Using Prettier with VSCode to write JavaScript
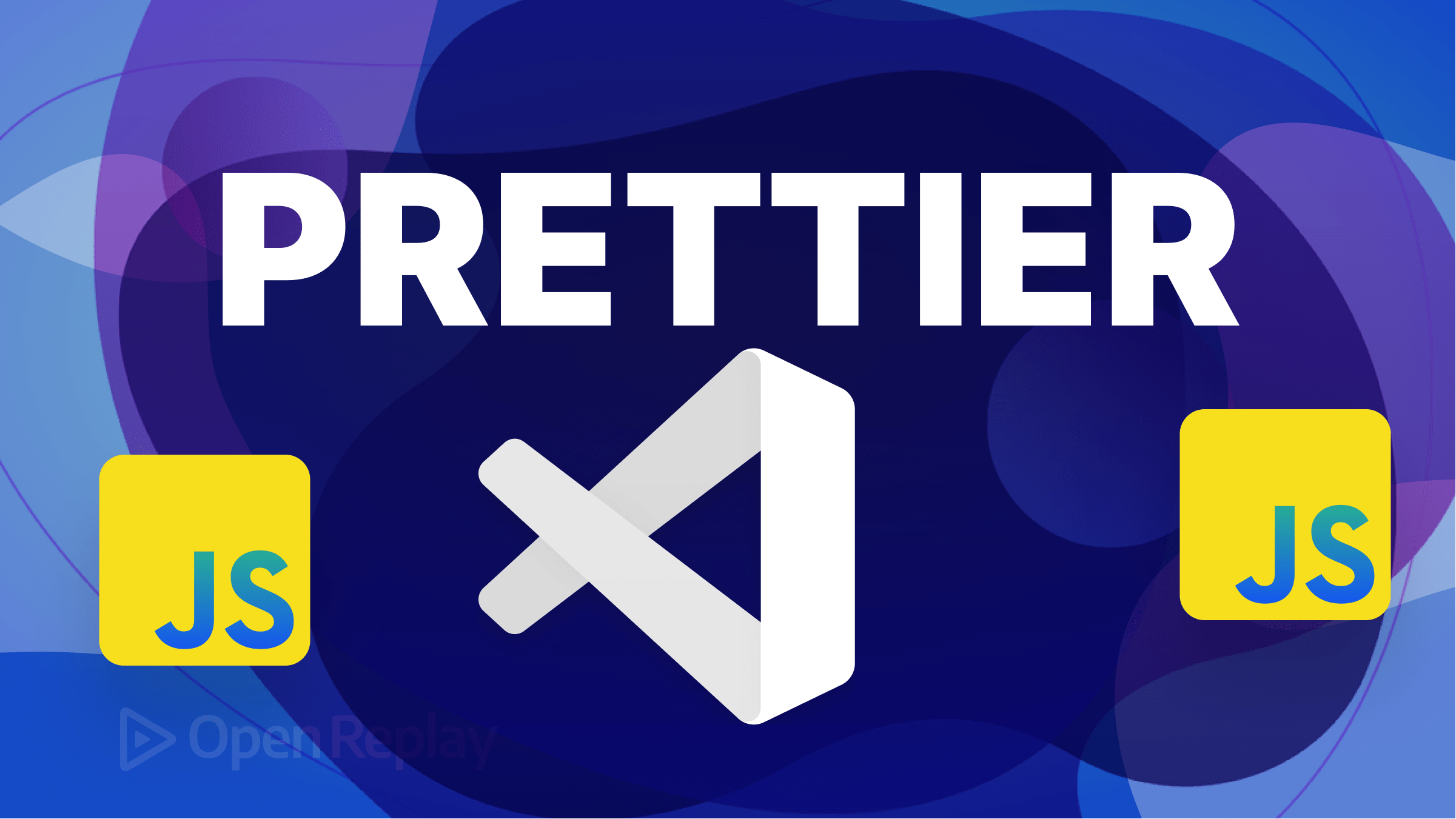
Prettier is an open-source code formatting tool that automatically formats your code to a consistent and organized style. It supports several programming languages, including JavaScript, TypeScript, HTML, CSS, and more. This article will explore using it with VS Code to write clean and organized code.
Why should you use Prettier to format your code?
-
Consistency: It ensures that your code is formatted consistently across your project, regardless of who wrote it. This can make your codebase more readable and maintainable.
-
Time-saving: Manually formatting code can be time-consuming, especially for larger codebases. It saves you time by formatting your code automatically.
-
Error reduction: Inconsistent code formatting can sometimes lead to errors or bugs. You can reduce the risk of such errors and save time on debugging.
-
Customizable: It is highly customizable and allows you to configure the formatting rules according to your project’s specific requirements.
-
Integration: It can be integrated into your code editor or build process, making it easy to incorporate into your workflow.
Installing Prettier in VSCode
You can install Prettier in VSCode by following these steps:
Open Visual Studio Code and navigate to the extensions view by clicking the Extensions icon in the left-hand side menu (or by pressing Ctrl+Shift+X
on Windows/Linux
or Cmd+Shift+X
on Mac).
Search for Prettier - Code formatter” in the search bar and click the “Install” button next to the extension.
After installation, you must configure it to format your code according to your desired settings. Add a .prettierrc
file to your project’s root directory or configure Prettier in your VSCode settings.
To format your code using Prettier, you can either use the “Format Document” command (Shift+Alt+F on Windows/Linux or Shift+Option+F on Mac) or set up Prettier to automatically format your code on save by adding the following line to your VSCode settings:
"editor.formatOnSave": true,
This will ensure your code is automatically formatted whenever you save your file.
That’s it! After installing and configuring it in VSCode, you can easily format your code to maintain consistency and readability throughout your project.
Customizing Prettier settings in VSCode
To customize Prettier settings in VSCode, you can use a .prettierrc
file or configure Prettier in your VSCode settings.
Here’s how to use a .prettierrc
file:
Create a new file called .prettierrc
in your project’s root directory.
Add your desired configuration options in JSON format to this file. For example:
{
"printWidth": 100,
"tabWidth": 2,
"useTabs": false,
"semi": true,
"singleQuote": true,
"trailingComma": "es5"
}
This configuration specifies a maximum line length of 100 characters, a tab width of 2 spaces, using spaces instead of tabs, semicolons at the end of statements, single quotes instead of double quotes, and a trailing comma where applicable.
Save the file.
Alternatively, you can configure it in your VSCode settings by following these steps:
Open your VSCode user settings by clicking “Preferences: Open User Settings” in the Command Palette (Ctrl+Shift+P on Windows/Linux or Cmd+Shift+P on Mac).
In the settings editor, search for Prettier and click Edit in settings.json
.
Add your desired configuration options in JSON
format to this file. For example:
{
"prettier.printWidth": 100,
"prettier.tabWidth": 2,
"prettier.useTabs": false,
"prettier.semi": true,
"prettier.singleQuote": true,
"prettier.trailingComma": "es5"
}
This configuration resembles the one in the .prettierrc
file above.
Save the file.
That’s it! You can now format your code according to your specific requirements with customized settings.
Formatting your JavaScript code with Prettier in VSCode
To format your JavaScript
code with Prettier in VSCode, use the “Format Document” command (Shift+Alt+F on Windows/Linux or Shift+Option+F on Mac) or set it up to format your code on save automatically.
Here’s how to format your JavaScript code with Prettier using the Format Document
command:
Open the JavaScript file you want to format in VSCode, press Shift+Alt+F on Windows/Linux or Shift+Option+F on Mac, or right-click in the editor and select Format Document
.
VSCode will format your code according to your preferred configuration settings.
If you want to automatically format your JavaScript code on save using Prettier, you can just open your VSCode user settings by clicking “Preferences: Open User Settings” in the Command Palette (Ctrl+Shift+P on Windows/Linux or Cmd+Shift+P on Mac). Then, search for “Editor: Format On Save” in the settings editor and check the box to enable this option.
Next, search for Prettier: Config Path” and set the path to your .prettierrc file
(if you have one). For example:
"prettier.configPath": "/path/to/your/project/.prettierrc"
Save the file.
Whenever you save your JavaScript file, Prettier will automatically format it according to your configured settings. This can save you time and help ensure your code is consistently formatted throughout your project.
Examples of Before/After Code
Here’s an example of before and after using Prettier to format a block of JavaScript code:
Before:
function myFunction() {
var x = document.getElementById("demo");
if (x.style.display === "none") {
x.style.display = "block";
} else {
x.style.display = "none";
}
}
After:
function myFunction() {
var x = document.getElementById("demo");
if (x.style.display === "none") {
x.style.display = "block";
} else {
x.style.display = "none";
}
}
Before:
const a=1;
const b=2;
if(a===1){
console.log("a is equal to 1");
}else{
console.log("a is not equal to 1");
}
After:
const a = 1;
const b = 2;
if (a === 1) {
console.log("a is equal to 1");
} else {
console.log("a is not equal to 1");
}
Here’s a larger example of before and after using Prettier to format a block of JavaScript code:
Before:
function getFibonacciSequence(n) {
if (n === 1) {
return [0, 1];
} else {
const sequence = getFibonacciSequence(n - 1);
sequence.push(sequence[sequence.length - 1] + sequence[sequence.length - 2]);
return sequence;
}
}
console.log(getFibonacciSequence(10));
After:
function getFibonacciSequence(n) {
if (n === 1) {
return [0, 1];
} else {
const sequence = getFibonacciSequence(n - 1);
sequence.push(sequence[sequence.length - 1] + sequence[sequence.length - 2]);
return sequence;
}
}
console.log(getFibonacciSequence(10));
Before:
function quicksort(arr) {
if (arr.length <= 1) {
return arr;
}
const pivot = arr[0];
const left = [];
const right = [];
for (let i = 1; i < arr.length; i++) {
if (arr[i] < pivot) {
left.push(arr[i]);
} else {
right.push(arr[i]);
}
}
return quicksort(left).concat(pivot, quicksort(right));
}
const unsorted = [3, 2, 1, 4, 5];
console.log(quicksort(unsorted));
After:
function quicksort(arr) {
if (arr.length <= 1) {
return arr;
}
const pivot = arr[0];
const left = [];
const right = [];
for (let i = 1; i < arr.length; i++) {
if (arr[i] < pivot) {
left.push(arr[i]);
} else {
right.push(arr[i]);
}
}
return quicksort(left).concat(pivot, quicksort(right));
}
const unsorted = [3, 2, 1, 4, 5];
console.log(quicksort(unsorted));
As you can see from the example above, Prettier has applied consistent indentation, added semicolons, and added whitespace to make the code more readable and maintainable. Even in a larger block of code like this, Prettier can make a big difference in the clarity and consistency of the code.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
Using Prettier with VSCode in a real-world project
-
Using Prettier with VSCode in a real-world project can help you and your team maintain a consistent and readable codebase. Here are some tips on how developers can use Prettier effectively:
-
Configure it to match your team’s coding style: It’s important to customize Prettier settings to match your team’s coding style, as this will ensure that every member is on the same page regarding code formatting. You can use a
.prettierrc
file or configure Prettier in your VSCode settings. -
Use Prettier with a linter: While Prettier can help with formatting, it does not check for errors in your code. To catch errors and enforce coding standards, you should use Prettier with a linter like TSLint.
-
Use Prettier with version control: When you commit your code changes to version control (e.g.,
Git
), Prettier can help ensure your code is formatted consistently across all contributors. You can set up apre-commit hook
to automatically format your code with Prettier before committing it.
Best practices for using Prettier with VSCode to write JavaScript
Here are some best practices for using Prettier with VSCode to write JavaScript:
-
Use a
.prettierrc
file to customize Prettier settings: Prettier provides many configuration options that can be used to customize the formatting of your code. Using a.prettierrc
file to set these options can help ensure consistency across your project and team. -
Install the extension for VSCode: The Prettier extension for VSCode provides integration with the editor, making it easy to format your code using Prettier. It also provides real-time feedback on formatting errors and suggestions.
-
Use with a linter: Prettier does not check for errors in your code, so it is important to use it with a linter like TSLint. This will help catch errors and enforce coding standards.
-
Use with version control: You can reduce conflicts and improve collaboration among team members by formatting your code consistently with Prettier. Set up a pre-commit hook to automatically format your code with Prettier before committing to version control.
-
Be aware of edge cases: While it can handle most code formatting scenarios, there may be some edge cases where you need to adjust the formatting manually. Be aware of these cases and be prepared to make manual adjustments as needed.
-
Avoid formatting code manually: Manually formatting code can be time-consuming and error-prone. Use the
Format Document
command or set up Prettier to automatically format your code whenever you save your work, save time and improve consistency. -
Follow the community standards: The Prettier community has established standards and conventions for using the tool. Following these standards can help ensure your code is formatted consistently with other users.
-
By following these best practices, you can use Prettier with VSCode to write JavaScript consistently, efficiently, and error-free.
-
Be aware of edge cases: While it can handle most code formatting scenarios, there may be some edge cases where you need to adjust the formatting manually. Be aware of these cases and be prepared to make manual adjustments as needed.
Edge Cases for Using Prettier
In terms of edge cases, there are a few things to keep in mind when using Prettier:
Inline code: If you have a lot of inline code, such as in a long string or template literal, Prettier may not be able to format it exactly as you expect. In these cases, you may need to manually adjust the formatting to ensure it is still readable.
Custom configurations: While Prettier comes with many sensible defaults, there may be cases where you need to customize the configuration to fit your specific project or coding style. This can require some experimentation and trial and error to get the settings just right.
Comments: Prettier will format comments by default, but you can customize how they are formatted by adjusting the configuration settings. However, it’s important to be mindful of how comments affect the readability of your code, especially if you have long, multi-line comments.
Conclusion
Prettier is a powerful code formatting tool that can help improve the consistency, readability, and maintainability of your JavaScript codebase. By integrating with VSCode and following best practices, such as customizing settings with a .prettierrc
file, using Prettier with a linter and version control, and avoiding manual formatting, you can save time and reduce errors in your code.