Working with the DOM in JavaScript
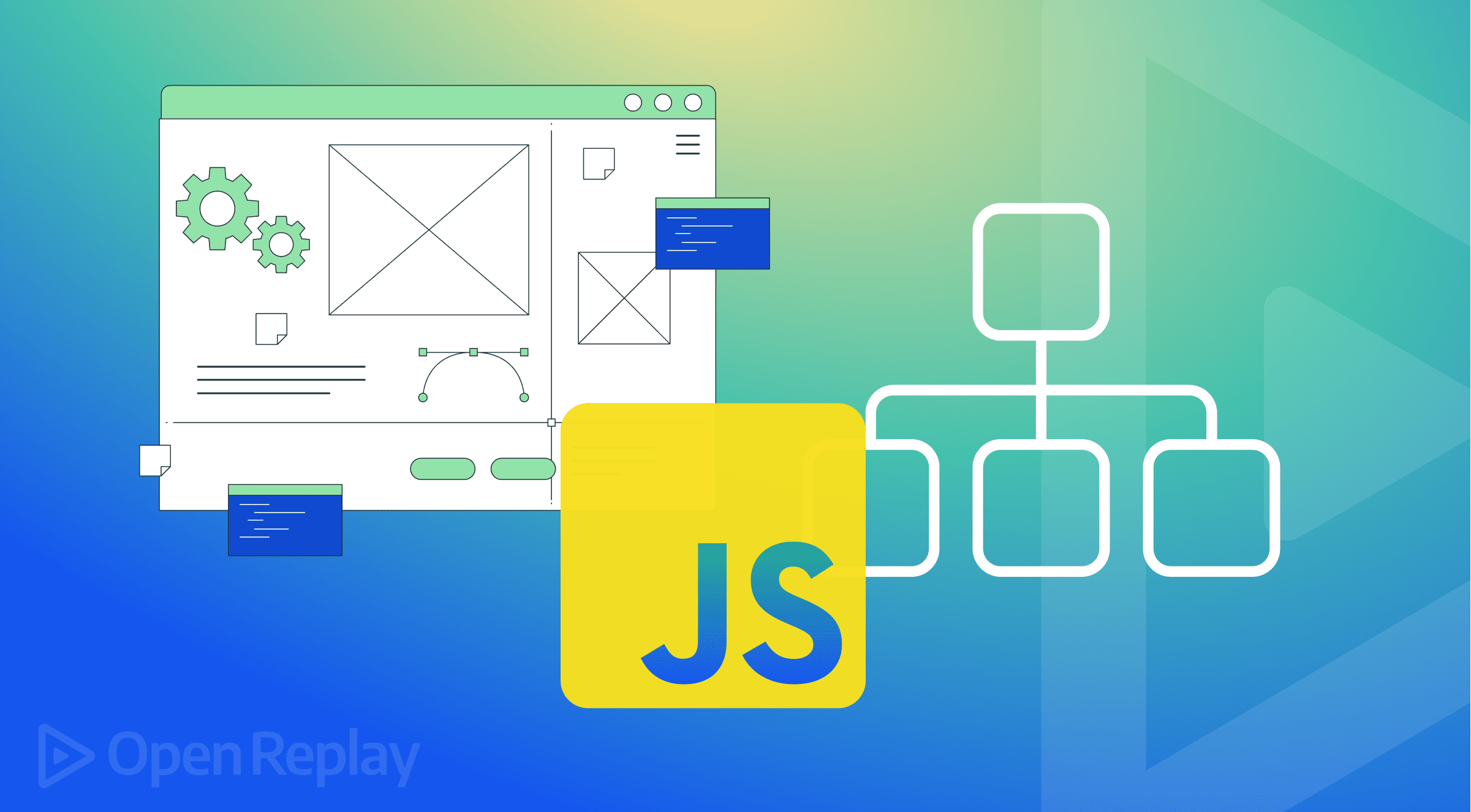
The JavaScript DOM (Document Object Model) is an interface that enables developers to manipulate a website’s content, structure, and style. In this tutorial, we will learn what the DOM is, how to create it and how it can be modified using JavaScript. Generally, a website consists of an HTML and CSS document, and the browser makes up a representation of the document, known as the Document Object Model (DOM). This document allows JavaScript to manipulate and work with the elements and styles of a website. The model is mostly built using a tree structure of objects and defines:
- HTML elements as objects
- Properties of these HTML elements and,
- Methods to access the same elements.
The JavaScript DOM Structure
The places of the elements are called nodes, and attributes and text also get their own nodes.
Features of the DOM
It is important to note that with the DOM, JavaScript can access and change all the elements of an HTML document. Hence, the following are the features of the DOM:
- JavaScript can change all the HTML attributes on the page.
- JavaScript can change all the HTML elements on the page.
- JavaScript can change all the CSS styles on the website.
- JavaScript can remove and manipulate existing HTML elements and attributes.
- JavaScript can also add new HTML elements and attributes.
- JavaScript can react to all existing HTML events on the page.
- JavaScript can also create new HTML events on the page.
Below is a table that specifies the DOM methods and their descriptions.
JavaScript DOM Methods | Description |
---|---|
addEventListener() | The “addEventListener()” method is used for attaching an event handler to a particular element |
appendChild() | The “appendChild()” method is used to add a new child node to an element |
blur() | The “blur()” method is used to remove focus from an HTML element. |
click() | The “click()” method puts on a mouse click on an HTML element. |
cloneNode() | The “cloneNode()” method is used to clone an element |
closest() | The “closest()” method is used in searching through the DOM tree for the closest Element, which matches with a specified CSS selector. |
compareDocumentPosition() | The “compareDocumentPosition()” method is used for comparing the document position of two HTML elements |
exitFullscreen() | The “exitFullscreen()” property cancels an element in fullscreen mode. |
focus() | The “focus()” method is used for assigning focus to an HTML element. |
getAttribute() | The “getAttribute()” method displays the attribute value of an element. |
getAttributeNode() | The “getAttributeNode()” method displays the specified attribute node. |
getBoundingNode() | The “getBoundingClientRect()” method outputs the position and size of an element that is related to the viewport. |
getElementsByClassName() | The “getElementByClassName()” method displays the list of child elements of a particular class. |
getElementsByTagName() | The “getElementsByTagName()” method displays the list of child elements of a specific tag. |
hasAttribute() | The “hasAttribute()” method outputs true if an element has the added attribute; otherwise, it returns false. |
hasChildNodes() | The “hasChildNodes()” method outputs true if a particular element has any child nodes; otherwise, it returns false. |
insertAdjacentElement() | The “insertAdjacentElement()” method is used for inserting an HTML element in a particular position related to the active Element. |
insertAdjacentHTML() | The “insertAdjacentHTML()” method is used for inserting an HTML formatted text in a particular position that is related to the active Element. |
insertAdjacentText() | The “insertAdjacentText()” method is used for inserting text in a particular position that is related to the active Element. |
insertBefore() | The “insertBefore()” method is used to insert a new child node before an existing child node. |
isDefaultNamespace() | The “isDefaultNamespace()” method outputs true if a particular namespaceURI is set as default; otherwise, it returns false. |
isEqualNode() | The “isEqualNode()” method is used for checking if two HTML elements are equal or not. |
isSameNode() | The “isSameNode()” method is used for verifying if two HTML elements are the same nodes or not. |
isSupported() | The “isSupported()” method outputs true if a particular feature is supported for the HTML element. |
matches() | The “matches()” method outputs a Boolean value indicating whether a specific CSS selector matches an element or not. |
normalize() | The “normalize()” method is used for joining adjacent text nodes and for eliminating empty text nodes from an HTML element. |
querySelector() | The “querySelector()” method is used to output the first child element that gets matched with a particular CSS selector of an HTML element. |
querySelectorAll() | The “querySelectorAll()” method returns all child elements that match a specified CSS selector of an element. |
remove() | The “remove()” method is used for removing a particular HTML element from the DOM. . |
removeAttribute() | The “removeAttribute()” method is used for removing a particular attribute from an HTML element |
removeAttributeNode() | The “removeAttributeNode()” method eliminates the added attribute node and shows it as an output. |
removeChild() | The “removeChild()” method is used for removing a particular child node from an HTML element. |
removeEventListener | The “removeEventListener()” method is used for removing an attached event handler. |
replaceChild() | The “replaceChild()” method is used for replacing a child node from an HTML element. |
requestFullscreen() | The “requestFullscreen()” method is used for showing the HTML element in fullscreen mode. |
scrollIntoView() | The “scrollIntoView()” method is used for scrolling through a particular HTML element and into the browser’s visible area. |
setAttribute() | The “setAttribute()” method is used for setting attributes to the added value. |
toString() | The “toString()” method is used for converting an element to a string. |
How to Create DOM in JavaScript
In this part, we will create the DOM in JavaScript and attach it to the DOM tree.
- First, we use the
createElement()
method.
const element = document.createElement(htmlTag);
- In our example, we will create a
<div>
element.
const e = document.createElement('div');
- We will fill the
<div>
with HTML content.
e.innerHTML = 'JavaScript DOM';
- Then, we attach the
<div>
element to the DOM tree by using theappendChild()
method:
document.body.appendChild(e);
- We can now use the DOM methods to create text nodes and append the text nodes to the new Element:
var textnode = document.createTextNode('JavaScript DOM');
e.appendChild(textnode);
- After that, we can now use the
appendChild()
method to attach the new Element to the DOM tree.
Fundamental Data Types and how they affect the DOM
The following table briefly describes the essential data types while creating the DOM in JavaScript.
Data type (Interfaces) | Description |
---|---|
Document | When an object of type document is returned, this object becomes the root document object itself. |
Node | Every object located within a document is a node. In an HTML document, an object can be an element node, a text node, or even an attribute node. |
Element | The element type is based on the node. This refers to an element returned by a member of the DOM API. For example, instead of saying that the document.createElement() method returns an object reference to a node, we can say that this method returns the Element that has just been created in the DOM. |
NodeList | A nodeList is an array of elements. Items in a nodeList are accessed by index in these two ways: list.item(1) or list[1] . In the first, item() is the single method on the nodeList object. In contrast, the other uses the typical array syntax to fetch the second item in the list. |
Attribute or Attr | When an attribute is returned (e.g., by the createAttribute() method, it acts as an object reference that exposes a special outlook for other attributes. |
NamedNodeMap | A namedNodeMap is like an array, but the items are accessed by name or index. Items can be added or removed from a namedNodeMap. |
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
Core interfaces in the DOM
The document and window objects are the objects whose interfaces we mostly use often in DOM programming. Basically, the window object represents something like the browser, and the document object is the root of the document itself. The following is a brief list of common APIs in web and XML page scripting using the DOM.
[document.querySelector(selector)](https://developer.mozilla.org/en-US/docs/Web/API/Document/querySelector)
[document.querySelectorAll(name)](https://developer.mozilla.org/en-US/docs/Web/API/Document/querySelectorAll)
[document.createElement(name)](https://developer.mozilla.org/en-US/docs/Web/API/Document/createElement)
[parentNode.appendChild(node)](https://developer.mozilla.org/en-US/docs/Web/API/Node/appendChild)
[element.innerHTML](https://developer.mozilla.org/en-US/docs/Web/API/Element/innerHTML)
[element.style.left](https://www.w3schools.com/jsref/prop_style_left.asp)
[element.setAttribute()](https://developer.mozilla.org/en-US/docs/Web/API/Element/setAttribute)
[element.getAttribute()](https://developer.mozilla.org/en-US/docs/Web/API/Element/getAttribute)
[element.addEventListener()](http://w3schools.com/jsref/met_element_addeventlistener.asp)
[window.content](https://developer.mozilla.org/en-US/docs/Web/API/Window/content)
[GlobalEventHandlers/onload](https://reference.codeproject.com/dom/GlobalEventHandlers/onload)
[window.scrollTo()](https://developer.mozilla.org/en-US/docs/Web/API/Window/scrollTo)
DOM modification using Code examples
How to set text content;
-
To get the text content of a node and its descendants, we will use the
textContent
property: let text = node.textContent; -
And we have the following HTML snippet already:
<div id="note">
JavaScript textContent Demo
<span style="display:none">Hidden Text</span>
</div>
- This example will use the
textContent
property to get the text of the<div>
element:
let note = document.getElementById('note');
console.log(note.textContent);
The above code is explained below;
-
First, select the
div
element with the id note using thegetElementById
method. -
Then, display the node’s text by accessing the
textContent
property.
The Code Output;
JavaScript textContent Demo
Hidden Text
- As you can see clearly from the output, the
textContent
property returns the concatenation of thetextContent
of every child node.
How to add a Child Element
The following example uses the appendChild()
method to add three list items to the <ul>
element:
- Assuming that we have the following HTML code:
<ul id="menu">
</ul>
- We create the following JavaScript code;
function createMenuItem(name) {
let li = document.createElement('li');
li.textContent = name;
return li;
}
// get the ul#menu
const menu = document.querySelector('#menu');
// add menu item
menu.appendChild(createMenuItem('Home'));
menu.appendChild(createMenuItem('Services'));
menu.appendChild(createMenuItem('About Us'));
The code is explained below:
- First, the
createMenuItem()
function creates a new list item element with a specified name using thecreateElement()
method. - After which, we elect the
<ul>
element with idmenu
using thequerySelector()
method. - Finally, we call the
createMenuItem()
function to create a new menu item and use theappendChild()
method to append the menu item to the<ul>
element.
Code Output;
How to get a collection of elements in the document
We will use the getElementsByTagName()
method in HTML to return the collection of all the elements in the document with a given tag name.
Syntax:
var elements = document.getElementsByTagName(name);
Where:
- elements is a collection of all the found elements in the order they appear with the given tag name in the document.
- name is a string representing the name of the elements.
Code Example
<!DOCTYPE html>
<html>
<head>
<title>DOM getElementsByTagName() Method</title>
</head>
<body style = "text-align: center">
<h1 style = "color: green;">
Great minds
</h1>
<h2 >
DOM getElementsByTagName()
</h2>
<p>A computer science portal for nerds.</p>
<button onclick="nerd()">Try it</button>
<script>
function nerd() {
var doc = document.getElementsByTagName("p");
doc[0].style.background = "green";
doc[0].style.color = "white";
}
</script>
</body>
</html>
The code is explained below:
- First, we created a
<p>
tag. - Secondly, we created a function called
nerd()
and used thegetElementsByTagName()
method to call the paragraph “p”.
- When a user clicks “Try it”, the text written in the
<p>
tag shows up in green.
Summary
In this tutorial, we explicitly defined the DOM, identified its features and methods, and introduced a demo code that shows how to create the DOM. We also outlined its fundamental data types and core interfaces then, illustrated how to modify the DOM with code examples. It is important to know that a lot can be done using the DOM methods and properties. I believe this tutorial has given the reader better insight and understanding of how to create and modify DOM in JavaScript.
Resources
- https://gabrieltanner.org/blog/javascript-dom-introduction/
- https://linuxhint.com/html-dom-methods-and-properties/
- https://www.javascripttutorial.net/dom/manipulating/create-a-dom-element/
- https://learn.saylor.org/mod/book/view.php?id=36824&chapterid=20206
A TIP FROM THE EDITOR: For a related topic, don’t miss our Shadow DOM: the ultimate guide article.