How to Create Toast Messages in React with Toastify
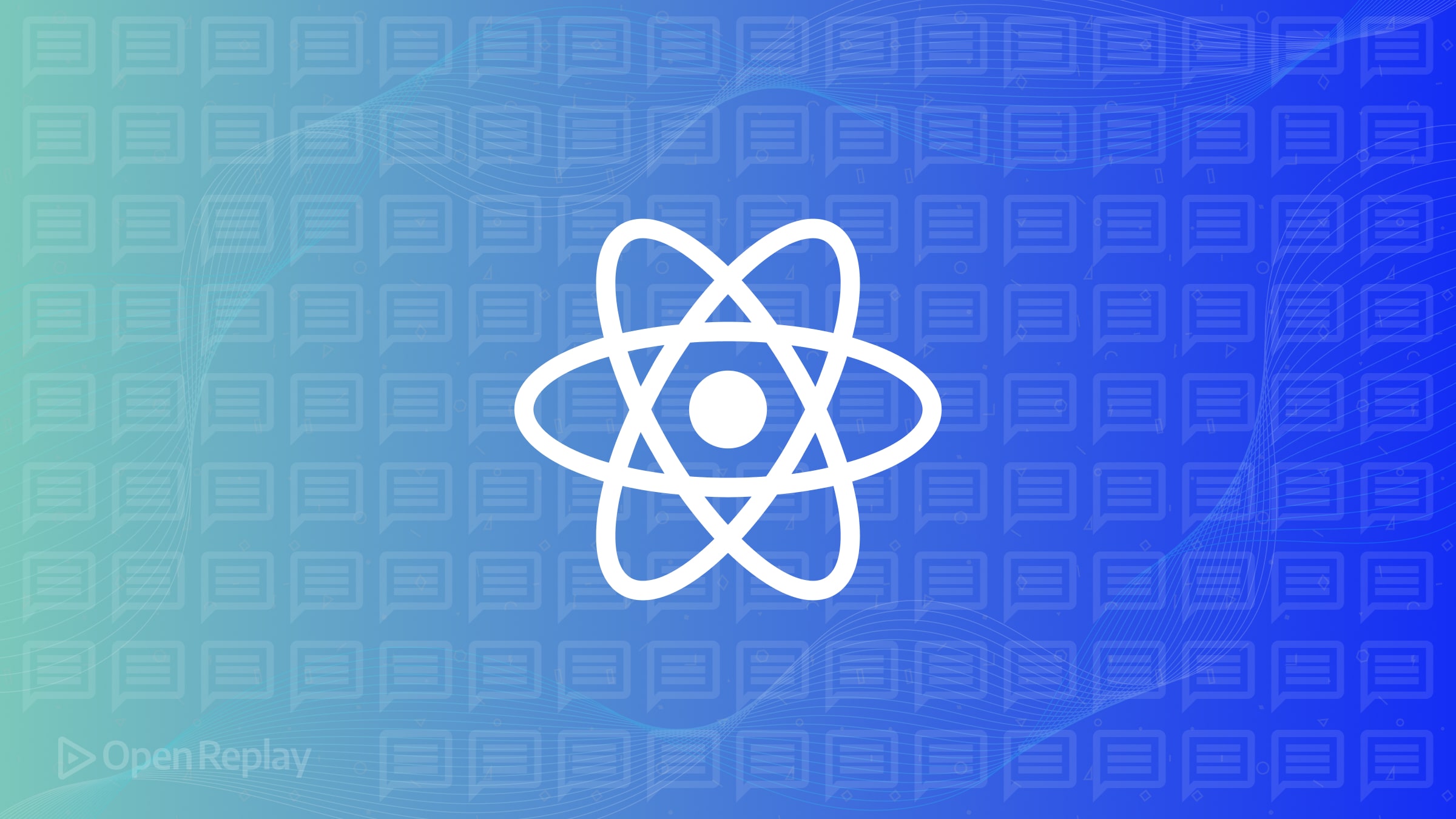
Toast notifications are quick, non-intrusive alerts that keep users informed without disrupting their workflow. React-Toastify simplifies implementing these notifications, balancing ease-of-use with customization.
Key Takeaways
- React-Toastify simplifies adding toast notifications.
- Supports multiple notification types (success, error, warning, info).
- Customizable positions and styles.
- Offers advanced features like promise-based notifications.
Getting Started with React-Toastify
Installation
Install React-Toastify:
npm install react-toastify
# or
yarn add react-toastify
Basic Setup
Import the necessary components and CSS:
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
Place ToastContainer
in your main App:
function App() {
return (
<div>
<button onClick={() => toast(""Hello, Toastify!"")}>Notify</button>
<ToastContainer />
</div>
);
}
Types of Toast Notifications
Use specific toast types for clarity:
- Success:
toast.success(""Saved successfully!"");
- Error:
toast.error(""An error occurred!"");
- Warning:
toast.warning(""Check your input!"");
- Info:
toast.info(""Update available!"");
- Default:
toast(""Neutral message"");
Customizing Toast Notifications
Positioning
Customize toast positions:
toast(""Message"", { position: ""bottom-center"" });
Positions include top-right
, top-center
, top-left
, bottom-right
, bottom-center
, and bottom-left
.
Styling
Apply custom styles:
toast(""Styled toast"", { className: ""custom-toast"" });
CSS example:
.custom-toast {
background: navy;
color: white;
border-radius: 4px;
}
Conditional Styling
Style based on type:
const notify = (type, message) => {
toast[type](message, { className: `${type}-toast` });
};
Advanced Features
Promise-based Toasts
Handle async operations seamlessly:
const promise = fetch('url').then(res => res.json());
toast.promise(promise, {
pending: ""Loading..."",
success: ""Loaded successfully!"",
error: ""Failed to load data.""
});
Custom Content
Include React components:
toast(<div>Custom HTML content <button onClick={() => alert('Clicked!')}>Click</button></div>);
Notification Center
Track notifications easily:
import { useNotificationCenter } from 'react-toastify/addons/use-notification-center';
const NotificationCenter = () => {
const { notifications, markAllAsRead } = useNotificationCenter();
return (
<div>
<button onClick={markAllAsRead}>Mark all read</button>
{notifications.map(n => <div key={n.id}>{n.content}</div>)}
</div>
);
};
Best Practices
- Use Sparingly: Avoid overwhelming users.
- Choose Appropriate Types: Clearly communicate message intent.
- Set Duration Carefully: Important messages require longer visibility.
- Accessibility: Ensure readable content and clear contrast.
- Concise Messages: Clearly indicate what’s needed.
Complete Implementation Example
import React, { useState } from ""react"";
import { ToastContainer, toast } from ""react-toastify"";
import ""react-toastify/dist/ReactToastify.css"";
function ProfileForm() {
const [data, setData] = useState({ username: """", email: """" });
const handleSubmit = (e) => {
e.preventDefault();
if (!data.username || !data.email) {
toast.warning(""All fields required!"");
return;
}
const saveData = new Promise((resolve, reject) => {
setTimeout(() => {
data.email.includes(""@"") ? resolve() : reject();
}, 1500);
});
toast.promise(saveData, {
pending: ""Saving..."",
success: ""Profile updated!"",
error: ""Invalid email!""
});
};
return (
<form onSubmit={handleSubmit}>
<input
type=""text""
placeholder=""Username""
value={data.username}
onChange={(e) => setData({ ...data, username: e.target.value })}
/>
<input
type=""email""
placeholder=""Email""
value={data.email}
onChange={(e) => setData({ ...data, email: e.target.value })}
/>
<button type=""submit"">Submit</button>
<ToastContainer />
</form>
);
}
Conclusion
React-Toastify helps create effective, unobtrusive notifications in React apps. Its simplicity and flexibility enable rapid integration while allowing deep customization to fit your app’s needs.
FAQs
Yes, React-Toastify supports custom React components as toast content.
Set `autoClose: false` in the toast options to persist notifications.
Yes, use `toast.update(toastId, options)` with the toast's ID.
Use the `limit` prop on `ToastContainer` to control simultaneous notifications.
Yes, ensure client-side imports and rendering, especially with frameworks like Next.js.