Incremental Static Regeneration (ISR) in Next.js
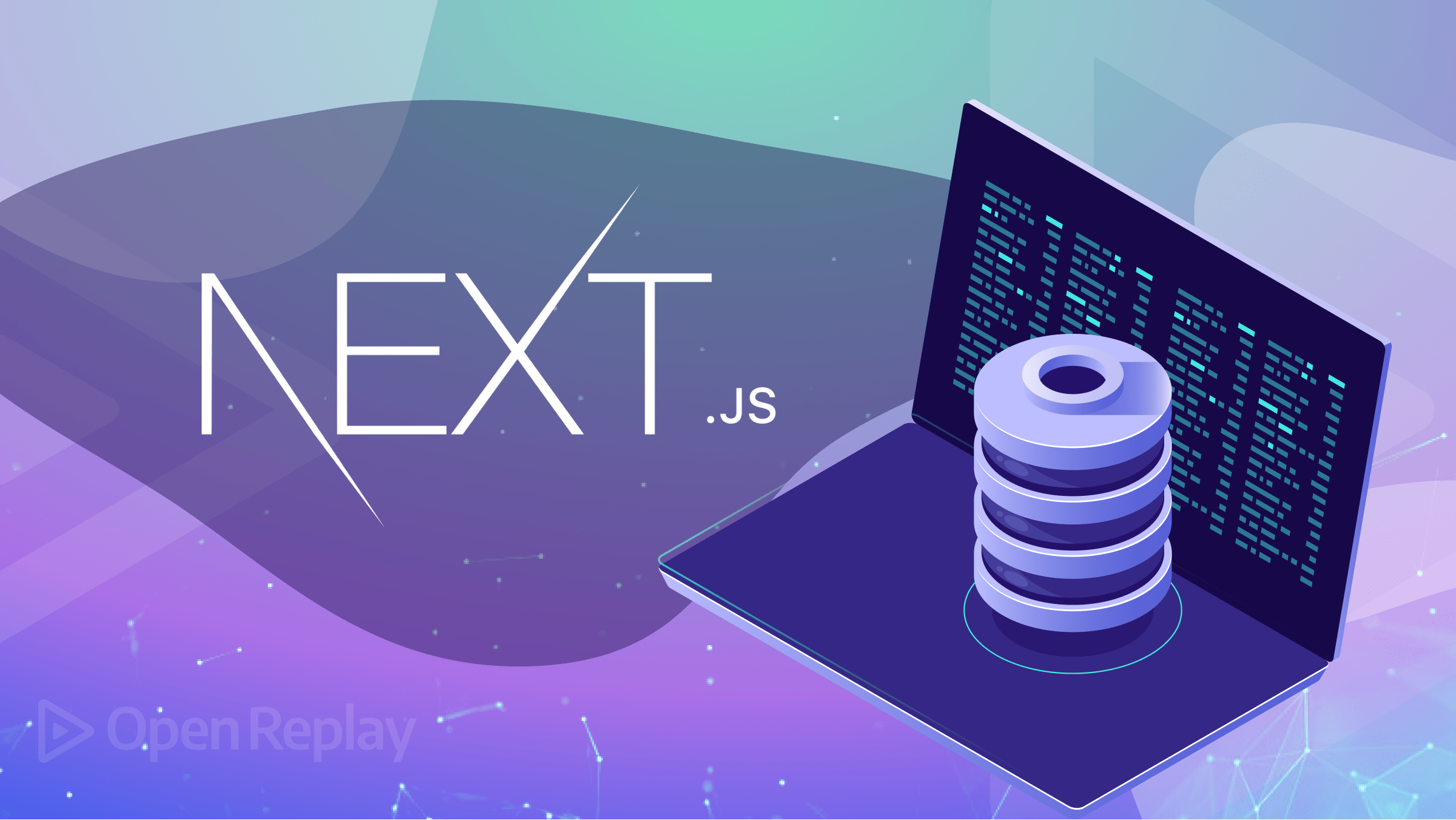
Incremental Static Regeneration (ISR) is a feature provided by the Next.js framework that allows pages to be rendered server-side so users will get data faster, for a better user experience. This article will explain how all of this works and how and when you can take advantage of this new possibility.
Nextjs is a React framework with a feature called Incremental Static Regeneration (ISR). In today’s digital landscape, users expect websites to load quickly and provide up-to-date information. Incremental Static Regeneration changes how websites are generated by enabling up-to-date content while benefiting from static pages’ speed and efficiency. It allows developers to create and serve pre-rendered pages on demand, balancing real-time updates and optimized performance. Traditionally, static site generation (SSG) involves generating all pages during the build process. While this approach ensures consistent and performant page loads, it falls short when displaying real-time or frequently updated content. On the other hand, server-side rendering (SSR) generates each page on every request, resulting in slower response times and increased server load.
Incremental Static Regeneration bridges this gap by introducing a layer on the statically generated pages. It allows you to define a revalidation period for each page, indicating how frequently the page should be regenerated in the background. The previously generated static page is served during this period, providing instant loading times. Once the revalidation period expires, Nextjs automatically renews the page, ensuring it has the latest data. This article will cover the benefits, problems, and use cases for Incremental Static Regeneration, how ISR differs from static site regeneration, and how ISR works in Nextjs.
Prerequisites: Familiarity with JavaScript and a basic understanding of Nextjs and its features.
Benefits of Incremental Static Regeneration
There are several benefits to using ISR in Nextjs:
-
Real-Time Updates: One advantage of Incremental Static Regeneration is its ability to update pages dynamically. By defining a revalidation period for each, Nextjs automatically regenerates and updates the stale pages in the background. It ensures users always have access to the most recent data. You can set the revalidation interval based on the frequency of content updates, striking a balance between real-time updates and server load.
-
Personalized Content: With Incremental Static Regeneration, you can generate personalized static pages based on user-specific data. You can fetch and render content tailored to users, using request parameters or cookies.
-
SEO-Friendly: ISR helps improve search engine optimization (SEO) by generating pre-rendered static HTML files. These files are easily accessible by search engine bots, resulting in better indexing and improved visibility in search engine results, ensuring that important pages are pre-rendered and up-to-date; ISR contributes to better SEO practices.
-
Developer Experience: Nextjs makes it easy to implement ISR with built-in support. Developers can utilize Nextjs intuitive APIs and configuration options to define revalidation intervals and handle content updates. It streamlines the development process and allows developers to focus on building performant web applications.
-
Cost: By leveraging ISR, you can reduce the resources required during the build process. The cost efficiency makes ISR attractive for projects with budget constraints or limited resources.
Problems Associated with Incremental Static Regeneration
There are a few challenges that ISR developers should be aware of. Here are some problems that can arise when using ISR:
-
Cache Invalidation: Caching can be a concern when using ISR. If the caching mechanism is not managed correctly, and the cache contents are not correctly invalidated when content updates occur, users may continue to see stale content even after regeneration. Developers must carefully consider caching strategies and ensure that cache invalidation mechanisms are in place to deliver new or fresh content to users.
-
Compatibility with External Data Sources: ISR relies on data from external sources, such as APIs or databases, to provide content updates. Ensuring compatibility and synchronization between the data sources and the regeneration process can be challenging, especially when dealing with distributed systems or third-party APIs.
-
Increased Complexity: Implementing ISR requires additional configuration and consideration. Developers must handle edge cases where content updates may occur during page regeneration. This increased complexity can make debugging and development difficult.
-
Server Load: Background regeneration processes can add additional server load. If many pages require frequent regeneration, it can impact server resources and result in slower regeneration with ISR
Some tips to help you counteract the problems associated with Incremental Static Regeneration include:
- Only use ISR for pages that need to be updated frequently.
- Ensure that your revalidation logic is efficient and that it only revalidates pages that have changed.
- Keep an eye on your server load to ensure that ISR is not causing too much of a problem.
- Test your pages to ensure they still perform well after enabling ISR.
How Incremental Static Regeneration differs from Static Site Generation
Incremental Static Regeneration(ISR) and Static Site generation (SSG) are two approaches to generating static HTML files in Nextjs, but they differ in how and when the pages are generated. In Static Site, all the static pages are generated during the initial build process. The pages are pre-rendered based on the defined templates and data sources, resulting in a complete set of static HTML files. On the other hand, ISR generates pages as requested, on demand. Initial static pages are still generated during the build process, but ISR allows updates and regeneration of specific pages without rebuilding the entire site.
ISR offers better scalability for websites with frequent content updates or large amounts of content. Since only specific pages are regenerated when necessary, the server load is reduced compared to rebuilding the entire site in SSG. It makes ISR suitable for websites that experience unpredictable traffic spikes or have high volumes of updates.
Below is a tabular comparison of the differences between Incremental Static Regeneration(ISR) and Static Site Generation(SSG):
Incremental Static Regeneration(ISR) | Static Site Generation(SSG) | |
---|---|---|
1 | Pages are generated as they are requested | All pages are generated during the building process |
2 | Allows for on-demand regeneration of specific pages | Requires a full rebuild to reflect any content changes |
3 | Balances static generation with dynamic content | Focuses on pre-rendering static pages without dynamic updates |
4 | Allows for real-time or near real-time updates through revalidation intervals | Does not offer real-time updates unless rebuilt |
5 | Reduces build time as only specific pages need regeneration | Longer build time due to generating all pages upfront |
6 | Provides a balance between performance and contents | Offers excellent performance for static pages |
7 | Scalable for websites with frequent content updates | Ideal for websites with static content and less frequent updates |
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
How Incremental Static Regeneration Works in Nextjs
To implement Incremental Static Regeneration in Next.js, you need to follow a few steps:
-
Specify the
revalidate
property: In your page component, you can define therevalidate
property to set the revalidation interval for that page. For example,revalidate: 60
would set a revalidation interval of 60 seconds. -
Generate static pages: Use Next.js’s static site generation capabilities to pre-render your pages during the build process. It can be done using functions like
getStaticProps
andgetStaticPaths
. -
Enable ISR: To enable Incremental Static Regeneration for a page, you must set the
fallback
property to eithertrue
orblocking
in thegetStaticPaths
function. It tells Next.js to generate the page at runtime if it hasn’t been pre-rendered yet or the revalidation interval has elapsed. -
Handle fallback rendering: When the page is requested and needs to be generated at runtime, Next.js provides fallback rendering. You can use this opportunity to display a loading state until the page is fully generated.
Let’s look at an example of how to use Incremental Static Regeneration (ISR) in Next.js to update a blog page whenever a new blog post is added.
Getting Started with your project
First, ensure you have Nodejs and npm installed on your machine. Then, create a new Nextjs project by running the following commands in your terminal:
npx create-next-app my-blog
cd my-blog
Create a directory called data
in the root of your project. Inside the data directory, create a file called blogPosts.json
. This file will serve as your blog post data source.
[
{
"id": "1",
"title": "First Blog Post",
"content": "This is my content for the first blog post."
},
{
"id": "2",
"title": "Second Blog Post",
"content": "This is my content for the second blog post."
}
]
Next, you create a new file called pages/blog/[id].js
inside the pages directory. This file will handle the routing for individual blog posts.
import { useRouter } from "next/router";
import { useEffect, useState } from "react";
const BlogPost = () => {
const router = useRouter();
const { id } = router.query;
const [post, setPost] = useState(null);
useEffect(() => {
const fetchPost = async () => {
const response = await fetch(`/api/blogPosts/${id}`);
const data = await response.json();
setPost(data);
};
fetchPost();
}, [id]);
if (!post) {
return <div>Loading...</div>;
}
return (
<div>
<h1>{post.title}</h1>
<p>{post.content}</p>
</div>
);
};
export default BlogPost;
In the code above, Nextjs’s routing by creating a file with square brackets [id].js
was used. It allows you to handle routes like /blog/1
, /blog/2
, etc. The useRouter
hook is used to access the id
parameter from the route. We also fetch the individual blog post data using the useEffect hook. Inside the fetchPost
function, make an API call to retrieve the specific blog post based on its ID.
Then you create a new file called pages/api/blogPosts/[id].js
. This file will serve as the API route to fetch individual blog posts.
import blogPosts from '../../../data/blogPosts.json';
export default (req, res) => {
const { id } = req.query;
const post = blogPosts.find((post) => post.id === id);
if (!post) {
res.status(404).json({ error: 'Blog post not found' });
} else {
res.status(200).json(post);
}
};
Here, the blogPosts
data from the blogPosts.json
file was imported. You extract the id
parameter from the request query inside the API route. Then you find the matching blog post based on the ID and send it back as a response.
Next, create a new file called pages/blog/index.js
. This file will serve as the blog index page that lists all the blog posts. We’ll use Incremental Static Regeneration to update this page when new blog posts are added.
import Link from "next/link";
import { useEffect, useState } from "react";
const BlogIndex = () => {
const [posts, setPosts] = useState([]);
useEffect(() => {
const fetchPosts = async () => {
const response = await fetch("/api/blogPosts");
const data = await response.json();
setPosts(data);
};
fetchPosts();
}, []);
return (
<div>
<h1>Blog Posts</h1>
<ul>
{posts.map((post) => (
<li key={post.id}>
<Link href={`/blog/${post.id}`}>
<a>{post.title}</a>
</Link>
</li>
))}
</ul>
</div>
);
};
In the code above, we fetch all the blog posts using the useEffect
hook. Inside the fetchPosts
function, we make an API call to retrieve all the blog posts from the API route /api/blogPosts
. We set the retrieved data in the posts
state. We then render a list of blog posts with links to their pages using the map
function. Each blog post item is wrapped in a Link
component from Nextjs, which provides client-side navigation without a full page reload.
You need to modify the getStaticProps
function in the pages/blog/index.js
file to enable Incremental Static Regeneration for the blog index page. This function will generate the initial static version of the page and update it incrementally when new blog posts are added.
Replace the existing BlogIndex
component code with the following:
import Link from 'next/link';
const BlogIndex = ({ posts }) => {
return (
<div>
<h1>Blog Posts</h1>
<ul>
{posts.map((post) => (
<li key={post.id}>
<Link href={`/blog/${post.id}`}>
<a>{post.title}</a>
</Link>
</li>
))}
</ul>
</div>
);
};
export async function getStaticProps() {
const response = await fetch('/api/blogPosts');
const data = await response.json();
return {
props: {
posts: data,
},
revalidate: 20, // Regenerate the page every 20 seconds
};
}
export default BlogIndex;
In the code above, we’ve replaced the useEffect
hook with the getStaticProps
function. This function is a Nextjs function that runs at build time and fetches the initial data for the page. Inside the getStaticProps
function, we make an API call to retrieve all the blog posts and set them as the posts
prop. We then return this prop as part of the props
object. The revalidate
option is set to 20, which means Nextjs will regenerate the page every 20 seconds. This allows the page to be incrementally updated with new blog posts without rebuilding the entire site.
To see the code in action, run the Nextjs development server using the following command:
npm run dev
This command will start the development server, and you should see an output indicating that the server is running. Open your web browser and visit http://localhost:3000/blog
. You should see the “Blog Posts” heading and a list of blog post titles.
To simulate adding a new blog post, let’s update the blogPosts.json file. Add a new blog post object to the array:
[
{
"id": "1",
"title": "First Blog Post",
"content": "This is my content for the first blog post."
},
{
"id": "2",
"title": "Second Blog Post",
"content": "This is my content for the second blog post."
},
{
"id": "3",
"title": "New Blog Post",
"content": "This is my content for the new blog post."
}
]
Don’t forget to save the files for changes to reflect.
After saving the blogPosts.json
file, wait for the page to refresh automatically. Nextjs will trigger Incremental Static Regeneration and update the blog index page with the new blog post without rebuilding the entire site.
Once the page refreshes, go back to http://localhost:3000/blog
. You should see the “New Blog Post” added to the list of blog posts without needing a full rebuild. Clicking on the new blog post should take you to its page.
That’s it! You’ve successfully implemented Incremental Static Regeneration in Nextjs to update the blog page without rebuilding the entire site when adding a new blog post.
Use Cases for Incremental Static Regeneration in Nextjs
Knowing when to use ISR depends on the specific requirement of your application. Here are some scenarios where developers can use ISR:
-
ISR can be a good choice if your website requires frequent updates to display the latest content. News websites, blogs, or e-commerce platforms that need to show real-time information or product availability can use ISR.
-
Applications such as stock market tickers, live chat, or social media feeds can benefit from ISR. By configuring appropriate revalidation intervals, you can ensure the data is updated regularly, providing users with the most recent information. For example, a weather forecasting website can utilize ISR to fetch real-time weather data and update the regular pages regularly.
-
If you want to conduct A/B testing on static pages, ISR can facilitate the process. By generating different page versions and leveraging ISR, you can serve alternative content to other users, measure their responses, and make data-driven decisions to improve user experiences.
-
Developers can use ISR in cases where data fetching or processing is resource-intensive, and ISR can help optimize performance. Instead of recalculating or fetching data for every request, you can generate pre-rendered pages using ISR. It will help reduce the load on the backend system.
Conclusion
Incremental Static Regeneration in Nextjs offers numerous benefits, and it also has its challenges. If you’re looking for a way to create static pages that can be updated without redeploying your entire site, then ISR is an excellent option. Happy Coding!