Design Systems for Front End Development
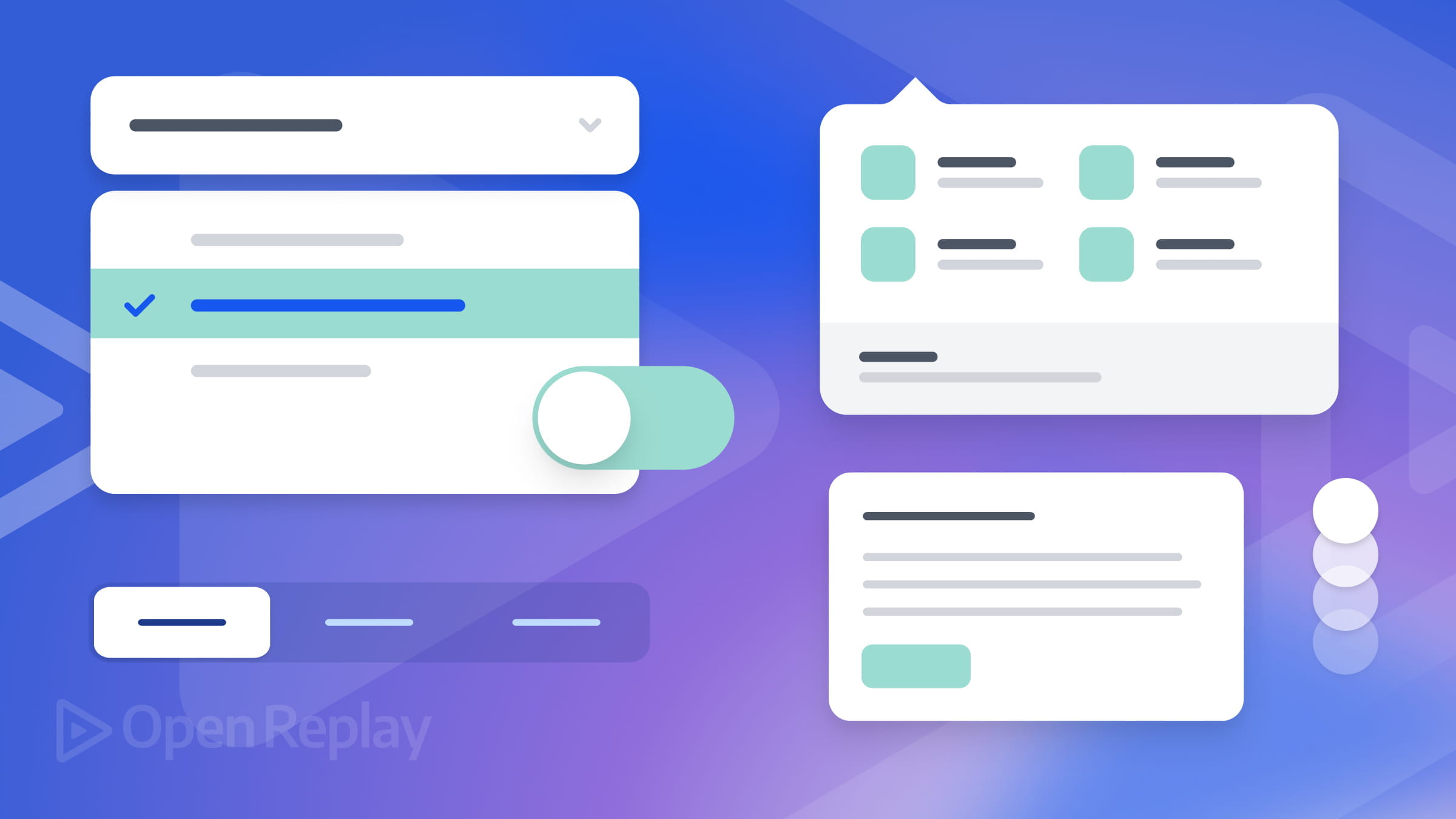
As Steve Jobs once said, “Design is not just what it looks like and feels like. Design is how it works.” Design is the backbone of every product, emphasizing functionality beyond aesthetics, and this article will explain the importance of design systems for your front end work.
Discover how at OpenReplay.com.
Design Systems in Front End Development
Design systems have evolved from the early days of Twitter Bootstrap, transforming into comprehensive collections of reusable components with clear design standards. These systems play a crucial role in supporting application development, enabling businesses to set their mobile and web applications apart while maintaining a consistent visual identity.
Defining Design Systems in Front End
A design system is a collection of reusable components guided by clear standards and design patterns. It goes beyond a mere UI library, encompassing documentation of standards, guidelines, visual language, and accessibility support. Notable technology companies such as Salesforce, Stack Overflow, and Microsoft publicly share their design systems, setting a precedent for best practices.
Key Constituents of a Design System
A design system in front end development comprises various crucial components that work together to ensure consistency, efficiency, and scalability across the user interface. These key constituents include:
Design Language
A guideline for developing products within a brand, covering colors, typography, spacing, imagery, motion dynamics, and audio cues. Design inconsistency can arise when different teams create their own UIs independently, making a design language crucial for maintaining a unified experience.
Component Library
A warehouse of reusable components that form the core building blocks of an application. Responsive, customizable, and accessible component libraries can be implemented using various technologies such as React, Vue, Angular, or Vanilla Javascript.
Style Guide
The user manual for the design language and component library consolidates essential information for designers and developers. It serves as a single, accessible location for guidelines and installation protocols.
Benefits of a Design System
A well-implemented design system in front end development offers a lot of advantages, fostering efficiency, collaboration, and a quality user experience. Some of these key benefits include:
Scalability
Scalability in the context of design systems refers to the system’s ability to maintain coherence and uniformity as a project grows, accommodating an expanding team. As a company or project scales, involving more designers and developers, a well-established design system serves as a guiding framework. It ensures that regardless of the size of the team, the user interface (UI) remains consistent. This consistency not only streamlines communication and collaboration but also contributes to overall business growth. A scalable design system becomes a foundation for building and expanding products without sacrificing the integrity of the user experience.
Design Consistency
Design consistency is the cornerstone of a successful design system, emphasizing the creation of a cohesive and uniform user interface (UI) across a spectrum of diverse products or applications. By adhering to established design patterns, color schemes, typography, and other visual elements, a design system reinforces brand identity. It ensures that users can seamlessly transition between different features or products, experiencing a unified and recognizable design language. This consistency not only fosters trust and brand recognition but also enhances the overall user experience by providing a sense of familiarity and coherence.
Faster Development
The implementation of a design system significantly accelerates the development process by minimizing the necessity to create components and styles from scratch. With a comprehensive collection of pre-designed and thoroughly tested UI components available in a design system, development teams can efficiently leverage these building blocks. This allows them to allocate more time and resources to the core aspects of product development, such as implementing unique features, addressing specific user needs, and enhancing overall functionality. Faster development, made possible by reusable components, not only speeds up time-to-market but also enhances the agility of development teams.
Accessibility
Accessibility is a fundamental principle embedded in design systems, advocating for the inclusive design of digital products. A well-constructed design system places a strong emphasis on creating user interfaces that cater to diverse user needs, including those with disabilities. By incorporating accessible design patterns, providing alternative text for images, ensuring proper contrast ratios, and implementing keyboard navigation, a design system promotes a digital environment that is usable by everyone. Prioritizing accessibility not only aligns with ethical design practices but also widens the audience reach, ensuring that the product is accessible and functional for users with varying abilities and preferences.
Crafting Design Systems
Developing a robust design system involves collaboration among various key stakeholders. The participation of designers, developers, product managers, and accessibility experts is vital to ensure the design system aligns seamlessly with customer needs.
Designers
Designers play a role in defining the visual language, creating design patterns, and ensuring a consistent user interface (UI) across the system.
Developers
Developers implement design components into code, ensuring functionality, responsiveness, and compatibility with different technologies.
Product Managers
Product Managers provide strategic direction, aligning the design system with overarching product goals. They prioritize features and ensure the system meets user requirements.
Accessibility Experts
Accessibility experts ensure the design system adheres to accessibility standards, making the product usable by a diverse audience with varying needs.
When Not to Opt for a Design System?
While design systems offer benefits, there are scenarios where their implementation might be unnecessary or less advantageous, especially for smaller-scale projects. Consider the following factors before deciding to opt for a design system:
Project Scale
For smaller projects, the overhead of creating and maintaining a design system may outweigh the benefits. In such cases, foundational design principles can be applied without a comprehensive design system.
Timeline Constraints
In situations where time is critical, such as tight deadlines, investing in a full-fledged design system might not be practical. Rapid development without the constraints of a design system may be more suitable.
Budgetary Constraints
Design systems require resources. If budget constraints are a concern, allocating resources to other critical aspects of the project may take precedence over building a design system.
Flexibility Requirements
Projects demanding a high level of customization might find a rigid design system limiting. In such cases, a more adaptive approach to design may be preferred.
Setting up a Project to Explain Design System
To illustrate the concepts discussed, let’s embark on the practical journey of creating a real-world design system for a blog website. In this example, we’ll utilize Angular, Bootstrap, and CSS to guide you through setting up a project and implementing reusable components for blog posts and comments.
Install Bootstrap in your Angular project
ng add @ng-bootstrap/ng-bootstrap
This command utilizes Angular's CLI
(ng) to add the NgBootstrap
package (@ng-bootstrap/ng-bootstrap) to your Angular project. NgBootstrap is a set of Angular directives for Bootstrap components, enhancing the styling and functionality of your application.
Create a component for your design system
ng generate component design-system
This command generates a new Angular component named design-system. Components in Angular are the building blocks for UI elements. Creating a dedicated component for your design system provides a modular structure to encapsulate and manage related functionalities and styles.
Open the design-system.component.html
file
Add the following code:
<!-- design-system.component.html -->
<div class="container">
<h1>Blog Design System</h1>
<div class="row">
<div class="col-md-8">
<app-blog-post
title="Introduction to Angular"
author="John Doe"
content="Angular is a powerful framework for building web applications."
></app-blog-post>
</div>
<div class="col-md-4">
<app-comment author="Jane Smith" text="Great article!"></app-comment>
<app-comment author="Bob Johnson" text="I learned a lot from this!"></app-comment>
</div>
</div>
</div>
This code sets up a basic layout for a blog page using Angular components. It features a main blog post and a sidebar with two comments. The layout is structured using Bootstrap’s grid system.
Create reusable components for a blog post and a comment
ng generate component blog-post
ng generate component comment
The commands above generate two new Angular components: blog-post
and comment
. These components will represent the reusable building blocks of your blog design system. blog-post
is for displaying blog posts, and comment
is for displaying user comments.
Customize the blog-post.component.html
and comment.component.html
<!-- blog-post.component.html -->
<div class="card">
<div class="card-body">
<h5 class="card-title">{{ title }}</h5>
<p class="card-text"><em>By {{ author }}</em></p>
<p class="card-text">{{ content }}</p>
</div>
</div>
The code above defines a Bootstrap card component for displaying a blog post. The card includes a title (<h5>
) dynamically populated using Angular’s interpolation, an author’s name in italics, and the content of the blog post. The card structure is encapsulated within a <div>
with the class card
, and the content body is defined in a <div>
with the class card-body
. The title, author, and content are dynamically provided using Angular’s data-binding features.
<!-- comment.component.html -->
<div class="card">
<div class="card-body">
<p class="card-text"><em>By {{ author }}</em></p>
<p class="card-text">{{ text }}</p>
</div>
</div>
The code above defines a Bootstrap card component for displaying comments. The card structure includes a body (<div class="card-body">
) containing the author’s name and the comment text. Both the author’s name and comment text are dynamically populated using Angular’s interpolation syntax ({{ author }}
and {{ text }}
).
The blog-post.component.ts
should look like this:`
import { Component, Input } from '@angular/core';
import { DesignSystemComponent } from '../design-system/design-system.component';
@Component({
selector: 'app-blog-post',
standalone: true,
imports: [DesignSystemComponent],
templateUrl: './blog-post.component.html',
styleUrl: './blog-post.component.css',
})
export class BlogPostComponent {
@Input() title!: string;
@Input() author!: string;
@Input() content!: string;
}
The comment.component.ts
should look like this.
import { Component, Input } from '@angular/core';
@Component({
selector: 'app-comment',
standalone: true,
imports: [],
templateUrl: './comment.component.html',
styleUrl: './comment.component.css',
})
export class CommentComponent {
@Input() author!: string;
@Input() text!: string;
}
Style your components
In the styles.css
file:
/* styles.css */
body {
font-family: Arial, sans-serif;
}
.container {
margin-top: 20px;
}
.card {
margin-top: 10px;
}
The CSS code above sets the font family for the entire application, adds spacing to the container and defines margins for the card elements.
Test your design system
ng serve
This command starts the Angular development server, allowing you to preview your design system in action. By visiting http://localhost:4200/ in your browser, you can interact with and observe the blog design system you’ve created.
This is what it looks like on the browser when the server is running.
Metrics and Analytics in Design Systems
In front end development, the adoption of design systems extends beyond visual aesthetics. As teams strive for sustained success, evaluating the long-term impact and effectiveness of these systems becomes crucial. This is where the strategic implementation of metrics and analytics becomes imperative, offering valuable insights to guide ongoing improvements and optimize the overall performance of the design system.
The Significance of Analytics
Understanding the impact and effectiveness of design systems is paramount in front end development. The strategic implementation of analytics provides a guide through which teams can gain valuable insights, steering their efforts toward continuous improvement. By delving into various metrics, teams can not only evaluate usability and performance but also refine their design systems to better align with user expectations and industry standards. This data-driven approach underscores the significance of analytics as a guiding force in the evolution of front end design. Below are the various significance of analytics:
User Satisfaction and Engagement
Assessing user feedback, satisfaction surveys, and engagement rates. Analytics serve as a reliable barometer for understanding how well the design system aligns with user expectations, preferences, and overall ease of interaction. Qualitative insights from user feedback, collected through surveys or direct feedback mechanisms within applications, complement quantitative data, helping identify areas for enhancement.
Development Speed and Efficiency
Measuring development time, code maintainability, and bug resolution speed. Analytics provide a quantitative lens to gauge the impact of design systems on the development lifecycle. Efficiency gains, reflected in reduced development time, enhanced code maintainability, and swift bug resolution, are key indicators of a successful design system. Regularly tracking these metrics enables development teams to quantify the tangible benefits derived from standardized component reuse.
Consistency Across Platforms
Evaluating cross-platform consistency and adherence to design guidelines. Design systems aim to ensure a uniform user experience across diverse platforms and devices. Analytics play a crucial role in assessing how well the design system maintains consistency in user interfaces across web and mobile platforms. Automated tools and manual audits contribute to tracking adherence to design guidelines, fostering a cohesive brand identity.
Leveraging Data-Driven Insights for Continuous Enhancement
In front end development, the application of data-driven insights is instrumental in shaping design systems. Through the scrutiny of metrics and analytics, teams gain a holistic understanding of user interactions, system efficacy, and overall performance.
Identifying Pain Points
Uncovering areas of user frustration or developer inefficiencies. Analytics shed light on specific components or interactions causing user dissatisfaction or impeding development efficiency. This data-driven approach enables targeted improvements, addressing identified pain points to enhance overall system performance.
Iterative Design and Development
Embracing an iterative approach for ongoing refinement. Analytics empower teams to adopt a continuous improvement mindset. By analyzing user interactions, teams can identify opportunities to refine existing components or introduce new ones. This iterative process, aligned with agile development principles, facilitates regular updates and enhancements based on observed data.
Performance Monitoring
Analyzing metrics related to page load times, rendering speed, and resource utilization. Performance analytics are essential for maintaining system efficiency as it scales. Insights into metrics such as page load times and rendering speed help identify potential bottlenecks. Proactive optimization efforts, guided by performance analytics, ensure a seamless user experience even as the application complexity grows.
Adapting to Technological Changes
Staying abreast of technological advancements and evolving industry standards. Analytics provide insights into areas where the design system may require updates or optimizations to align with the latest front end technologies. Whether it involves adopting a new framework or optimizing for emerging devices, data-driven insights enable teams to stay ahead of technological changes.
Conclusion
In conclusion, a well-structured design system bridges the gap between design and engineering, fostering a harmonious user experience across various products and platforms. It transcends being a mere component library, encompassing research, upkeep, and detailed documentation.
Nathan Curtis aptly describes it: “A design system is not a project or a side project. It is a product serving other products.” To succeed, a design system must be esteemed as a valuable asset within the corporate framework, ensuring its assimilation and realizing its full potential.
Truly understand users experience
See every user interaction, feel every frustration and track all hesitations with OpenReplay — the open-source digital experience platform. It can be self-hosted in minutes, giving you complete control over your customer data. . Check our GitHub repo and join the thousands of developers in our community..