Securing React Apps: A Guide to Preventing Cross-Site Scripting with DOMPurify
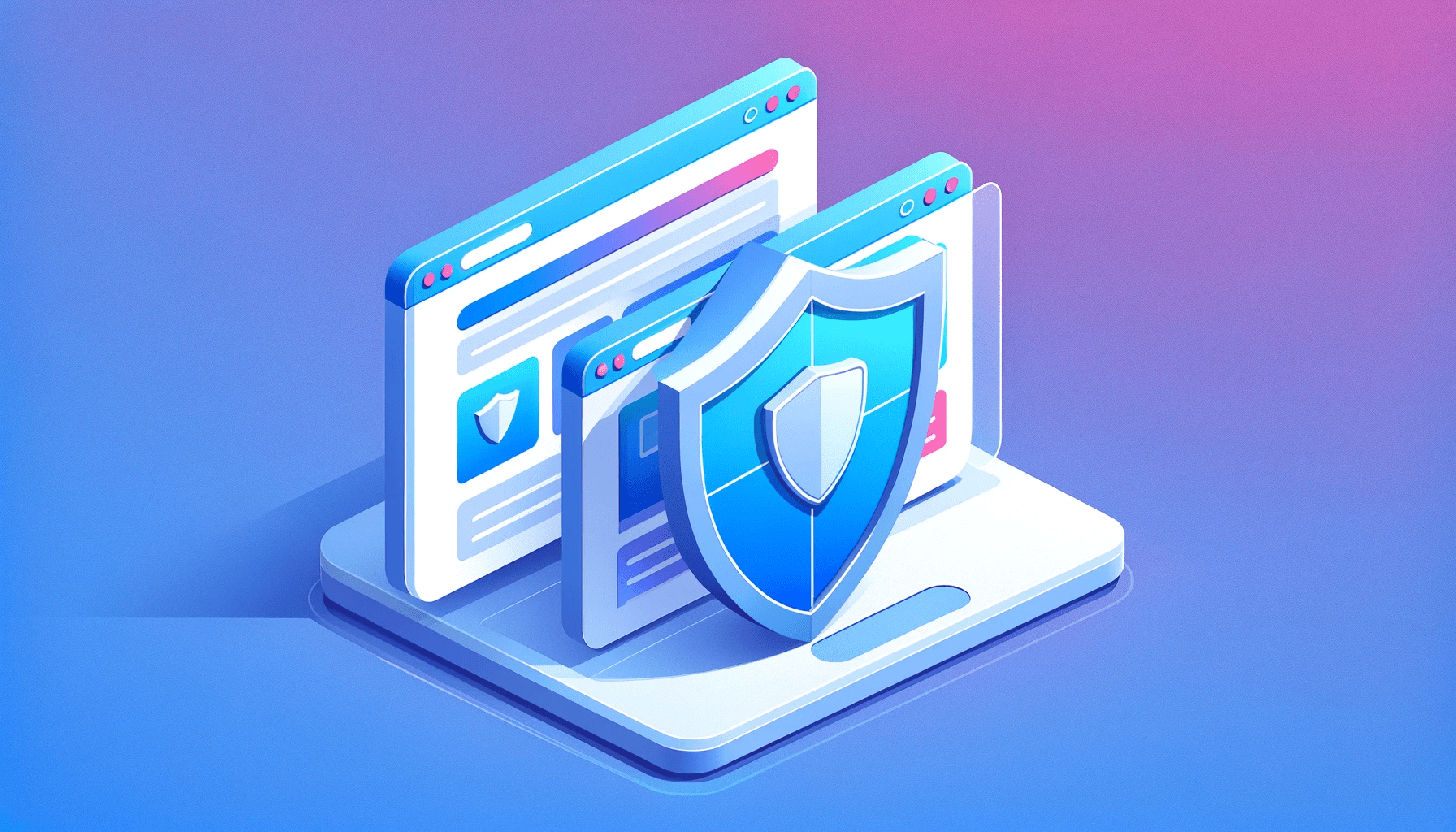
Discover how at OpenReplay.com.
In the dynamic world of web development, where React.js orchestrates seamless user experiences, Cross-Site Scripting (XSS) vulnerabilities threaten innovation, casting a shadow on security. As our digital footprint expands, the significance of online security amplifies. XSS, a technique used by attackers to inject malicious scripts into websites, poses a formidable risk to the integrity of web applications. Developers face a tough task, needing to innovate while also protecting against sneaky attacks.
In the quest for cyber fortification, DOMPurify emerges as a strong defender. This article delves into the intricacies of securing React applications against XSS attacks and explores how DOMPurify
, a potent sanitization tool, stands as a defense against the relentless tide of malicious script injections. Join us in this exploration of security, where we unravel the nuances of XSS vulnerabilities, examine React’s susceptibility to these threats, and equip ourselves with the knowledge and implementation strategies needed to fortify React applications with DOMPurify’s protective shield.
Understanding Cross-Site Scripting (XSS)
XSS fundamentally entails injecting unauthorized scripts into a web application. These injected scripts execute within the user’s browser, compromising the application’s security. XSS can lead to the theft of sensitive user data and website defacement. A thorough understanding of XSS is crucial for developing effective countermeasures. To better understand XSS, let’s consider the different types of XSS.
Reflected XSS
Reflected XSS occurs when user input is immediately reflected back to the browser. This often transpires through URLs or form fields, and the injected script is executed in real time. Attackers typically craft phishing links or exploit vulnerabilities in input validation to carry out these attacks. Let’s consider search functionality on a website where the search query is reflected in the response without proper validation. Suppose the website handles a search query like this in its backend code:
// This is a simplified example for illustration purposes
app.get('/search', (req, res) => {
const searchTerm = req.query.q; // Assuming the search query is provided in the URL query parameters
//Responding with search results in JSON format
const searchResults = {
searchTerm: searchTerm,
results: [
"Result 1",
"Result 2"
// ...
]
};
res.json(searchResults);
});
On the client side, a vulnerability is created if the user’s input is directly used in the HTML without proper escaping.
Suppose an attacker crafts a malicious search term with a script payload:
https://example.com/search?q=<script>alert('XSS');</script>
When a user visits this URL, the server responds with JSON data:
{
"searchTerm": "<script>alert('XSS');</script>",
"results": [
"Result 1",
"Result 2"
// ...
]
}
The Client then renders the HTML without properly escaping the searchTerm
:
<div>
<h1>Search Results for: <script>alert('XSS');</script></h1>
<ul>
<li>Result 1</li>
<li>Result 2</li>
<!-- ... -->
</ul>
</div>
This introduces a reflected XSS vulnerability, as the script is executed in the context of users viewing the search results, which show them information from the attacker.
Stored XSS
In the realm of stored XSS, malevolent scripts are injected into a web application and persistently stored on a server. These scripts affect all users who access a particular page or view the compromised content, making them a potent vector for spreading malware and compromising user data. Let’s consider a different scenario where a website allows users to create and customize their profiles, including a profile description.
Suppose the website handles profile updates like this in its backend code:
// This is a simplified example for illustration purposes
app.post('/update_profile', (req, res) => {
const userProfileDescription = req.body.description; // Assuming description is sent in the request body
// Save the profile description to a database
// Insecure implementation: No validation or sanitization
saveProfileDescriptionToDatabase(userProfileDescription);
res.send('Profile updated successfully!');
});
// Function to save profile description to the database (insecure implementation)
function saveProfileDescriptionToDatabase(description) {
// Insecure: Saving user input directly without proper validation or sanitization
db.save({ description: description });
}
Now, let’s consider an example of a malicious profile description that includes a stored XSS payload:
<script>
// Malicious JavaScript code here, e.g., redirecting the user to an attacker-controlled site
window.location.href = 'https://attacker.com';
</script>
When a user updates their profile with this malicious description, the website stores the entire payload in the database without proper validation or sanitization. Later, when another user views the profile page, the malicious script is executed in their browser.:
<!-- Displayed on the user's profile page -->
<div>
<h1>User123's Profile</h1>
<p>Description: </p>
<script>
// Malicious JavaScript code here
window.location.href = 'https://attacker.com';
</script>
</div>
In this case, the attacker could use the stored XSS vulnerability to perform actions like redirecting users who view the affected profile to a phishing site.
DOM-based XSS
DOM-based XSS exploits vulnerabilities in the Document Object Model (DOM) of a web page. Attackers manipulate the structure and behavior of the DOM, often targeting client-side scripts that dynamically modify the content. This form of XSS is particularly elusive, requiring a nuanced understanding of the application’s frontend architecture. Let’s consider a scenario where a client is vulnerable to DOM-based XSS due to improper handling of user input. In this example, the vulnerability arises from a situation where user input is directly incorporated into the client and rendered on the page without proper sanitization.
Suppose the Client looks like this:
<body>
<div class="user-profile-container">
<h1>User Profile</h1>
<p>Bio: <span id="userBio"></span></p>
<!-- ... other profile information ... -->
<textarea
id="bioInput"
placeholder="Enter your bio..."
oninput="handleBioChange()"
></textarea>
</div>
<script>
// Insecure implementation: User input is directly incorporated into the JavaScript code
let userBio = '';
function handleBioChange() {
// Insecure: User input is directly used without proper validation or sanitization
userBio = document.getElementById('bioInput').value;
document.getElementById('userBio').textContent = userBio;
}
</script>
</body>
Now, let’s consider a scenario where an attacker tricks a user into visiting a specially crafted URL that includes a malicious payload:
https://example.com/user-profile?bio=<img src=x onerror="alert('XSS Attack!')" />
If the client does not properly validate or sanitize the bio parameter from the URL, the malicious payload will be directly rendered in the component, leading to a DOM-based XSS vulnerability. The rendered HTML might look like this:
<div>
<h1>User Profile</h1>
<p>Bio: <img src=x onerror="alert('XSS Attack!')" /></p>
<!-- ... other profile information ... -->
<textarea
placeholder="Enter your bio..."
value="<img src=x onerror="alert('XSS Attack!')" />"
onChange={handleBioChange}
/>
</div>
In this example, the attacker injects an <img>
tag with an onerror attribute containing JavaScript code. The JavaScript code is executed when the client renders this content, leading to an XSS attack.
XSS attacks leverage diverse vectors, each with its own set of characteristics and potential risks. Attackers may inject malicious code through input fields, manipulate URL parameters, or exploit vulnerabilities in third-party scripts integrated into the web application. The consequences span a wide spectrum, from compromising user data and hijacking sessions to injecting harmful content that becomes visible to other users.
XSS in React
React is not XSS foolproof, but React does several things under the hood to protect React applications from XSS attacks, one of which is escaping by default. When rendering dynamic content using curly braces {}
. React escapes the content, treating it as plain text rather than HTML. This default behavior helps prevent the injection of malicious scripts into the DOM. This works for inputs from users and URL parameters. Consider a basic React app that takes in a user’s input and displays it;
import React, { useState } from 'react';
function App() {
// State to store user input
const [inputValue, setInputValue] = useState('');
// State to store the validated message
const [validatedMessage, setValidatedMessage] = useState('');
// Function to handle input change
const handleInputChange = (event) => {
setInputValue(event.target.value);
};
// Function to handle form submission
const handleSubmit = (event) => {
event.preventDefault();
// Validate the input (you can replace this with your own validation logic)
if (inputValue.trim() === '') {
setValidatedMessage('Input cannot be empty');
} else {
setValidatedMessage(`Valid input: ${inputValue}`);
}
};
return (
<div>
<form onSubmit={handleSubmit}>
<label>
Input:
<input type="text" value={inputValue} onChange={handleInputChange} />
</label>
<button type="submit">Submit</button>
</form>
<p>{validatedMessage}</p>
</div>
);
}
export default App;
If an attacker should input a script in the input box, React treats it as a string, not as HTML. Here’s an example:
This prevents the execution of JavaScript within the <script>
, preventing any potential DOM-based or reflective XSS attack. This protective measure shields your application from attackers attempting to execute malicious code.
Rendering HTML Elements Dynamically in React
Now let’s consider a situation where you’re building a blog, and you’d want to render HTML elements directly to preserve the format of your writeups. If I input this:
<h3>Explore the Latest Blog Post</h3>
<p>Discover engaging content in this blog. Experience a mix of <strong>bold</strong> elements and <em>italic</em> elements throughout the text!</p>
React escapes the input by default, so we’ll get the following;
This secures the React application from XSS attacks but ruins the user experience. As such, you’ll need to display the input as a markup instead of rendering it as a string.
To do this, we’ll use the prop dangerouslySetInnerHTML
. This allows us to display the input as a markup; it takes in a key _html
whose value is the HTML markup you wish to render inside the container, in our case, validatedMessage
.
return (
<div >
<form onSubmit={handleSubmit} >
<label>
Input:
<input type="text" value={inputValue} onChange={handleInputChange} />
</label>
<button type="submit">Submit</button>
</form>
<div dangerouslySetInnerHTML={{__html:validatedMessage}}>
</div>
</div>
);
}
export default App;
With this done, we’ll get this;
The image above shows that we inputted markup into the input field instead of rendering it as a string. React renders it as a markup, preserving its format.
This improvement now opens up our application to XSS attacks;
From the image above, we can see that if the attacker inputs a script in our input box, it is executed by our React application. To deal with this, we’ll make use of the package DOMPurify.
DOMPurify: A Powerful XSS Defense Mechanism
DOMPurify
is an open-source JavaScript library designed to sanitize and clean HTML content, making it safe for rendering in the Document Object Model (DOM). Developed specifically to mitigate XSS risks, DOMPurify
acts as a stringent gatekeeper, meticulously filtering out any potentially harmful elements and attributes from user-generated or dynamic content. DOMPurify
ensures that user-generated content is thoroughly sanitized, removing any embedded scripts and potential XSS threats before rendering. By purifying the DOM, DOMPurify
helps maintain the integrity of the Document Object Model, ensuring that dynamic content additions do not compromise the security of the application. DOMPurify
is crafted with compatibility in mind, seamlessly integrating into React applications to provide a robust defense against XSS vulnerabilities.
How Does DOMPurify Work?
- HTML Sanitization:
DOMPurify
employs a robust HTML sanitization process, carefully parsing and validating HTML content to remove any elements or attributes that could pose a security risk. - Whitelist-Based Filtering: Instead of adopting a blacklist approach, which identifies and blocks known malicious patterns,
DOMPurify
employs a whitelist-based strategy. It allows only specified, safe elements and attributes to pass through, providing a more proactive and resilient defense. - Context-Aware Filtering:
DOMPurify
is context-aware, adapting its filtering rules based on the specific context in which the HTML content is used. This ensures that the sanitization process is tailored to the requirements of different DOM contexts.
Integrating DOMPurify into React Apps
Securing React applications against Cross-Site Scripting (XSS) vulnerabilities involves seamlessly integrating DOMPurify
into the development workflow. Here’s a step-by-step guide to installing and utilizing DOMPurify
in a React project:
- Install DOMPurify via npm
Open your terminal and navigate to your React project. Install DOMPurify
using npm:
npm install dompurify
- Import DOMPurify in your React component
In the React component where you want to sanitize user-generated content, import DOMPurify
:
import DOMPurify from 'dompurify';
- Use DOMPurify to sanitize HTML content
Suppose you have a React component that receives HTML content, such as a comment or a dynamic piece of data. Before rendering it, pass the content through DOMPurify
:
import React, { useState } from "react";
import DOMPurify from "dompurify";
function App() {
// State to store user input
const [inputValue, setInputValue] = useState("");
// State to store the validated message
const [validatedMessage, setValidatedMessage] = useState("");
// Function to handle input change
const handleInputChange = (event) => {
setInputValue(event.target.value);
};
// Function to handle form submission
const handleSubmit = (event) => {
event.preventDefault();
// Validate the input (you can replace this with your own validation logic)
if (inputValue.trim() === "") {
setValidatedMessage("Input cannot be empty");
} else {
setValidatedMessage(`Valid input: ${inputValue}`);
}
};
const sanitizedBlog = DOMPurify.sanitize(validatedMessage);
return (
<div>
<form onSubmit={handleSubmit}>
<label>
Input:
<input type="text" value={inputValue} onChange={handleInputChange} />
</label>
<button type="submit">Submit</button>
</form>
<div dangerouslySetInnerHTML={{ __html: sanitizedBlog }}></div>
</div>
);
}
export default App;
Using dangerouslySetInnerHTML
, React allows rendering HTML content, and DOMPurify
ensures that it is thoroughly sanitized before being injected into the DOM
. With this done, React will render our inputs as markups, while DOMPurify
will sanitize the markup to ensure scripts aren’t executed.
Configuring DOMPurify for specific use cases and requirements
While DOMPurify
is effective right out of the box, it also offers configuration options to tailor its behavior to specific use cases. Here’s how you can configure DOMPurify
in a React project:
Whitelist Specific Elements and Attributes
DOMPurify employs a stringent approach to sanitizing HTML content by default, aiming to filter out known malicious patterns and elements. These patterns often include scripting elements and attributes commonly associated with Cross-Site Scripting (XSS) attacks, such as <script>
tags and event handlers like onload
or onclick
.
Whitelisting, as opposed to blacklisting, takes a proactive stance. Instead of maintaining an exhaustive list of forbidden elements, developers selectively permit only the elements and attributes deemed safe for the application’s context.
DOMPurify.addHook('uponSanitizeElement', (node, data) => {
// Allow specific elements
if (data.tagName === 'a') {
// Whitelist attributes for the 'a' element
data.allowedAttributes.href = true;
}
});
In this example, we whitelist the a
(anchor) element. The focus is on the ‘a’ (anchor) element, a common source of potential security threats. By explicitly allowing the href
attribute for the a
element, developers are exercising fine-grained control over the permissions granted to this element during the sanitization process. This selective approach ensures that only the necessary attributes are permitted, mitigating the risk of unintended security loopholes and enhancing the overall security posture of the application and explicitly allowing the href
attribute.
Customize URI Schemes
DOMPurify, by default, allows a broad range of URI schemes to accommodate diverse use cases. However, developers may need to customize URI schemes to enhance security by explicitly allowing or restricting certain links.
DOMPurify.addHook('beforeSanitizeAttributes', (node) => {
if (node.tagName === 'a' && node.getAttribute('href')) {
// Allow only specific URI schemes
const uri = DOMPurify.sanitizeURL(node.getAttribute('href'));
if (!uri.startsWith('http://') && !uri.startsWith('https://')) {
node.removeAttribute('href');
}
}
});
In the example, a hook is employed to customize URI schemes for anchor (<a>
) elements. It checks the href
attribute and allows only URIs that start with http://
or https://
. This customization ensures that only links with secure and well-defined schemes are permitted, mitigating the risk of potentially malicious links.
You can learn more here
Best practices for incorporating DOMPurify into React components
To ensure the smooth integration and optimal performance of DOMPurify
in React components, consider the following best practices:
- Centralized Configuration: Maintain a centralized configuration for
DOMPurify
to ensure consistency across the application. - Use Hooks or Higher-Order Components: Leverage React hooks or higher-order components to encapsulate the sanitization logic, promoting reusability.
Suppose you have a
UserContent
component that renders user-generated HTML content. Here’s how you can implement a custom hook for sanitization:
import React, { useState, useEffect } from "react";
import DOMPurify from "dompurify";
// Custom hook for sanitizing HTML content
const useSanitizeContent = (initialContent) => {
const [sanitizedContent, setSanitizedContent] = useState(initialContent);
useEffect(() => {
const sanitized = DOMPurify.sanitize(initialContent);
setSanitizedContent(sanitized);
}, [initialContent]);
return sanitizedContent;
};
// Example component using the custom hook
const UserContent = ({ content }) => {
const sanitizedContent = useSanitizeContent(content);
return <div dangerouslySetInnerHTML={{ __html: sanitizedContent }} />;
};
// Usage of the UserContent component
const App = () => {
const userContent =
'<p>Hello, <a href="#" onclick="alert(\'XSS Attack!\')">click me</a></p>';
return (
<div>
<h1>User Content</h1>
<UserContent content={userContent} />
</div>
);
};
export default App;
By encapsulating the sanitization logic within a custom hook, you promote reusability and maintain a clear separation of concerns. This approach allows you to easily apply the same sanitization logic across multiple components in your React application.
- Test Extensively: Perform thorough testing of your React components, especially those handling user-generated content, to validate the effectiveness of
DOMPurify
. - Regular Updates: Keep
DOMPurify
and other dependencies up to date to benefit from the latest security patches and improvements.
Certainly! Let’s explore the section on additional security measures for React applications:
Additional Security Measures
While DOMPurify
provides robust protection against Cross-Site Scripting (XSS) vulnerabilities in React applications, a comprehensive security strategy involves implementing additional measures. Safeguarding your application’s integrity requires a multifaceted approach that extends beyond client-side sanitization. Here are additional security measures to fortify your React app:
Content Security Policy (CSP) considerations for React apps
Content Security Policy (CSP) is a crucial defense mechanism that helps prevent different types of attacks, including XSS. Implementing a well-defined CSP
involves specifying which sources of content are considered trustworthy and which are not. In a React application, you can set up a CSP
header to control the execution of scripts and other resources. Here is an example of CSP Headers;
<meta http-equiv="Content-Security-Policy" content="default-src 'self'; script-src 'self' https://apis.example.com; style-src 'self' https://fonts.googleapis.com; img-src 'self' data:">
In this example:
default-src 'self'
: Specifies that the default source for content (except scripts, styles, and images) is the same origin.script-src 'self' https://apis.example.com
: Allows scripts only from the same origin and fromhttps://apis.example.com
.style-src 'self' https://fonts.googleapis.com
: Permits styles from the same origin and fromhttps://fonts.googleapis.com
.img-src 'self' data:
: Permits images from the same origin and data URIs.
Carefully tailor your CSP
based on the specific requirements of your React application to strike a balance between security and functionality. You can learn more here
Server-side validation and security measures
While client-side validation and sanitization are crucial, relying solely on them leaves your React app susceptible to attacks if malicious data reaches the server. Implement robust server-side validation to verify the integrity of incoming data and ensure that it adheres to expected formats and constraints. This includes validating user input, form submissions, and any data sent to the server.
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
// Enable JSON parsing middleware
app.use(bodyParser.json());
// Example route with server-side validation
app.post('/submit-form', (req, res) => {
const { username, email, message } = req.body;
// Server-side validation
if (!username || !email || !message) {
return res.status(400).json({ error: 'Incomplete form data' });
}
// Process the form data
// ...
return res.status(200).json({ success: true });
});
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server running on port ${PORT}`);
});
Example Server-side Validation (Node.js with Express)
Ensure that your server is configured securely, follows best practices, and receives regular security updates.
Ongoing monitoring and testing for XSS vulnerabilities
Security is an ongoing process, and regular monitoring and testing are essential components of a robust security strategy. Conduct regular security audits, penetration testing, and code reviews to identify and address potential vulnerabilities. Stay informed about the latest security threats and updates to the tools and libraries used in your React application.
-
Automated Security Testing: Integrate automated security testing tools into your development workflow to catch potential vulnerabilities early in the development process.
-
Manual Security Audits: Periodically, perform manual security audits to complement automated testing. Manual audits allow for a deeper analysis of complex security issues and ensure a comprehensive understanding of your application’s security landscape.
By incorporating these additional security measures, you create a robust defense perimeter for your React application, addressing potential vulnerabilities at various layers of the development stack.
Conclusion
In the realm of web development, securing React applications against XSS vulnerabilities demands a comprehensive strategy. DOMPurify
, our formidable defense mechanism, stands guard, ensuring user inputs are sanitized and malicious scripts are thwarted.
However, the battle extends beyond DOMPurify
. Content Security Policy (CSP), robust server-side validation, and ongoing testing are essential pillars of a resilient security posture.
As we conclude, remember that security is an ongoing effort. Regular updates, adherence to best practices, and vigilance against emerging threats are vital. In your journey, empower yourself with knowledge, leverage tools like DOMPurify
, and foster a security-first mindset.