A Treasury of Tips, Tricks and Techniques for JavaScript
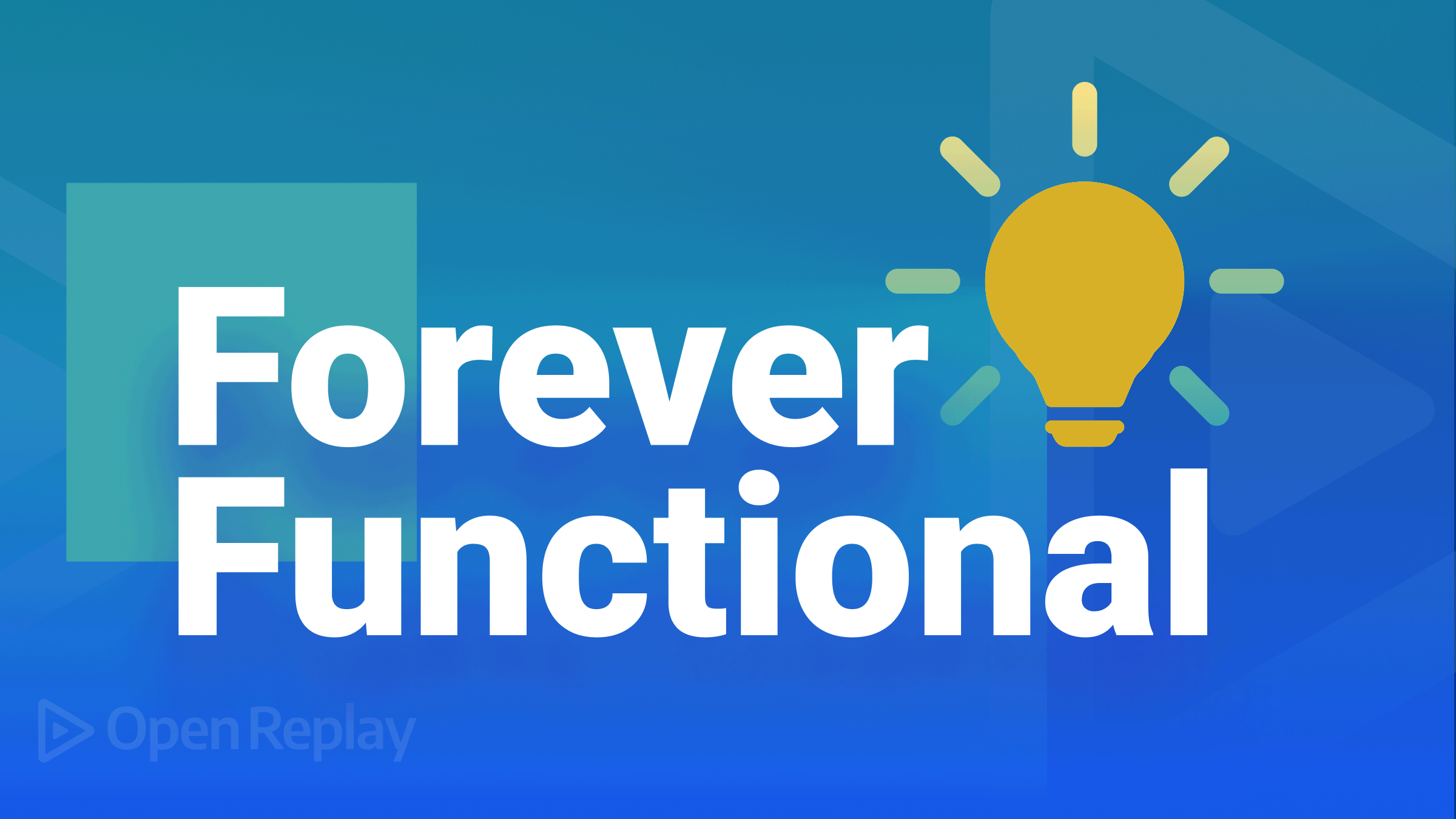
We have published several articles providing tips, tricks and techniques derived from functional programming, and applied properly they let you write shorter, clearer, easily tested and maintained code. Let’s review now all the suggested enhancements for your work here.
Functions
Functions are at the heart of JavaScript programming, and the language offers many interesting (sometimes tricky!) ways to achieve higer performance, shorter code, etc.
- Memoizing Functions for Performance discusses a technique that saves results of previous calls, so if they are needed again you won’t have to redo calculations. This is, by the way, a technique similar to React’s useMemo(…) hook - but more potent!
- From methods to functions, and back shows you how to transform methods (such as .map() or .reduce(), to just mention two) into functions, and how to go the other way, and transform any function of your own into an object’s method.
- Debouncing and throttling for performance introduce two techniques that can avoid firing events repeateadly, thus getting a better performance for your web pages.
- Many Flavors of Currying and Working with functions… but partially! show important functional methods that let you write shorter, clearer code.
- Higher Order Functions shows how you can use functions to modify other functions, wrapping, altering, or creating new functionality out of previous functions, including examples such as timing, logging, and more.
- Pointfree Style Programming lets you work in a clearer way, with shorter, more readable functions.
Objects and methods
It’s sometimes considered that JavaScript really isn’t an Object Oriented Programming language, but the fact is that it provides all needed features for working with classes and methods, and the following list provides several good solutions to achieve clearer and safer code.
- The Mighty Reduce and The Mighty Reduce, Part 2 show you how to apply the powerful .reduce(…) method in many ways.
- Immutable Objects for Safer State introduces the concept of immutability, needed in frameworks such as React, when using Redux — with it, you can understand several ways of avoiding unwanted or undesired changes to objects, thus making impossible several types of errors.
- Generating better, functional ranges for loops describes a technique that lets you write loops in a shorter, clearer, declarative-style way, avoiding errors such as “off by one” bugs. This article also shows a way to use generators for best performance, which is a plus.
- Chaining Calls for Fluent Interfaces shows how to write your own API in a way that will simplify calling a series of methods.
Open Source Session Replay
OpenReplay is an open-source, session replay suite that lets you see what users do on your web app, helping you troubleshoot issues faster. OpenReplay is self-hosted for full control over your data.
Start enjoying your debugging experience - start using OpenReplay for free.
Promises
Working with promises is the modern way to do async work. We have discussed the topic in several articles:
- Memoizing Promises shows how you can avoid making repeated calls to APIs, by internally saving previous results and using them when needed
- Waiting with Promises discusses how to implement “wait loops” (in which you just have to make time until some condition occurs) in an efficient way.
Algorithms
In this section let’s mention a couple of articles that provide a lot of algorithms, with immediate application for your own code.
- Sorting recipes for JavaScript describes how to use JavaScript’s own .sort(…) method to sort all kinds of data.
- Shuffling an Array provides a solution often needed for games: how to put a list of elements in random order — something you could use to “shuffle” a deck of cards, for example.
Frameworks
Frameworks are commonly used for both front- and back-end work, but how to properly structure your project is not easy, and these articles show you how to organize your work, to get more maintainable and testable code.
- Structuring a React project functionally describes a way to plan your React project, including considerations for state, services, and routing.
- Dependency Injection and Higher Order Routing in Node.js does a similar job, by showing how to plan and organize your Node.js project to enable better testing and maintenance.
- Injecting for Purity describes a key design pattern, at the root of the previous two articles, that enables better structured projects and simpler testing for your code.
A TIP FROM THE EDITOR: Several of the articles shown here let you work in a more declarative way; learn more about this by reading our Programming - Imperative vs Declarative article.