Next.js or SvelteKit: which one should you use for your next project?
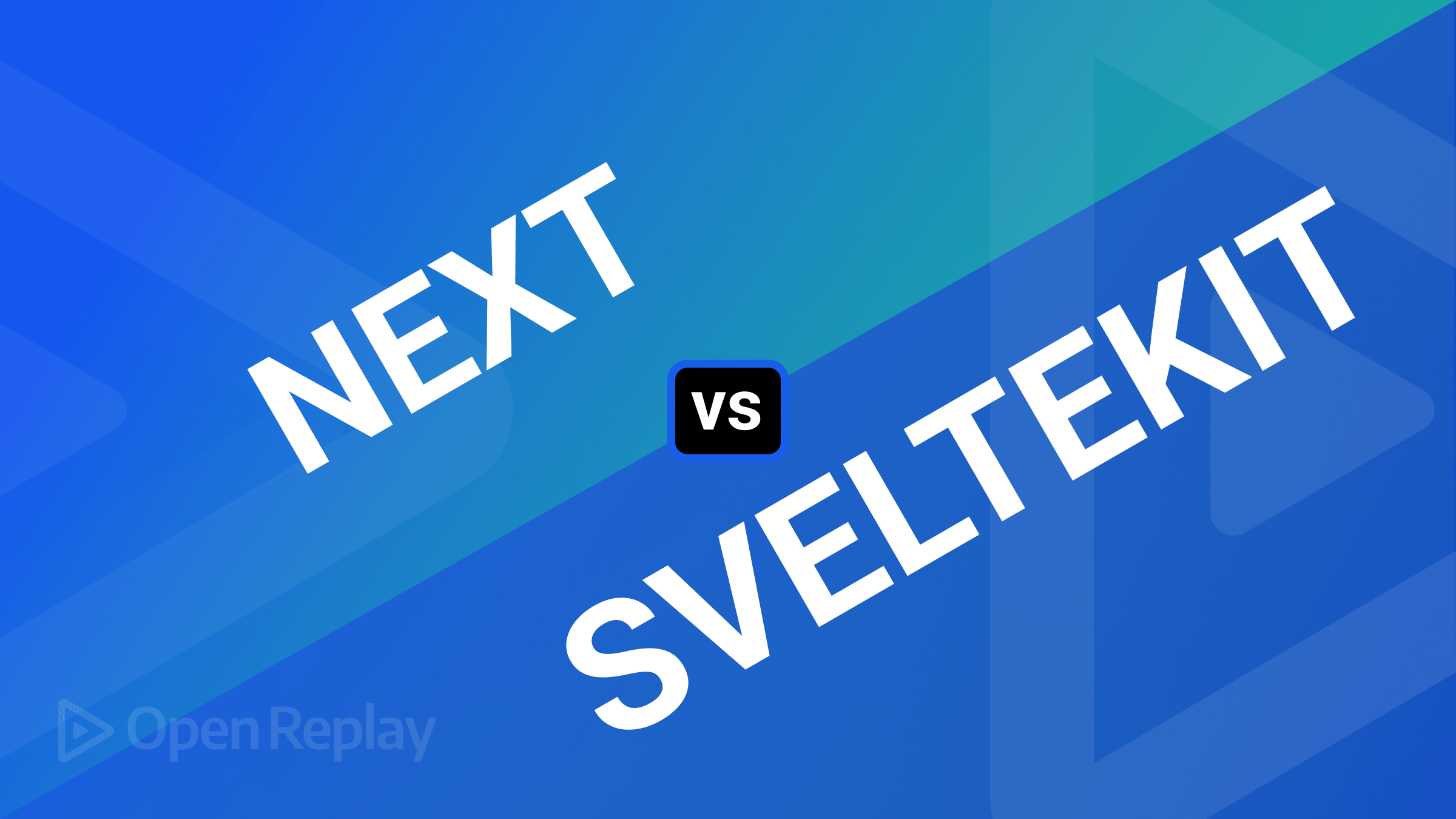
Next.js and SvelteKit are incredibly popular frameworks for building JavaScript web applications. Both of them provide a variety of features and advantages, and I’ll compare them in this article to assist you in selecting the best one for your next project.
What is Next.js?
Next.js is an open-source popular JavaScript framework for building server-side rendered (SSR) React applications. It is developed and maintained by the company Vercel. Next.js is designed to make it easy to create fast, dynamic, and scalable web applications that deliver a seamless user experience. As a framework, it provides a simple and intuitive API, making it easy for developers to build web applications quickly. It ensures that your website is compatible with a wide range of browsers, so you don’t have to worry about compatibility issues. Developers who have experience with React are increasingly using the framework. React offers a virtual DOM program and server-side rendering, enhancing app speed. Additionally, it is becoming increasingly well-liked among those who require the flexibility of a hybrid architecture that combines server-side rendering (SSR) and static-site generation (SSG) in a single project. Delivering full-stack capability to the front end is the purpose of Next.js.
Sites made with Next.js include:
Here are some of its features:
Server-side Rendering (SSR)
One of the most important features of Next.js is its ability to perform server-side rendering (SSR). This means that the HTML is generated on the server and sent to the client, providing a fast and optimized first-load experience.
For example, we have a component that returns a welcome message and a link to the “About” page. The Link
component handles navigation between pages in the application.
import Link from 'next/link';
function HomePage() {
return (
<div>
<h1>Welcome to Next.js!</h1>
<Link href="/about">
<a>About</a>
</Link>
</div>
);
}
export default HomePage;
Here, the Link
component handles navigation between pages in the application. When the user clicks on the “About” link, they will be taken to the “About” page, defined in another Next.js component. This allows for seamless navigation between pages in the application while providing the benefits of server-side renderings, such as improved load times and optimized performance.
Automatic Code Splitting
Another great feature of Next.js is its ability to split your code into smaller chunks and only load the parts of your application that are needed. This helps keep your initial load times fast, even as your application grows in size.
The example below has a component that returns information about Next.js. The Head
component is used to set the title of the page for better SEO.
import Head from 'next/head';
function AboutPage() {
return (
<div>
<Head>
<title>About | Next.js Example</title>
</Head>
<h1>About Next.js</h1>
<p>Next.js is a popular React-based JavaScript framework.</p>
</div>
);
}
export default AboutPage
The Head
component is used to set the title of the page, which is an important aspect of search engine optimization (SEO). Using the Head component, we can ensure that the page’s title is set correctly, even when the page is dynamically generated on the server.
Static File Serving
Next.js also makes it easy to serve static files, such as images, fonts, and stylesheets. You can simply place your static files in a public
directory at the root of your project, and they will be available to be served by Next.js. In the code below, we have a Next.js component that displays a welcome message and an image of the Next.js logo. The styles
module is used to set the styling of the page.
import styles from '../styles/Home.module.css';
function Home
What is Sveltekit?
SvelteKit is a new framework from the creators of the popular JavaScript library Svelte, aimed at making it easier for developers to build web applications with a modern and efficient approach. SvelteKit is built on top of Svelte and provides a comprehensive suite of tools and features for building, deploying, and scaling web applications. It is known for its approach to building web applications by compiling components to highly optimized JavaScript code during the build process, making it faster and more efficient than other frameworks that rely on runtime computations. SvelteKit takes this one step further by providing a complete end-to-end solution for building and deploying web applications. In addition, it allows you to focus on building and refining your web applications without having to worry about underlying infrastructure or deployment processes.
Sites made with SvelteKit:
- Svelte.dev
- nytimes.com
- daisyui.com
SvelteKit offers a wide range of features. Here are some features of SvelteKit:
Easy setup
SvelteKit makes getting started with a new project incredibly simple. With just a few commands, you can create a new project and have a working application up and running in no time.
npx @sveltejs/kit init my-app
cd my-app
npm run dev
The first command creates a new SvelteKit project named “my-app”. The second command changes to the project directory, and the third command starts a local development server.
Component-based architecture
SvelteKit follows a component-based architecture, where each piece of UI is represented as a self-contained component. This makes it easy to manage and maintain your application and enables you to reuse components across your application.
<script>
let name = "World";
</script>
<h1>Hello {name}!</h1>
We define a component that displays a greeting to the user. The component has a single property called name
that can be used to customize the greeting. The name
property is set to “World” by default, but it can be overridden by passing a new value to the component.
Server-side rendering
SvelteKit provides built-in server-side rendering, making it easy to generate HTML on the server and deliver it to the client. This results in faster initial load times and better SEO.
<script context="module">
export async function load({ page }) {
const res = await fetch(`https://api.example.com/posts/${page.params.slug}`);
const post = await res.json();
return { props: { post } };
}
</script>
<script>
export let post;
</script>
<h1>{post.title}</h1>
<p>{post.content}</p>
Here, we define a SvelteKit page that displays a blog post. The load function is used to fetch the blog post from an API and pass it as a prop to the page. The page is rendered on the server and sent to the client as HTML.
Static site generation
SvelteKit also supports static site generation, allowing you to build fast, lightweight, and scalable websites that can be easily hosted and served from a CDN.
npm run build
npm run export
The first command builds your SvelteKit application for production. The second command exports your application as a set of static files that can be easily hosted and served from a CDN.
Routing
Another important feature of SvelteKit is its powerful routing system. With SvelteKit, you can easily define routes for your application, and the framework will automatically handle the navigation between different pages. Here’s an example of how to define routes in SvelteKit:
<svelte:head>
<title>My App</title>
</svelte:head>
<nav>
<a href="/" class:active={segment === ''}>Home</a>
<a href="/about" class:active={segment === 'about'}>About</a>
<a href="/contact" class:active={segment === 'contact'}>Contact</a>
</nav>
<main>
<svelte:component this={segment} />
</main>
<script>
import Home from './routes/Home.svelte';
import About from './routes/About.svelte';
import Contact from './routes/Contact.svelte';
const routes = {
'': Home,
'about': About,
'contact': Contact
};
export default {
components: routes,
preload({ query }) {
return {
segment: query.s
};
}
};
</script>
In the example above, the routes
object maps the URL segments to components. The segment
is passed to the preload
function, which is executed on the server, and the value is then available in the component. The main
section then uses the segment
to determine which component to render.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
Comparing Next.js and Sveltekit
In this section, I will give brief similarities and differences between the two frameworks.
Common features
Next.js and SvelteKit have built-in server-side rendering support, allowing for better performance, improved SEO, and a more seamless user experience. They provide a straightforward way to define and handle routing in your application. Both follow a component-based architecture, which makes it easy to maintain and structure your codebase. In addition, both frameworks have a modern development experience, with features like hot-reloading, easy build, and deployment. They aim to deliver a fast and responsive user experience.
Tooling
Next.js and SvelteKit differ in their tooling. Next.js is part of the React ecosystem, which means that it has a rich set of tools and libraries that you can use to build your applications. This includes tools like Create React App, React DevTools, and a large number of plugins for popular code editors like Visual Studio Code. SvelteKit, on the other hand, is a relatively new framework and has a smaller set of tools and libraries available. The Svelte community is growing quickly, and new tools are being developed.
Architecture
Next.js is a server-side rendered (SSR) framework built on top of React. This means that Next.js generates the HTML for your application on the server and sends it down to the browser, where JavaScript takes over and makes the page dynamic. This approach can improve the performance and SEO of your application, as the initial load time is faster, and search engines can easily index the content. Here’s an example of a component in Next.js:
import React from 'react';
function HomePage() {
return <h1>Hello, Next.js!</h1>;
}
export default HomePage;
SvelteKit, on the other hand, is a client-side framework built on top of the Svelte library. SvelteKit generates the JavaScript for your application on the client, and the page is fully rendered in the browser. This approach is generally faster and more efficient than SSR, as the JavaScript only needs to be downloaded once and can be reused across multiple pages. And here’s an equivalent component in SvelteKit:
<h1>Hello, SvelteKit!</h1>
Routing
Another key difference between Next.js and SvelteKit is their approach to routing. Next.js has built-in support for client-side routing, which allows you to navigate between different pages in your application without reloading the entire page. This is done using the Link
component and the useRouter
hook.
Here’s an example of routing in Next.js:
import React from 'react';
import Link from 'next/link';
function HomePage() {
return (
<>
<h1>Hello, Next.js!</h1>
<Link href="/about">
<a>About</a>
</Link>
</>
);
}
export default HomePage;
SvelteKit requires you to set up client-side routing yourself. This can be done using a library such as svelte-routing
, but it does require some additional setup.
And here’s an example in SvelteKit:
<script>
import { Link, navigate } from 'svelte-routing';
</script>
<h1>Hello, Sveltekit!</h1>
<Link to="/about">About</Link>
State management
State management is another area where Next.js and SvelteKit differ. Next.js uses the React framework, which means that you have access to all of the popular state management tools and libraries, such as Redux and MobX.
Here’s an example of managing state in Next.js using the useState
hook:
import React, { useState } from 'react';
function HomePage() {
const [count, setCount] = useState(0);
return (
<>
<h1>Hello, Next.js!</h1>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</>
);
}
export default HomePage;
SvelteKit does not have a built-in state management solution. Instead, you can use the Svelte store to manage the state, or you can use a third-party library such as XState or Recoil. Here’s an example in SvelteKit:
<script>
let count = 0;
function increment() {
count += 1;
}
</script>
<h1>Hello, Sveltekit!</h1>
<p>Count: {count}</p>
<button on:click={increment}>Increment</button>
Here is a table comparing Next.js and SvelteKit:
Features | Next.js | SvelteKit |
---|---|---|
Framework | React based | Svelte based |
Language | JavaScript | JavaScript |
Routing | Built-in with good customization options | External library needed, offers limited customization options |
Tooling | Large ecosystem | Growing ecosystem |
Learning curve | Steep for new React users | Shallow for those familiar with Svelte |
Server-side rendering (SSR) | Built-in, supports automatic (SSR) and Static site generation (SSG) | Built-in, supports automatic SSR |
Library size | Larger | Smaller |
Code splitting | Built-in and automatic | External library needed, manual code splitting |
Community support | large and active | Growing, but smaller than Next.js |
Which one should you use on your next project?
Next.js is a good choice if you want a complete, all-in-one solution for building a web application that can handle both server-side and client-side rendering, and if you have a complex application with dynamic data and a lot of user interactions, it has a rich ecosystem of plugins and integrations with other technologies. Next.js also provides a suite of features for building performant and scalable web applications, including automatic code splitting, optimized performance, and improved SEO.
On the other hand, SvelteKit is a good choice if you want to build scalable and cost-effective applications with low operational overhead, and if your application has a lot of independent, small tasks that can be broken down into separate functions. It allows you to write your application logic as a small, single-purpose function that can be easily deployed and run on a serverless application. In addition, the choice between Next.js and SvelteKit depends on your specific needs and requirement for your project. If you have a complex, data-driven web application, Next.js is probably a better choice, while SvelteKit is better suited for smaller, independent tasks.
Conclusion
Next.js and SvelteKit are both popular frameworks for building applications. The choice between them will depend on your personal preference and the needs of your project, but both frameworks are definitely worth considering for your next project.