temporal API? article.