Mastering Error Handling and Logging: Elevate Your Blazor Application's Reliability
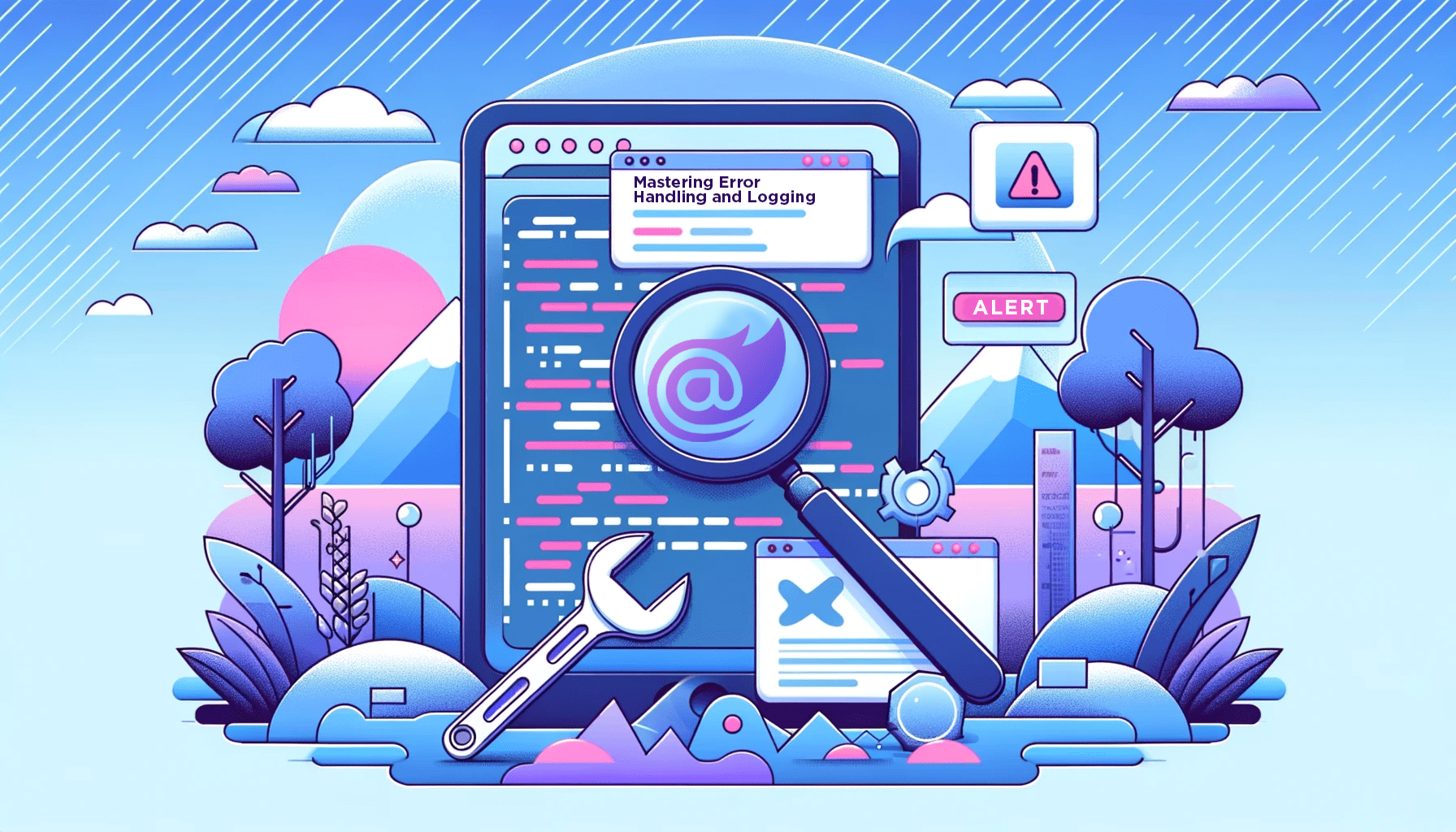
Managing errors and keeping detailed logs are crucial to building a user-friendly and reliable application. Efficient error handling leads to a smooth user experience and simplifies the process of identifying and rectifying bugs. This approach saves users significant time by streamlining the debugging process. This article will study why error management and logging are essential in Blazor applications.
Discover how at OpenReplay.com.
Applications are expected to be reliable and responsive. When an error occurs, it can disrupt the user’s workflow and may sometimes lead to frustration.
Effective handling ensures that errors are managed properly, preventing the application from crashing and offering clear and user-friendly error messages. Logging provides valuable insights into the application’s behavior and helps identify and fix issues more efficiently.
Blazor is a powerful framework for building web applications using C# and .NET. However, like any software application, Blazor applications can encounter errors during runtime. Runtime errors, or runtime exceptions, occur during the execution phase of an application.
These errors may range from unexpected exceptions to network-related issues; they can negatively impact user experience. Handling errors gracefully is vital to prevent the application from crashing and provide a seamless experience to the users.
Imagine being in complete darkness, trying to find a specific item without any light to guide you. In contrast, think about searching for the same item in well-lit conditions.
The likelihood of successfully locating the item is significantly higher when you have adequate light to assist you, instead of searching in total darkness. This analogy explains the significance of efficiently managing errors in an application.
Error Handling in Blazor
Blazor applications can encounter errors, including runtime exceptions, network failures, and data retrieval issues. It’s important to handle these errors gracefully to avoid application instability.
Blazor provides built-in mechanisms for error handling, such as try-catch blocks, to manage and recover from exceptions.
Handling Common Errors
Blazor applications can encounter common errors, such as Runtime Exceptions and Network-Related errors. These errors will be explained below;
- Runtime Exceptions: These errors occur when the application has been compiled successfully and an unexpected condition disrupts the flow of the application. A comprehensive explanation follows:
@page "/"
<p>Enter numerator (top-value):</p>
<input @bind="numerator" type="number" />
<p>Enter denominator (bottom-value):</p>
<input @bind="denominator" type="number" />
<button @onclick="HandleDivision">Divide @numerator by @denominator</button>
<p>@outputResult</p>
@code {
int numerator = 0;
int denominator = 0;
string outputResult = "";
void HandleDivision()
{
int result = numerator / denominator;
outputResult = $"Result: {result}";
}
}
Let’s break down the code step by step:
<input @bind="numerator" type="number" />
- The code snippet above creates an input field bound to a variable (in this case, it is the
numerator
). The@bind
directive ensures that the value entered by a user in the input field is automatically synchronized with the variable.
<button @onclick="HandleDivision">Divide @numerator by @denominator</button>
- Here is a button with an
@onclick
directive. When the button is clicked, it triggers theHandleDivision
method. The text inside the button dynamically displays the values ofnumerator
anddenominator
.
<p>@outputResult</p>
- This line creates a
paragraph (<p>)
element that will display the result of the division. The content of this paragraph is bound to theoutputResult
variable, which will be updated when the division is performed.
@code {
int numerator = 0;
int denominator = 0;
string outputResult = "";
void HandleDivision()
{
int result = numerator / denominator;
outputResult = $"Result: {result}";
}
}
- In the
@code
block, we have the C# code associated with the Blazor component. Three variables are declared:denominator
,numerator
, andoutputResult
. Thedenominator
andnumerator
are initialized to0
, andoutputResult
is an empty string("")
.
The HandleDivision
method is invoked when the button is clicked. It performs an integer division of numerator
by denominator
and updates the output result
string to display the result.
The code provided above compiles successfully without any errors. When you run this code with any number other than zero, it behaves as expected. Below there’s an image illustrating this scenario.
Do you want to guess what happens when you run the application with the number zero? See the image below;
What do you notice from the image above? The application failed! What exactly happened? How do we know what went wrong?
From the image above, the only detail provided is An unhandled exception has occurred
. We have this text because the exception was not gracefully handled. I will explain how to gracefully handle this type of exception when we are discussing Built-in Error Handling Mechanisms.
- Network-Related Errors: Connectivity problems can affect the application’s functionality. A comprehensive explanation follows;
@using System.Net.Http;
@page "/"
<h2>Replicating a Network-Related Issue.</h2>
<p><button @onclick="HandleApiRequest">Fetch data</button></p>
<p>@result</p>
@code {
string result = "";
async Task HandleApiRequest()
{
HttpClient client = new HttpClient();
result = await client.GetStringAsync("https://jsonplaceholder.typicode.com/todos/1");
}
}
Let’s take a deep dive into what the code snippet above does.
<p><button @onclick="HandleApiRequest">Fetch data</button></p>
- The line above, creates a
paragraph (<p>)
element containing a button. When the button is clicked, it triggers theHandleApiRequest
method.
<p>@result</p>
- This paragraph element displays the result of the API (Application Programming Interface) request. The content is bound to the
result
variable, which will be updated after processing the API request.
@code {
string result = "";
async Task HandleApiRequest()
{
HttpClient client = new HttpClient();
result = await client.GetStringAsync("https://jsonplaceholder.typicode.com/todos/1");
}
}
- In the
@code
block, aresult
string variable is declared to store the data retrieved from the API.
The HandleApiRequest
method is an Asynchronous method (async Task). Inside this method, an instance of HttpClient
is created to make HTTP
requests.
The GetStringAsync
method is then used to asynchronously fetch the content from the specified URL (Uniform Resource Locator) (in this case, “https://jsonplaceholder.typicode.com/todos/1”). The output is assigned to the result
variable.
The code you see above compiles smoothly with no errors. When you attempt to retrieve data with your network connection active, it does so without any issues. Take a look at the image below for reference.
The image shows that data retrieval is successful due to the functioning network connectivity. What do you think happens when there’s a connectivity issue? See the image below;
As seen in the image above, the application encountered a failure, but there’s no clear indication of the issue. I’ll delve into how to gracefully manage this type of exception when we cover Built-in Error Handling Mechanisms.
Significance of Graceful Error Handling
In the dynamic landscape of software, mastering effective error handling is very important. Here’s how it ensures your application stays robust and user-friendly:
- Effective error handling ensures that when an error occurs in an application, the application can rebound gracefully instead of crashing or presenting users with perplexing error messages.
- It’s crucial to display straightforward and user-friendly error messages while keeping detailed and well-written logs for easy debugging.
Built-in Error Handling Mechanisms
Blazor offers built-in error handling mechanisms, such as the try-catch
blocks, which allow developers to intercept exceptions and take appropriate actions. Let’s take a look at how to use try-catch blocks effectively.
try
{
// Code that might throw an exception
}
catch (Exception ex)
{
// Handle the exception, log it, and display a user-friendly error message
}
Here contains a list of exceptions you might find helpful.
With the try-catch
blocks, I will gracefully handle the Network and Runtime exceptions respectively.
@code {
async Task HandleApiRequest()
{
try
{
HttpClient client = new HttpClient();
result = await client.GetStringAsync("https://jsonplaceholder.typicode.com/todos/1");
}
catch (HttpRequestException ex)
{
result = "Unable to establish a connection. Please check your internet connectivity.";
}
}
}
Replace the HandleApiRequest()
method in Network-Related Errors, with the code above.
Can you guess what happens when we run the application above and there’s a connectivity issue?
From the image above, you can see the exception has been gracefully handled, and it’s very easy to detect what exactly went wrong while trying to fetch the data. This is one of the advantages of gracefully handling an exception.
@code {
void HandleDivision()
{
try
{
int result = numerator / denominator;
outputResult = $"Result: {result}";
}
catch (DivideByZeroException ex)
{
outputResult = $"Error: {ex.Message}";
}
}
}
Replace the HandleDivision()
method in Runtime Exceptions, with the code above.
Can you guess what happens when we run the application above and there’s a connectivity issue?
As shown in the image above, a clear error message has been displayed to the user, making debugging much easier.
Client-Side Logging with Browser Console
Client-side logging is a useful tool for developers because it lets you capture and examine how the application behaves directly from the user’s web browser. Blazor applications can make use of the browser’s console for logging activities.
Using the Browser’s Console
In Blazor applications, you can log messages (console.log
), warnings (console.warn
), and errors (console.error
) using the browser’s console object.
Using the console.log
directly in a Blazor application without involving JSRuntime
is impossible. Blazor is a .NET-based framework, and C# code runs on the server side, while the browser’s console.log
function operates on the client side through JavaScript.
To bridge the gap between C# and JavaScript, you must use JSRuntime
to invoke JavaScript functions like console.log
from your Blazor components.
JSRuntime
is the recommended way to interact with JavaScript from a Blazor application and provides a safe and controlled way to execute JavaScript functions in the browser.
Without JSRuntime
, you wouldn’t have direct access to client-side JavaScript functions from your Blazor components.
Here’s a guide on how to log various types of messages:
@page "/"
<h2>Console Error in Blazor</h2>
<button @onclick="LogMessage">Log Message</button>
@code {
[Inject]
private IJSRuntime JSRuntime { get; set; }
private async Task LogMessage()
{
await JSRuntime.InvokeVoidAsync("console.error", "This is a log message from Blazor.");
}
}
I will explain what the code above does.
<button @onclick="LogMessage">Log Message</button>
This line creates a button. When the button is clicked, it triggers the LogMessage
method.
[Inject]
private IJSRuntime JSRuntime { get; set; }
The function of the [Inject]
directive is to inject services, such as IJSRuntime
, into a Blazor component. In this case, we are injecting IJSRuntime
to enable interaction with client-side JavaScript from the Blazor component.
private async Task LogMessage()
{
await JSRuntime.InvokeVoidAsync("console.error", "This is a log message from Blazor.");
}
The LogMessage
method is Asynchronous (async Task). Inside the method above, it uses the injected JSRuntime
to invoke a JavaScript function. The InvokeVoidAsync
method calls a JavaScript function named console.error
with the provided log message string.
To view the log message from the above, do the following;
- Open your browser’s developer tools (usually by pressing
F12
or right-clicking and selecting “Inspect”), and go to the console tab. You should see the log message printed there. After clicking the ’ Log Message ’ button, the result should look like the image below.
Logging Techniques in Blazor
Blazor provides multiple logging techniques, allowing you to effectively capture and analyze application behavior. Two commonly used methods are using the Console
object and the ILogger
service.
Using the Console Object
The Console
object is a simple way to log messages directly to the browser’s console, as demonstrated in the previous section.
Using the ILogger Service
The ILogger
service represents a sophisticated and versatile logging mechanism that offers enhanced capabilities. It enables users to finely configure logging levels and capture bespoke information within log entries.
I will explain how to seamlessly integrate logging within a Blazor component and the practical applications of logging messages using the ILogger
interface.
@using Microsoft.Extensions.Logging
@page "/"
@inject ILogger<Index> Logger
<h1>Logger Example</h1>
<button @onclick="LogInfo">Log Info</button>
<button @onclick="LogError">Log Error</button>
<button @onclick="LogWarn">Log Warn</button>
NOTE: Make sure you have the right @using
properly imported.
- The
@page "/"
directive defines the route for the component; in this case, we are targetting the root component("/")
. - The
@inject
is like asking for something, andILogger<Index>
is specifying what you want – it’s saying you want a logger for theIndex
class which is also the component. And theLogger
is like the nickname or a handle you’re giving to this logger so you can use it easily in your code.
@code {
private void LogInfo()
{
Logger.LogInformation("Information message from Blazor component.");
}
private void LogError()
{
Logger.LogError("Error message from Blazor component.");
}
private void LogWarn()
{
Logger.LogWarning("Warning message from Blazor component");
}
}
- The
@code
block contains the C# code for the component which logs the error.
Three methods, LogInfo
, LogError
and LogWarn
, demonstrate logging scenarios from the Logger
above, with different log levels: information
, error
and warn
. Running the code above will produce the result below.
Configuring Logging Levels
You can configure logging levels to filter and control the volume of log messages. Common levels include:
Information
: General information about application behavior.Warning
: Warnings that may not prevent the application from functioning.Error
: Errors that can impact the application’s functionality.
Implementing Error Handling
Incorporating error handling in Blazor requires tactics to manage and showcase errors to users, simultaneously capturing comprehensive error details for effective debugging.
Presenting User-Friendly Error Messages
Providing clear and user-friendly error messages ensures users are not only informed about issues but also guided on the necessary steps to navigate through challenges. Here’s a breakdown of essential practices when dealing with errors:
- Provide user-friendly error messages when an error occurs.
- Clearly explain the issue to help users understand what went wrong.
- Offer guidance on how to proceed in response to the error.
Best Practices and Recommendations
To ensure effective error handling and logging in Blazor applications, consider the following best practices and recommendations:
Differentiating Between Expected and Unexpected Errors
Error differentiation is a crucial aspect of maintaining a robust system. By categorizing errors based on their anticipated or unforeseen nature.
- Distinguish between anticipated errors, which can be handled gracefully.
- Identify unforeseen errors that require immediate attention.
- Unexpected errors may indicate a critical system breakdown.
Securing Error Information
Ensuring application security involves strategic handling of error information. Here are key considerations to minimize security threats and safeguard sensitive data:
- To reduce potential security threats, refrain from disclosing sensitive error details to end-users.
- Guarantee that access to error information is restricted solely to authorized personnel.
Conclusion
In summary, incorporating error handling and logging into the development of Blazor applications is essential. Ensuring effective error management is key to maintaining a seamless user experience, averting system crashes, and delivering precise and informative error messages.
By adhering to best practices and adopting recommended approaches, you have the opportunity to craft web applications that excel in both resilience and user-friendliness while upholding security standards. Keep in mind that adept error handling and logging play a pivotal role in the overall success of your Blazor projects.
Understand every bug
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — the open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.