Handle User Interactions: Five Essential Event Bindings in Angular 18
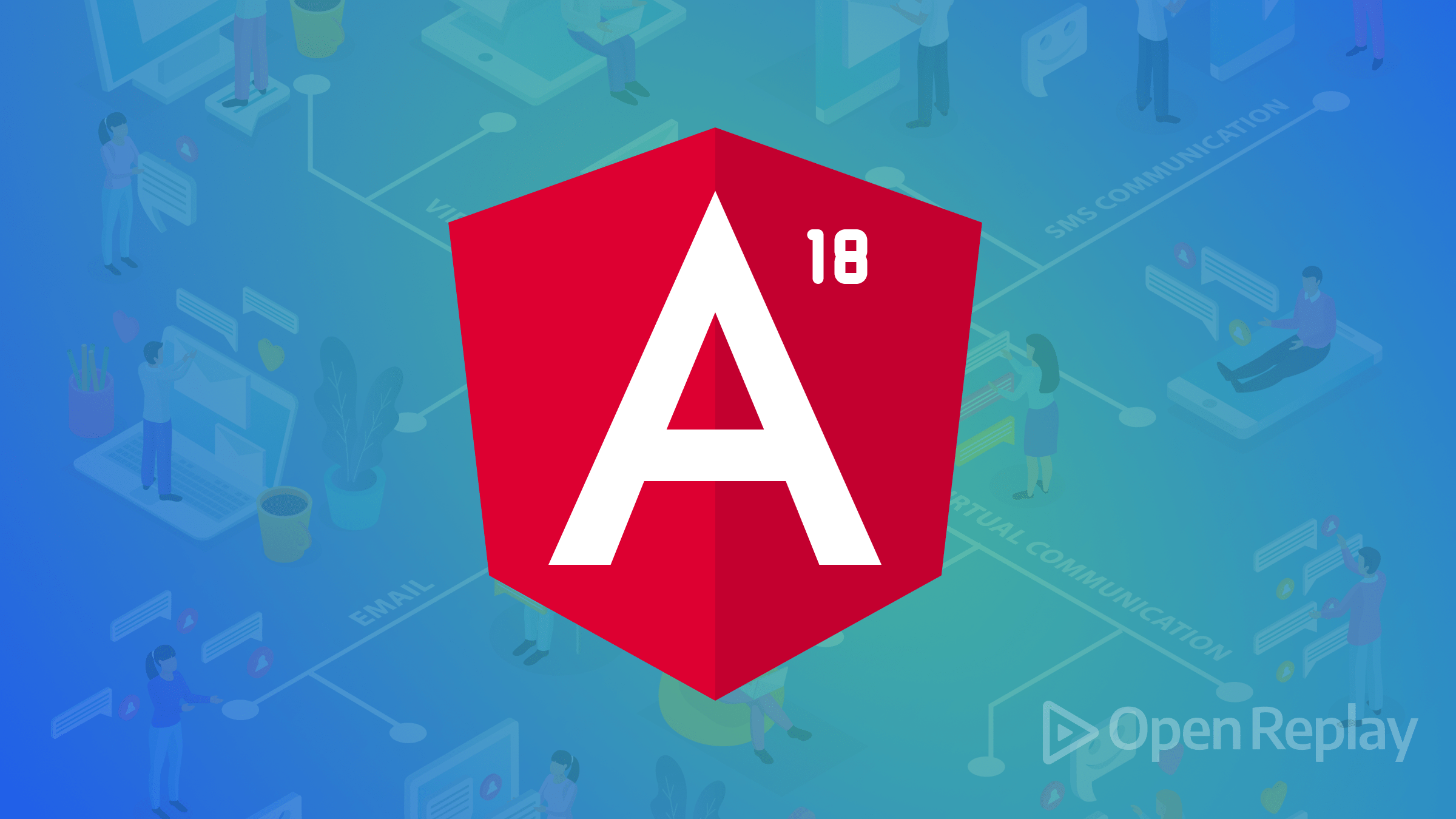
When users interact with forms, what happens behind these interactions are some event bindings that are implemented to listen and execute specific functions when a user submits a form, clicks an input field, types, or clicks outside of an input field. There are five key events you must handle in Angular 18, and this article will show you how.
Discover how at OpenReplay.com.
Let’s imagine a scenario where a car reverses because the gear is in reverse or where the vehicle abruptly stops due to the application of a brake. We can liken event bindings to the short examples given. What event bindings do is listen for a user’s interaction with various HTML elements and respond by doing something. So, now, a connection is created between the user interface and the logic by capturing events such as click
, input
, blur
, and other actions done by a user.
The use of forms makes it easy for users’ information to be captured in a database or processed for many purposes, including but not limited to event registration, surveys, or job applications. In this article, we’ll review five important event bindings in Angular used when users interact with forms.
Understanding Real-Time Data Capture with Angular’s Input Event Binding
We now have an understanding of what event binding is, and in this section, we’ll look at one of the various types of event listeners.
The different types of event bindings listen for actions performed by a user differently, and this type, which we’ll discuss in this section, listens and triggers a function immediately when a user types. For example, when a user types a value in an input field, the input
event listener catches the changes made to that value in real-time just as the user types. Its importance is not limited to live search, form updates, and data validation.
Let’s look at an example to know better.
In your event-binding.component.html
file, enter the code below:
<section class="border border-danger w-50 mt-4 p-4 form-section">
<div>
<h4 class="text-center">Contact Form</h4>
<form action="">
<div class="mt-3">
<label class="form-label">First Name</label>
<input
type="text"
placeholder="Enter your first name here"
class="form-control"
(input)="handleUserInput($event)"
aria-describedby="basic-addon3"
/>
<small class="text-success" *ngIf="nameFound">Name Found</small>
<small class="text-danger" *ngIf="firstName && !nameFound"
>Name Not Found</small
>
</div>
<div class="mt-4">
<label class="form-label">Last Name</label>
<input
type="text"
placeholder="Enter your last name here"
class="form-control"
(input)="handleUserInput($event)"
aria-describedby="basic-addon3"
/>
</div>
<div class="mt-4">
<button class="bg-primary border border-primary text-white w-50 p-1">
Submit
</button>
</div>
</form>
</div>
</section>
When we run the above code, we’ll see a basic form in our browser. This is the template we’ll use throughout this article.
In this part, we attached an input
event listener to the firstName
and lastName
fields. We also added a success text that shows up if a condition is satisfied.
Enter this code in your event-binding.component.ts
file:
import { Component } from "@angular/core";
@Component({
selector: "app-event-binding",
templateUrl: "./event-binding.component.html",
styleUrls: ["./event-binding.component.css"],
})
export class EventBindingComponent {
firstName: string = "";
lastName: string = "";
nameFound!: boolean;
public firstNames = ["Chioma", "Fola", "Ahmed", "Yunussa", "Ugo", "Amaka"];
ngOnInit(): void {}
handleUserInput(event: any) {
this.firstName = event.target.value;
console.log("First name: ", this.firstName);
this.nameFound = this.firstNames.some(
(firstName) => firstName.toLowerCase() === this.firstName,
);
console.log(this.nameFound);
if (this.nameFound) {
console.log("First name was found!!");
}
}
}
We defined an array of names so that when a user enters a value in the firstName
field, the input
event listener listens for changes to the value and then uses the value to check through the array if it matches with any of the names there. If we find a match, that is, if the value entered matches any of the names in the array, a success text is displayed; if not, we show a failure text.
Here is a demo showing what we’ve covered in this section.
Reacting to Element Focus Loss using Blur Event Binding
The blur
event listener listens for when the focus on an element is lost. Using filling out a form as an example, the blur
event listener pays attention to when your cursor is moved outside your input field. This event listener is used especially to provide feedback to a user when filling out a form. It is used to apply CSS styles to an element when it loses focus, fetch data, and save data automatically.
For example, we can use the blur
event listener to check for the maximum number of characters we want our password to have, and if it exceeds, we give a warning feedback to the user.
Let’s add this password
input field to our existing template.
<div class="mt-4">
<label class="form-label">Password</label>
<input
type="password"
(blur)="handlePasswordLength($event)"
placeholder="Enter your password here"
class="form-control"
aria-describedby="basic-addon3"
/>
<small *ngIf="passwordErrorMsg">{{ passwordErrorMsg }}</small>
</div>
In your existing component file, add the following code to your variable definition section:
passwordErrorMsg!: string;
maxPasswordLength: number = 8;
Here, we define the passwordErrorMsg
as a string that will display an error message if any, and we also define a variable maxPasswordLength
to hold the maximum password length as a number and set it to have a value of 8
.
Also, add the following function to your component file:
handlePasswordLength(event: any): void {
const userPassword = event.target.value;
this.passwordErrorMsg =
userPassword.length > this.maxPasswordLength
? `Your password is more than the maximum length of ${this.maxPasswordLength} characters`
: 'Approved password length';
}
After a user enters their password and clicks outside of the password input field, the blur
event listener listens for the password inputted by the user and then calls the handlePasswordLength
function, which in turn checks if the password length exceeds the value set in maxPasswordLength
. If it exceeds, it will throw a warning text that says the password is longer than the required length.
Here is a demo showing one of the numerous actions the blur
event listener plays.
Note that the warning message can also pop up as a dialog box.
Listening for Changes Effectively with the Change Event Binding
The change
event listener detects changes to an element value such as the text, radio button, checkbox, and file input elements. It updates content only after an element loses focus or a change has been made. It is important because it helps process data or values entered by a user immediately, and it also makes the user interface respond directly to user input changes.
In the following example, we’ll see how we can use the change
event listener to update a preview of Markdown content.
In your event-binding.component.html
file, enter the code below:
<form action="">
<div class="mt-2">
<h4>Markdown Editor</h4>
<textarea
(change)="updateMrkDwnPreview($event)"
placeholder="Enter markdown content here"
rows="5"
cols="40"
></textarea>
</div>
<div class="mt-3">
<h5>Preview Markdown Content</h5>
<div>
{{ markDownContent ? markDownContent : "You have no content yet" }}
</div>
</div>
</form>
Here we show a textarea
and a preview section of the content entered by a user in the textarea
.
In your event-binding.component.ts
file, enter the code below:
// add the code below to the variable definition section
markDownContent: string = '';
updateMrkDwnPreview(event: any) {
this.markDownContent = event.target.value;
console.log(this.markDownContent);
}
The markDownContent
variable holds the markdown content. The function updateMrkDwnPreview
updates the Markdown preview whenever a user enters a value in the textarea
and clicks outside the input field, as seen in the demo below.
Triggering Actions on User Clicks with Angular’s Click Event Binding
The click
event listener is interesting because it enables an action to take place with just a click. So, for example, while going through a website and you navigate to a page, that action is triggered by a click
event. You should note that any element is clickable.
A click
event listener is necessary as it is used for several actions such as interacting with web elements, navigation, and form submission, amongst many other actions.
Let’s take an example where we will see the click
event listener in action. So in this example, when we click on a like, comment, or retweet icon, it increases its count
.
In your event-binding.component.html
file, enter the code below:
<section class="border border-primary d-flex justify-content-between">
<div class="cursor-pointer">
<i
class="fas fa-heart fs-2 text-danger icon"
(click)="handleCount('like')"
></i>
<p class="text-center">{{ likeCount }}</p>
</div>
<div>
<i
class="far fa-comment fs-2 text-danger icon"
(click)="handleCount('comment')"
></i>
<p class="text-center">{{ commentCount }}</p>
</div>
<div>
<i
class="fas fa-retweet fs-2 text-danger icon"
(click)="handleCount(retweet)"
></i>
<p class="text-center">{{ retweetCount }}</p>
</div>
</section>
In your event-binding.component.ts
file, enter the code below:
// add these first three lines of code to the variable definition section
likeCount: number = 0;
commentCount: number = 0;
retweetCount: number = 0;
handleCount(action: string) {
switch (action) {
case 'like':
this.likeCount = this.likeCount + 1;
break;
case 'comment':
this.commentCount = this.commentCount + 1;
break;
case 'retweet':
this.retweetCount = this.retweetCount + 1;
break;
default:
break;
}
}
First, we define the icon count
variables and set it to a default value of 0
. Then we define the handleCount
function that is triggered anytime any of the icons are clicked. The function takes a string
parameter action
indicating the type of icon that was clicked (‘like’, ‘comment’, or ‘retweet’) before increasing the count
of the respective icon.
Here is a demo that shows what we’ve achieved in this section.
Capturing Form Submissions with Angular’s Submit Event Binding
The submit
event binder is used for handling form submission events. So, whenever a form is submitted, it triggers the submit
event binder, which in turn calls the function to send all form data to a server. The submit
event handler helps to validate a user’s input before submitting, and this includes preventing incorrect or incomplete data from being sent to a server.
Let’s see an example where the submit
event handler can be used.
Add the following code to the form
element in your existing event-binding.component.html
file:
(submit)="handleFormSubmit()"
Add the following code to your existing event-binding.component.ts
file:
handleFormSubmit() {
this.formData = {
firstName: this.firstName,
lastName: this.lastName,
password: this.password
}
console.log('Data submitted: ', this.formData)
}
The handleFormSubmit
function, when called, collects the values of firstName
, lastName
, and password
and logs them to your console. You can also have a code in the function to send the formData
to an endpoint.
Here is a demo.
Conclusion
We’ve gone through five essential event binders that can be used in Angular to either listen to click events, capture input changes, validate form submissions, react to element focus loss, or detect changes in form fields.
Understand every bug
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — the open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.