Axios vs Fetch API: The Definitive Guide to HTTP Requests in 2025
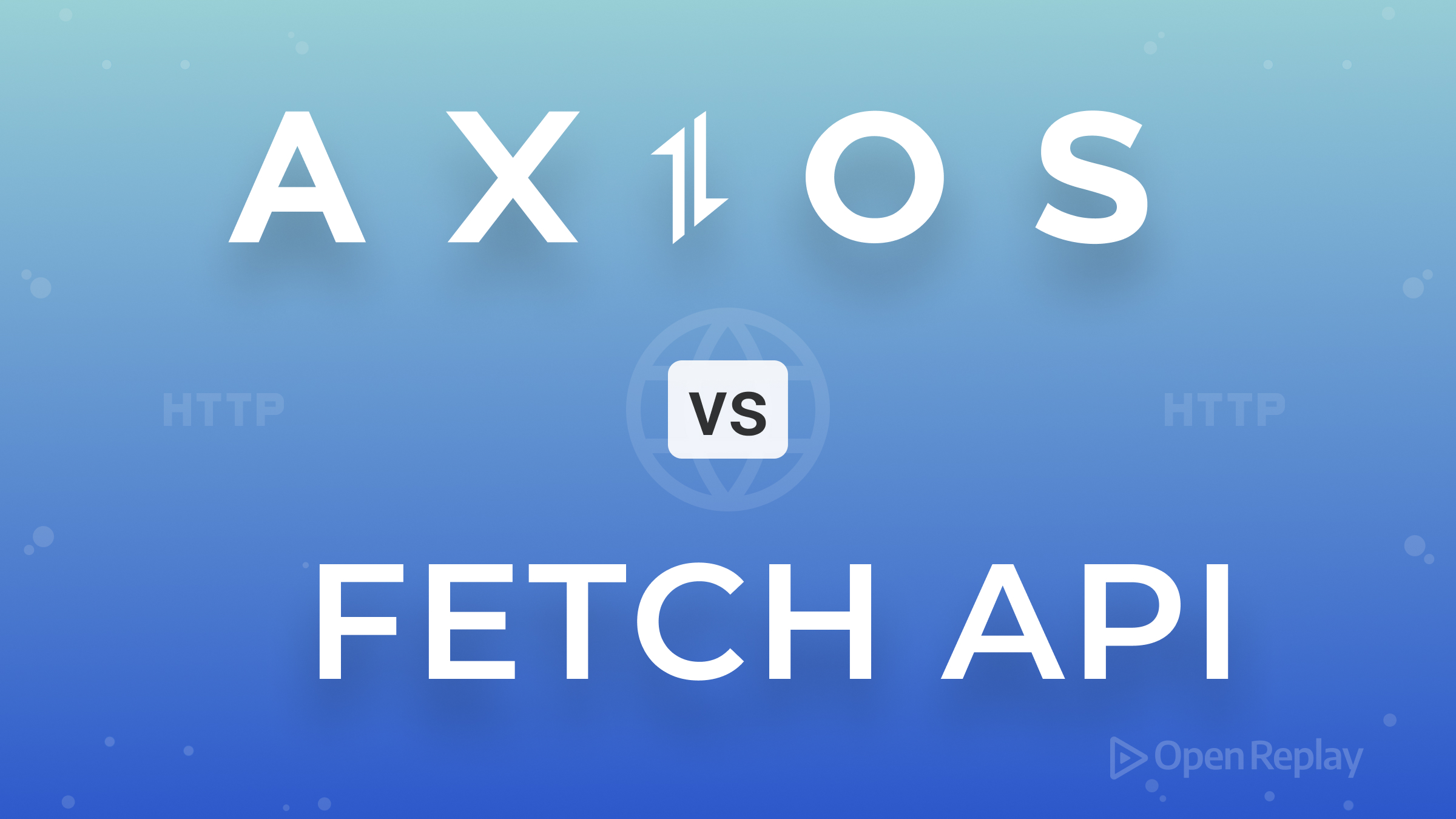
Making HTTP requests is a daily task for web developers, but choosing the right tool can save you hours of frustration. Axios and Fetch API both promise to simplify network communication, but they’re not created equal. This guide cuts through the noise to help you pick the right library for your project.
Key Takeaways
- Axios offers more features and easier error handling compared to Fetch API
- Fetch API provides native browser support without additional dependencies
- Choosing between Axios and Fetch depends on project complexity and requirements
- Both libraries have unique strengths for different use cases
Understanding HTTP Request Libraries
Axios vs Fetch API: Core Differences
Axios Advantages
- Simplified API with dedicated methods for different HTTP verbs
- Automatic JSON parsing and stringification
- Built-in request and response interceptors
- More intuitive error handling
Fetch API Limitations
- Requires manual JSON parsing
- More verbose configuration
- Requires additional setup for advanced features
Request Methods Comparison
Axios Request Methods:
axios.get(url[, config])
axios.post(url[, data[, config]])
axios.put(url[, data[, config]])
axios.patch(url[, data[, config]])
axios.delete(url[, config])
Fetch API Equivalent:
fetch(url, {
method: 'GET', // or POST, PUT, PATCH, DELETE
headers: {},
body: JSON.stringify(data)
})
Error Handling Comparison
Axios Error Handling:
axios.get(url)
.then(response => console.log(response.data))
.catch(error => console.error('Request failed', error));
Fetch API Error Handling:
fetch(url)
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.catch(error => console.error('Request failed', error));
Timeout and Request Cancellation
Axios Timeout:
axios.get(url, { timeout: 5000 });
Fetch API Timeout:
const controller = new AbortController();
const timeoutId = setTimeout(() => controller.abort(), 5000);
fetch(url, { signal: controller.signal })
.then(response => response.json())
.catch(err => {
if (err.name === 'AbortError') {
console.log('Request timed out');
}
});
When to Choose Each Library
Recommended Axios Use Cases
- Complex applications requiring advanced request handling
- Projects needing consistent cross-browser behavior
- Applications with complex interceptor requirements
Recommended Fetch API Use Cases
- Lightweight web applications
- Projects targeting modern browsers
- Minimal HTTP request needs
- Avoiding external dependencies
Practical Implementation
Axios Example
const fetchData = async () => {
try {
const response = await axios.get('https://api.example.com/data');
console.log(response.data);
} catch (error) {
console.error('Error fetching data', error);
}
};
Fetch API Example
const fetchData = async () => {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error fetching data', error);
}
};
Useful Resources
Conclusion
Both Axios and Fetch API are powerful tools for making HTTP requests, each with unique strengths. Axios provides a more feature-rich, developer-friendly experience, while Fetch API offers native browser support and simplicity.
For most modern web applications, Axios remains the recommended choice due to its robust feature set, ease of use, and consistent behavior across different environments.
FAQs
It depends on your project needs. Axios offers more features and easier error handling, while Fetch API provides native browser support without additional dependencies.
Yes, Axios is a third-party library that needs to be installed via npm or included via CDN. Fetch API is built into modern browsers.
Yes, Axios works seamlessly in both browser and Node.js environments, making it a versatile choice for full-stack JavaScript development.
For simple requests, Fetch API might have a slight performance advantage. For complex scenarios, Axios provides more comprehensive features with minimal performance overhead.
Axios provides a more straightforward method for file uploads with built-in support for FormData. With Fetch API, you'll need to manually construct the request.