Routing in Next.js
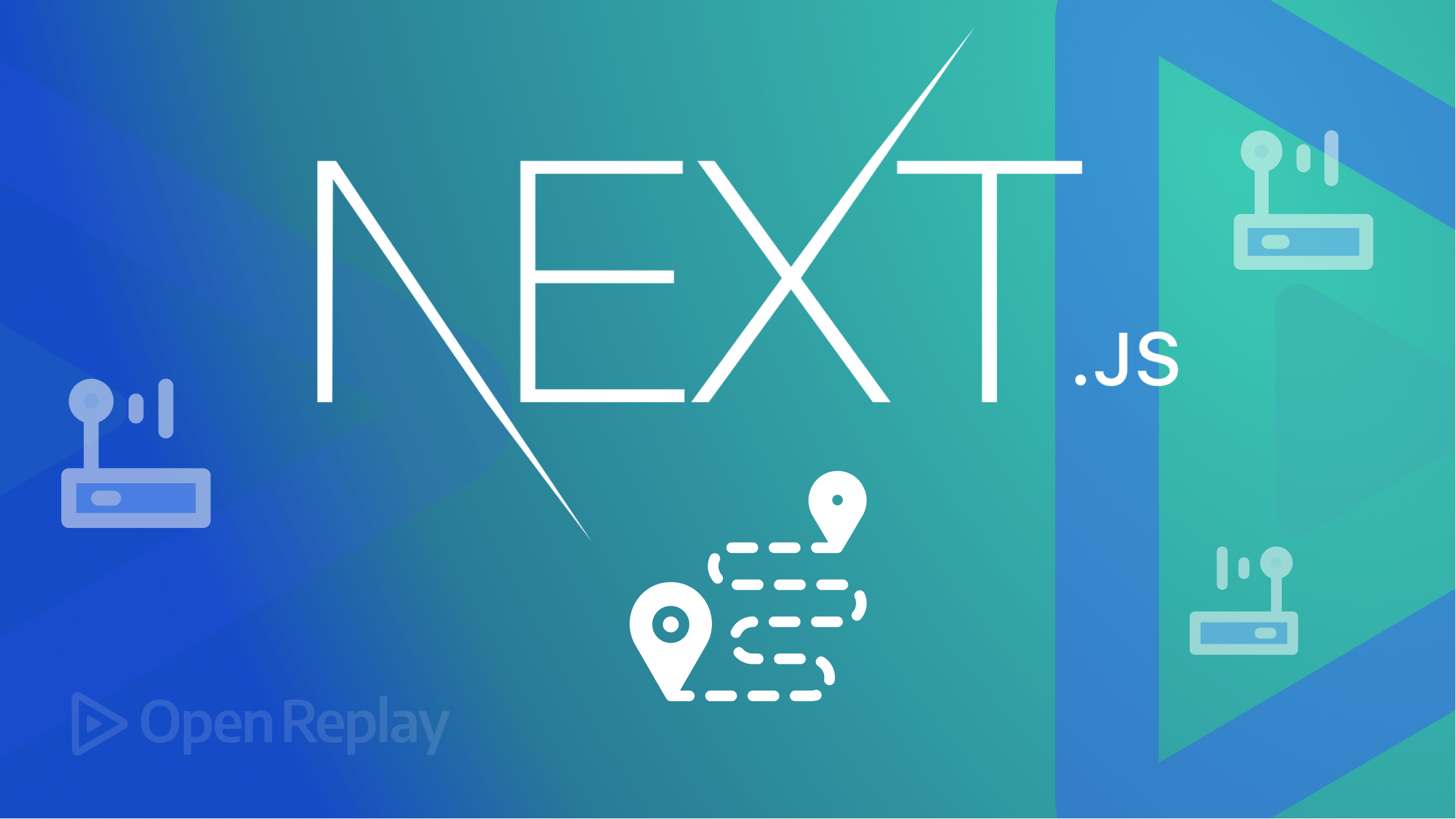
When we build a project in Next.js, we use the file components structure, such as the pages folder and API folder, and sometimes we also create a component folder. Each folder is a container for the files, which contain the code for each component of our page, the JSON data we want to pass to our pages, and many other reusable components.
When using these files, components, or pages, we do what is known as routing in Next.js in other to pass data and information and link pages or components to one another. Creating routes in Next.js is simple but can also become very complex, especially when nesting files or folders in other folders. It can also become complex when the need arises to make dynamic routes for more dynamic pages. Before going ahead, let us understand what routing is in Next.js.
What is routing?
Routing means navigating from one page to another. Yes, that’s what routing is. But when navigating from page to page, data is undoubtedly being passed as well, most times but not always. For example, if you are building a company website to just talk about the company and its services, it will have a home page, about page, services page, possibly a staff page, and others. This website will have a navigation bar or panel, and that navigation panel will be used to navigate through the website.
Now let us imagine a different web app or website, like an e-commerce store with various products. The web app will have a home page listing all the goods or products they sell, but that page won’t describe the products in detail. To work on this, we have to create another page that describes each product which means we then have to navigate to the product description page not through a navigation bar but directly by clicking the product itself.
In the two examples, we use routes to navigate through the pages. But the first example uses a navigation bar or panel, while the second passes data to another page, more like a dynamic page. Let us also get a look at how file systems work in Next.js.
How does the Next.js file system work?
In Next.js, there are two file routing structures with which we work with which are;
- API routes
- Pages Routes
API routes
An API route is made in a folder with the name api
in the Next.js app. When files are saved into this folder, it is automatically associated with the API route directory in Next.js. This route serves as an endpoint for any API file.
Pages routes
A page route is set in the pages
****directory in the Next.js app. Files saved in this directory are displayed as web pages through this directory route.
Next.js has a built-in route system whereby you don’t need to specify any route or URL. All you need to do is create a file and put them in any of the two routes so Next.js can tell what each file is for and how it will be routed.
Routing in Next.js
Now we know that pages or files are routed based on the two-file route system in Next.js, let us learn about the different types of routes when using the file system. When working on our Next.js app, we have different ways to structure or page routes based on the project they are;
- Index routing
- Nested routing, and
- Dynamic routing
Index routes
These are the most basic routes created in the pages
root folder with the name index.js
. They can end in index.tsx
if it’s TypeScript.
The example below shows what the folder structure looks like.
├── pages
│ ├── index.js
Nested routes
This type of page route is simple and used when we have a particular folder containing several linked pages. This type of route is created by creating a folder in the pages and then the files in it. Example:
├── pages
│ ├── blog
│ │ ├── index.js
│ │ ├── first.js
│ │ ├── second.js
In the example, we have a folder called blog
, which contains three files. Whenever we type in the URL http://localhost:3000/pages/blog
it shows the page index.js
because using the file name index.js
automatically tells Next.js that this is the root file.
We can even access the blog pages as http://localhost:3000/blog
it will still work as long as the blog folder exists. We use /blog/first
or /blog/second
to access the other pages.
The blog folder can even have another folder in it with its pages. This is an example of a nested page route.
Dynamic routes
What if our blog or product page has 100 items or posts? Do we then create 100 pages that are nested? No, this is where dynamic routes come into play. Example
├── pages
│ ├── blog
│ │ ├── [id].js
│ │ ├── index.js
This type of route has the index.js
file and a special file with its name enclosed in a square bracket; this file is called a dynamic file. We use the URL http://localhost:3000/blog/[id]
to access this file. The ID of the item or post will be inserted at the position of [id], for example;
http://localhost:3000/blog/1
to indicate the first item or post to be displayed on a page.
This is how the dynamic route works.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
Routing components in Next.js
Now let us look at some code examples to perform routing. In Next.js, there we can perform routing using the following;
- Link component
- Dynamic routing
Link components
This component is one of the ways to perform routing, which Next.js made. In other to do so,
- In the
pages
folder, create a file called index.js. If there is one already, delete the contents of the file or delete the file and create a new one. - Create two more files called home.js and about.js. The folder structure will be like this;
├── pages
│ ├── about.js
│ ├── home.js
│ ├── index.js
- Next, in the index file, import the Link component.
import Link from 'next/link'
- In the
return
function, write in the Link component; in it, write the text you want to use as navigation wrapped in the anchor tags. It could also be a button wrapped in the anchor tag.
import Link from 'next/link'
const index = () => {
return (
<div>
<Link href={"./content"}>
<a>Home</a>
<a>about</a>
</Link>
<div> Hello index page </div>
)
}
export default index
- In the home file copy write the following;
import Link from 'next/link'
const home = () => {
return (
<div>
<Link href={"./content"}>
<a>index</a>
<a>about</a>
</Link>
<div> Hello home page </div>
)
}
export default home
- In the about file, write the following code;
import Link from 'next/link'
const about = () => {
return (
<div>
<Link href={"./content"}>
<a>index</a>
<a>home</a>
</Link>
<div> Hello home page </div>
)
}
export default about
After writing the code for each file, run the URL http://localhost:3000/pages,
and it should show the links you can use to route or navigate through each page.
Dynamic routing
Now let us take a look at how dynamic routing works in Next.js. To do so, we will use the useRouter
hook.
- First, in your pages folder, create another folder. I named my folder blog.
- Next, create two files in the folder, one called first.js and another called second.js.
- Let us import the Link component from Next.js, create a component with the name Route and pass a property into it.
import Link from 'next/link'
const Route = props => (
<p>
<Link href={`/blog/second?title=${props.title}`}>
{props.title}
</Link>
</p>
)
- In the page component, we will create a list with the Route component.
const first = () => {
return(
<div>
<h1>Home Page</h1>
<Route title='title 1'/>
<Route title='title 2'/>
</div>
)
}
export default first
- Next, in the
second.js
file, we will use theuseRouter
hook to display the details in the title from the first page to the second page.
import { useRouter } from "next/router";
const second = () => {
const router= useRouter();
return(
<div>
<h1>{router.query.title}</h1>
</div>
)
}
export default second
The screencast below it shows what it looks like when run.
There are other ways to use dynamic routes in Next.js, but they involve getting data from a different source using getStaticProps
and getStaticPaths
, which is not the scope of this article.
Conclusion
In this article, we looked at what routing is, how the Next.js file system works and how to route or navigate pages using the Link
component and dynamic routing using the useRouter
hook provided by Next.js.
Note: Using routes in Next.js is pretty simple, but you also have to note how the file structure works because it’s based on the file structure Next.js performs its route between pages.
A TIP FROM THE EDITOR: We have other interesting articles on Next.js, like State Management In Next.Js With Redux Toolkit, SEO Tips For Next.Js Sites, and How To Deploy A Next.Js App To Production.